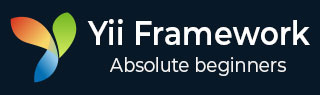
- Yii Tutorial
- Yii - Home
- Yii - Overview
- Yii - Installation
- Yii - Create Page
- Yii - Application Structure
- Yii - Entry Scripts
- Yii - Controllers
- Yii - Using Controllers
- Yii - Using Actions
- Yii - Models
- Yii - Widgets
- Yii - Modules
- Yii - Views
- Yii - Layouts
- Yii - Assets
- Yii - Asset Conversion
- Yii - Extensions
- Yii - Creating Extensions
- Yii - HTTP Requests
- Yii - Responses
- Yii - URL Formats
- Yii - URL Routing
- Yii - Rules of URL
- Yii - HTML Forms
- Yii - Validation
- Yii - Ad Hoc Validation
- Yii - AJAX Validation
- Yii - Sessions
- Yii - Using Flash Data
- Yii - Cookies
- Yii - Using Cookies
- Yii - Files Upload
- Yii - Formatting
- Yii - Pagination
- Yii - Sorting
- Yii - Properties
- Yii - Data Providers
- Yii - Data Widgets
- Yii - ListView Widget
- Yii - GridView Widget
- Yii - Events
- Yii - Creating Event
- Yii - Behaviors
- Yii - Creating a Behavior
- Yii - Configurations
- Yii - Dependency Injection
- Yii - Database Access
- Yii - Data Access Objects
- Yii - Query Builder
- Yii - Active Record
- Yii - Database Migration
- Yii - Theming
- Yii - RESTful APIs
- Yii - RESTful APIs in Action
- Yii - Fields
- Yii - Testing
- Yii - Caching
- Yii - Fragment Caching
- Yii - Aliases
- Yii - Logging
- Yii - Error Handling
- Yii - Authentication
- Yii - Authorization
- Yii - Localization
- Yii - Gii
- Gii – Creating a Model
- Gii – Generating Controller
- Gii – Generating Module
- Yii Useful Resources
- Yii - Quick Guide
- Yii - Useful Resources
- Yii - Discussion
Yii - Using Controllers
Controllers in web applications should extend from yii\web\Controller or its child classes. In console applications, they should extend from yii\console\Controller or its child classes.
Let us create an example controller in the controllers folder.
Step 1 − Inside the Controllers folder, create a file called ExampleController.php with the following code.
<?php namespace app\controllers; use yii\web\Controller; class ExampleController extends Controller { public function actionIndex() { $message = "index action of the ExampleController"; return $this->render("example",[ 'message' => $message ]); } } ?>
Step 2 − Create an example view in the views/example folder. Inside that folder, create a file called example.php with the following code.
<?php echo $message; ?>
Each application has a default controller. For web applications, the site is the controller, while for console applications it is help. Therefore, when the http://localhost:8080/index.php URL is opened, the site controller will handle the request. You can change the default controller in the application configuration.
Consider the given code −
'defaultRoute' => 'main'
Step 3 − Add the above code to the following config/web.php.
<?php $params = require(__DIR__ . '/params.php'); $config = [ 'id' => 'basic', 'basePath' => dirname(__DIR__), 'bootstrap' => ['log'], 'components' => [ 'request' => [ // !!! insert a secret key in the following (if it is empty) - this is //required by cookie validation 'cookieValidationKey' => 'ymoaYrebZHa8gURuolioHGlK8fLXCKjO', ], 'cache' => [ 'class' => 'yii\caching\FileCache', ], 'user' => [ 'identityClass' => 'app\models\User', 'enableAutoLogin' => true, ], 'errorHandler' => [ 'errorAction' => 'site/error', ], 'mailer' => [ 'class' => 'yii\swiftmailer\Mailer', // send all mails to a file by default. You have to set // 'useFileTransport' to false and configure a transport // for the mailer to send real emails. 'useFileTransport' => true, ], 'log' => [ 'traceLevel' => YII_DEBUG ? 3 : 0, 'targets' => [ [ 'class' => 'yii\log\FileTarget', 'levels' => ['error', 'warning'], ], ], ], 'db' => require(__DIR__ . '/db.php'), ], //changing the default controller 'defaultRoute' => 'example', 'params' => $params, ]; if (YII_ENV_DEV) { // configuration adjustments for 'dev' environment $config['bootstrap'][] = 'debug'; $config['modules']['debug'] = [ 'class' => 'yii\debug\Module', ]; $config['bootstrap'][] = 'gii'; $config['modules']['gii'] = [ 'class' => 'yii\gii\Module', ]; } return $config; ?>
Step 4 − Type http://localhost:8080/index.php in the address bar of the web browser, you will see that the default controller is the example controller.
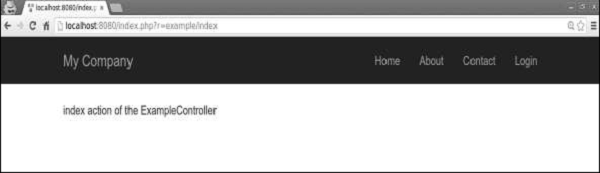
Note − The Controller IDs should contain English letters in lower case, digits, forward slashes, hyphens, and underscores.
To convert the controller ID to the controller class name, you should do the following −
- Take the first letter from all words separated by hyphens and turn it into uppercase.
- Remove hyphens.
- Replace forward slashes with backward ones.
- Add the Controller suffix.
- Prepend the controller namespace.
Examples
page becomes app\controllers\PageController.
post-article becomes app\controllers\PostArticleController.
user/post-article becomes app\controllers\user\PostArticleController.
userBlogs/post-article becomes app\controllers\userBlogs\PostArticleController.