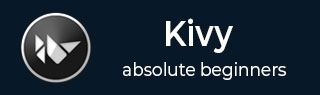
- Kivy Tutorial
- Kivy - Home
- Kivy Basics
- Kivy - Getting Started
- Kivy - Installation
- Kivy - Architecture
- Kivy - File Syntax
- Kivy - Applications
- Kivy - Hello World
- Kivy - App Life Cycle
- Kivy - Events
- Kivy - Properties
- Kivy - Inputs
- Kivy - Behaviors
- Kivy Buttons
- Kivy - Buttons
- Kivy - Button Events
- Kivy - Button Colors
- Kivy - Button Size
- Kivy - Button Position
- Kivy - Round Buttons
- Kivy - Disabled Buttons
- Kivy - Image Button
- Kivy Widgets
- Kivy - Widgets
- Kivy - Label
- Kivy - Text Input
- Kivy - Canvas
- Kivy - Line
- Kivy - Checkbox
- Kivy - Dropdown List
- Kivy - Windows
- Kivy - ScrollView
- Kivy - Carousel
- Kivy - Slider
- Kivy - Images
- Kivy - Popup
- Kivy - Switch
- Kivy - Spinner
- Kivy - Splitter
- Kivy - Progress Bar
- Kivy - Bubble
- Kivy - Tabbed Panel
- Kivy - Scatter
- Kivy - Accordion
- Kivy - File Chooser
- Kivy - Color Picker
- Kivy - Code Input
- Kivy - Modal View
- Kivy - Toggle Button
- Kivy - Camera
- Kivy - Tree View
- Kivy - reStructuredText
- Kivy - Action Bar
- Kivy - Video Player
- Kivy - Stencil View
- Kivy - VKeyboard
- Kivy - Touch Ripple
- Kivy - Audio
- Kivy - Videos
- Kivy - Spelling
- Kivy - Effects
- Kivy - Input Recorder
- Kivy - OpenGL
- Kivy - Text
- Kivy - Text Markup
- Kivy - Settings
- Kivy Layouts
- Kivy - Layouts
- Kivy - Float Layout
- Kivy - Grid Layouts
- Kivy - Box Layouts
- Kivy - Stack Layout
- Kivy - Anchor Layout
- Kivy - Relative Layout
- Kivy - Page Layout
- Kivy - Recycle Layout
- Kivy - Layouts in Layouts
- Kivy Advanced Concepts
- Kivy - Configuration Object
- Kivy - Atlas
- Kivy - Data Loader
- Kivy - Cache Manager
- Kivy - Console
- Kivy - Animation
- Kivy - Multistroke
- Kivy - Clock
- Kivy - SVGs
- Kivy - UrlRequest
- Kivy - Clipboard
- Kivy - Factory
- Kivy - Gesture
- Kivy - Language
- Kivy - Graphics
- Kivy - Drawing
- Kivy - Packaging
- Kivy - Garden
- Kivy - Storage
- Kivy - Vector
- Kivy - Utils
- Kivy - Inspector
- Kivy - Tools
- Kivy - Logger
- Kivy - Framebuffer
- Kivy Applications and Projects
- Kivy - Drawing App
- Kivy - Calculator App
- Kivy - Stopwatch App
- Kivy - Camera Handling
- Kivy - Image Viewer
- Kivy - Bezier
- Kivy - Canvas Stress
- Kivy - Circle Drawing
- Kivy - Widget Animation
- Kivy - Miscellaneous
- Kivy Useful Resources
- Kivy - Quick Guide
- Kivy - Useful Resources
- Kivy - Discussion
Kivy - Bezier
In this chapter, we shall create a Kivy app that will interactively draw a Bezier line along the list of points. If a closed line is drawn making use of the x and y coordinates computed with the help of following code segment, the resulting figure resembles a Pacman character −
from math import cos, sin, radians x = y = 300 z = 200 self.points = [x, y] for i in range(45, 360, 45): i = radians(i) self.points.extend([x + cos(i) * z, y + sin(i) * z]) with self.layout.canvas: Color(1.0, 0.0, 1.0) self.line = Line( points=self.points + self.points[:2], dash_offset=10, dash_length=100 )
It generates a line pattern as the following −
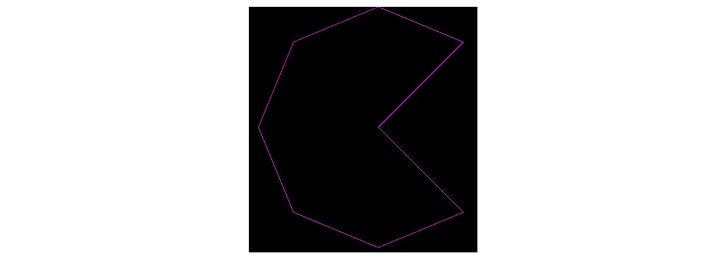
Then, we draw a Bezier line making use of the same list of points.
