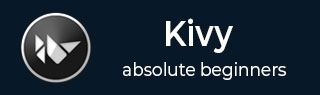
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Stencil View
The StencilView widget in Kivy library limits the canvas area of other children widgets added to it. Any instructions trying to draw outside the stencil view area will be clipped.
Stencil graphics instructions are used under the hood by the StencilView widget. It provides an efficient way to clip the drawing area of children.
The StencilView class is defined in the "kivy.uix.stencilview" module.
from kivy.uix.stencilview import StencilView
It may be noted that StencilView is not a layout. Hence, to add widgets to StencilView, you have to combine a StencilView and a Layout in order to achieve a layout's behavior. Further, you cannot add more than 128 stencil-aware widgets to the StencilView.
The general usage of StencilView is as follows −
st = StencilView(size=(x,y)) w = Widget() st.add_widget(w)
To understand how exactly StencilView limits the drawable area of a widget, let us first execute certain graphics drawing instructions, and then impose StencilView to see the difference.
Example
In the following code, a mywidget class extends the Widget class, and draws 200 circles at random positions and with random RGB values. (This doesn't use StencilView yet)
from kivy.app import App from kivy.uix.widget import Widget from kivy.graphics import * import random from kivy.core.window import Window Window.size = (720, 350) class mywidget(Widget): def __init__(self, *args): super().__init__(*args) for i in range(200): colorR = random.randint(0, 255) colorG = random.randint(0, 255) colorB = random.randint(0, 255) posx = random.randint(0, Window.width) posy = random.randint(0, Window.height) self.canvas.add(Color(rgb=(colorR / 255.0, colorG / 255.0, colorB / 255.0))) d = 30 self.canvas.add(Ellipse(pos=(posx, posy), size=(d, d))) class circlesapp(App): def build(self): w = mywidget() return w circlesapp().run()
Output
As you can see, the circles are drawn at random positions all over the app window area.
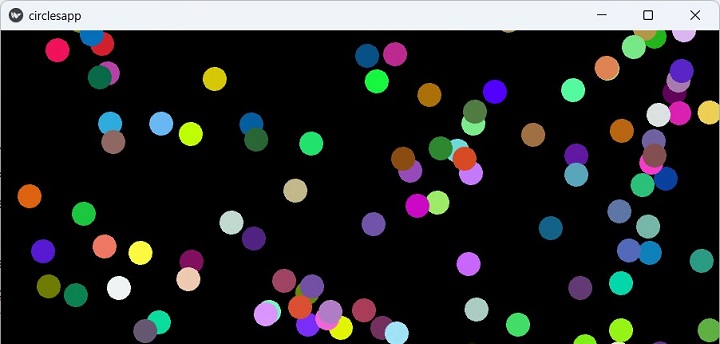
Now we chall apply the StencilView, and restrict the drawing area to 400×300 pixels in the center of the main window.
The StencilView object is created and we add a Widget object to it. The drawing loop remains the same.
class circlesapp(App): def build(self): st = StencilView( size_hint=(None, None), size=(400, 300), pos_hint={'center_x':.5, 'center_y':.5} ) w=widget() st.add_widget(w) return st
If we now run the app, we should see the random circles appearing inside the stencil area, for all the positions outside it the drawing instructions are restricted.
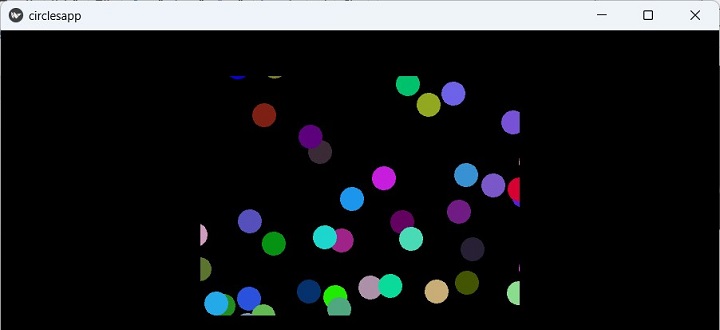
To Continue Learning Please Login