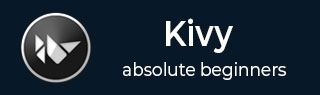
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Calculator App
In this chapter, we shall learn to build a calculator app using the Kivy library. A calculator consists of buttons for each digit and operators. It should have a button with "=" caption that computes the operation, and a button to clear the result.
Let us start with the following design in mind −

The above layout shows a input box at the top, followed by a 3-column layout for buttons, besides which the four operator buttons are arranged in one column.
We shall use a top grid layout with one column, and add the right-aligned TextInput below which we shall put another 2-column grid. The left cell of this grid houses digit, = and C buttons in three columns. The second column is another one-column grid for all the arithmetic operators.
The following "kv" file adapts this logic −
<calcy>: GridLayout: cols:1 TextInput: id:t1 halign:'right' size_hint:1,.2 font_size:60 GridLayout: cols:2 GridLayout: cols:3 size:root.width, root.height Button: id:one text:'1' on_press:root.onpress(*args) Button: id:two text:'2' on_press:root.onpress(*args) Button: id:thee text:'3' on_press:root.onpress(*args) Button: id:four text:'4' on_press:root.onpress(*args) Button: id:five text:'5' on_press:root.onpress(*args) Button: id:six text:'6' on_press:root.onpress(*args) Button: id:seven text:'7' on_press:root.onpress(*args) Button: id:eight text:'8' on_press:root.onpress(*args) Button: id:nine text:'9' on_press:root.onpress(*args) Button: id:zero text:'0' on_press:root.onpress(*args) Button: id:eq text:'=' on_press:root.onpress(*args) Button: id:clr text:'C' on_press:root.onpress(*args) GridLayout: cols:1 size_hint:(.25, root.height) Button: id:plus text:'+' on_press:root.onpress(*args) Button: id:minus text:'-' on_press:root.onpress(*args) Button: id:mult text:'*' on_press:root.onpress(*args) Button: id:divide text:'/' on_press:root.onpress(*args)
Note that each button is bound with onpress() method on its on_press event.
The onpress() method basically reads the button caption (its text property) and decides the course of action.
If the button has a digit as its caption, it is appended to the text property of the TextInput box.
self.ids.t1.text=self.ids.t1.text+instance.text
If the button represents any arithmetic operator (+, -, *, /) the number in the textbox at that time is stored in a variable for further operation and the textbox is cleared.
if instance.text in "+-*/": self.first=int(self.ids.t1.text) self.ids.t1.text='0' self.op=instance.text
If the button's text property is '=', the number in the text box at that time is the second operand. Appropriate arithmetic operation is done and the result is displayed in the text box.
if instance.text=='=': if self.first==0: return self.second=int(self.ids.t1.text) if self.op=='+': result=self.first+self.second if self.op=='-': result=self.first-self.second if self.op=='*': result=self.first*self.second if self.op=='/': result=self.first/self.second self.ids.t1.text=str(result) self.first=self.second=0
Finally, if the button has C as the caption, the text box is set to empty.
if instance.text=='C': self.ids.t1.text='' self.first=self.second=0
Example
The above "kv" file is loaded inside the build() method of the App class. Here is the complete code −
from kivy.app import App from kivy.uix.button import Button from kivy.uix.gridlayout import GridLayout from kivy.core.window import Window Window.size = (720,400) class calcy(GridLayout): def __init__(self, **kwargs): super(calcy, self).__init__(**kwargs) self.first=self.second=0 def onpress(self, instance): if instance.text=='C': self.ids.t1.text='' self.first=self.second=0 elif instance.text in "+-*/": self.first=int(self.ids.t1.text) self.ids.t1.text='0' self.op=instance.text elif instance.text=='=': if self.first==0: return self.second=int(self.ids.t1.text) if self.op=='+': result=self.first+self.second if self.op=='-': result=self.first-self.second if self.op=='*': result=self.first*self.second if self.op=='/': result=self.first/self.second self.ids.t1.text=str(result) self.first=self.second=0 else: self.ids.t1.text=self.ids.t1.text+instance.text class calculatorapp(App): def build(self): return calcy(cols=3) calculatorapp().run()
Output
Run the above program and perform all the basic arithmetic calculations with this app.
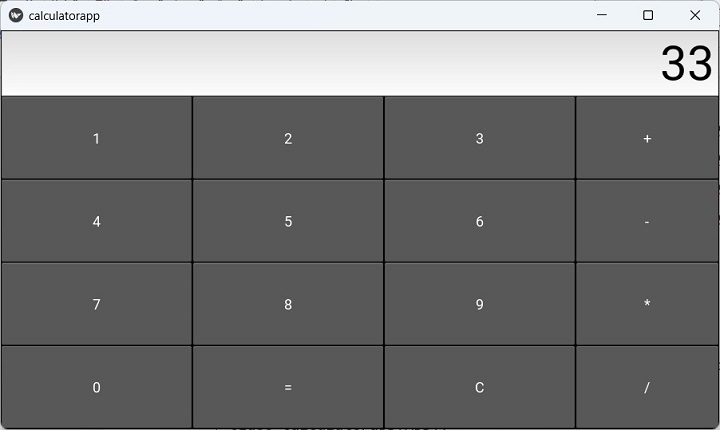
To Continue Learning Please Login