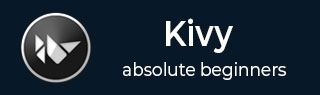
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Dropdown List
A drop-down widget in Kivy is quite different from similar widgets in other GUI toolkits. Kivy's dropdown shows not only labels, but any other widgets such as buttons, images, etc.
The DropDown class is defined in the "kivy.uix.dropdown" module.
from kivy.uix.dropdown import DropDown dropdown=DropDown()
Following steps are required to construct a dropdown object −
When adding other widgets in this object, we need to specify the height manually by disabling the size_hint, thereby the dropdown calculates the required area.
For each of the child widgets added in DropDown, you need to attach a callback that will call the select() method on the dropdown. Bind each child and add to the drop down object.
Add the dropdown to a main button and bind it with open() method of dropdown class
Finally, run the app and click the main button. You will see a list of child widgets dropping down. Click on any of them to invoke its associated callback.
Example
In this example, we shall demonstrate how Drop Down widget in Kivy works. We shall add ten buttons to the dropdown with a for loop as shown below −
dropdown = DropDown() for index in range(1, 11): btn = Button(text ='Button '+str(index), size_hint_y = None, height = 40) btn.bind(on_release = lambda btn: dropdown.select(btn.text)) dropdown.add_widget(btn) box.add_widget(dropdown)
We place a main button in the BoxLayout, add the dropdown object to it, and bind the main button with open() method of dropdown object.
box = BoxLayout(orientation='vertical') mainbutton = Button(text ='Drop Down Button', size_hint=(None, None), size =(250, 75), pos_hint ={'center_x':.5, 'top':1}) box.add_widget(mainbutton) mainbutton.add_widget(dropdown) mainbutton.bind(on_release = dropdown.open)
Finally, we need to listen for the selection in the dropdown list and assign the data to the button text.
dropdown.bind(on_select = lambda instance, x: setattr(mainbutton, 'text', x))
All these steps are included in the build() method of App class in the following code −
from kivy.app import App from kivy.uix.dropdown import DropDown from kivy.uix.button import Button from kivy.uix.boxlayout import BoxLayout from kivy.core.window import Window Window.size = (720, 400) class Drop_down_app(App): def build(self): box = BoxLayout(orientation='vertical') mainbutton = Button( text='Drop Down Button', size_hint=(None, None), size=(250, 75), pos_hint={'center_x': .5, 'top': 1} ) box.add_widget(mainbutton) dropdown = DropDown() for index in range(1, 11): btn = Button(text='Button ' + str(index), size_hint_y=None, height=40) btn.bind(on_release=lambda btn: dropdown.select(btn.text)) dropdown.add_widget(btn) box.add_widget(dropdown) mainbutton.add_widget(dropdown) mainbutton.bind(on_release=dropdown.open) dropdown.bind(on_select=lambda instance, x: setattr(mainbutton, 'text', x)) return box Drop_down_app().run()
Output
When we run the above code, the main button is visible.
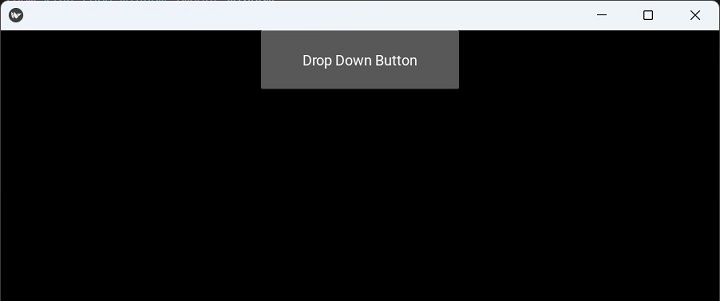
Click the button. As a result, the list of buttons drop down. The caption of the main button will change to that of the button from the list when clicked.
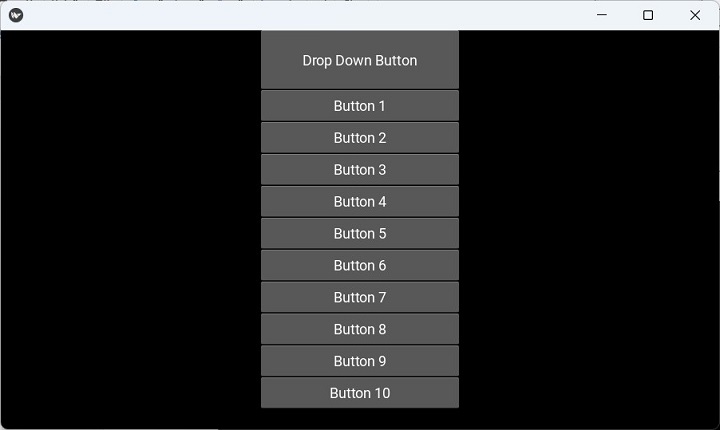
To Continue Learning Please Login