Color(1.0, 0.0, 1.0) self.line = Line( points=self.points + self.points[:2], dash_offset=0, dash_length=100 )
The Line as well as the Beizer will appear as this −
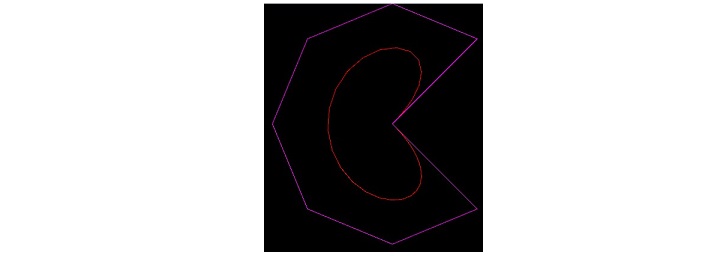
Now we want to construct these two curves dynamically. We use two slidersto change the dash length and offset values of both the line instruction and Bezier instruction as the value property of each slider changes with the following event handlers −
def _set_bezier_dash_offset(self, instance, value): # effect to reduce length while increase offset self.bezier.dash_length = 100 - value self.bezier.dash_offset = value def _set_line_dash_offset(self, instance, value): # effect to reduce length while increase offset self.line.dash_length = 100 - value self.line.dash_offset = value
As a result, change the slider values to see how the two curves are redrawn dynamically.
Example
The complete code is given below −
from kivy.app import App from kivy.uix.floatlayout import FloatLayout from kivy.uix.label import Label from kivy.uix.slider import Slider from kivy.graphics import Color, Bezier, Line from kivy.core.window import Window Window.size = (720,400) class Main(App): title='Bezier Example' def _set_bezier_dash_offset(self, instance, value): # effect to reduce length while increase offset self.bezier.dash_length = 100 - value self.bezier.dash_offset = value def _set_line_dash_offset(self, instance, value): # effect to reduce length while increase offset self.line.dash_length = 100 - value self.line.dash_offset = value def build(self): from math import cos, sin, radians x = y = 300 z = 200 # Pacman ! self.points = [x, y] for i in range(45, 360, 45): i = radians(i) self.points.extend([x + cos(i) * z, y + sin(i) * z]) print (self.points) self.layout = FloatLayout() with self.layout.canvas: Color(1.0, 0.0, 0.0) self.bezier = Bezier( points=self.points, segments=150, loop=True, dash_length=100, dash_offset=10 ) Color(1.0, 0.0, 1.0) self.line = Line( points=self.points + self.points[:2], dash_offset=10, dash_length=100) l1=Label( text='Beizer offset', pos_hint={'x':.1}, size_hint=(.1, None), height=50 ) self.layout.add_widget(l1) s1 = Slider( y=0, pos_hint={'x': .3}, size_hint=(.7, None), height=50 ) self.layout.add_widget(s1) s1.bind(value=self._set_bezier_dash_offset) l2=Label( text='Line offset', y=50, pos_hint={'x':.1}, size_hint=(.1, None), height=50 ) self.layout.add_widget(l2) s2 = Slider( y=50, pos_hint={'x': .3}, size_hint=(.7, None), height=50 ) self.layout.add_widget(s2) s2.bind(value=self._set_line_dash_offset) return self.layout if __name__ == '__main__': Main().run()
Output
The following screenshots show the curves at two different slider positions −
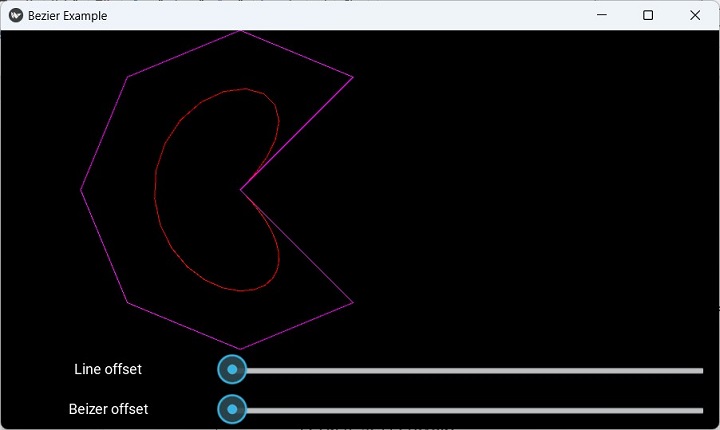
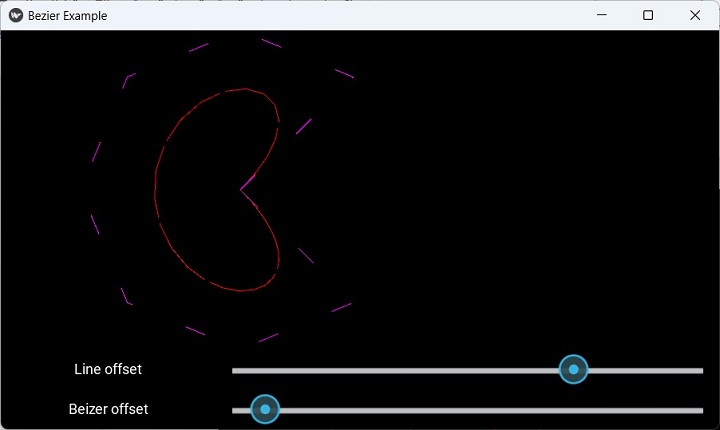
To Continue Learning Please Login