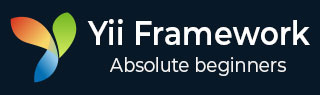
- Yii Tutorial
- Yii - Home
- Yii - Overview
- Yii - Installation
- Yii - Create Page
- Yii - Application Structure
- Yii - Entry Scripts
- Yii - Controllers
- Yii - Using Controllers
- Yii - Using Actions
- Yii - Models
- Yii - Widgets
- Yii - Modules
- Yii - Views
- Yii - Layouts
- Yii - Assets
- Yii - Asset Conversion
- Yii - Extensions
- Yii - Creating Extensions
- Yii - HTTP Requests
- Yii - Responses
- Yii - URL Formats
- Yii - URL Routing
- Yii - Rules of URL
- Yii - HTML Forms
- Yii - Validation
- Yii - Ad Hoc Validation
- Yii - AJAX Validation
- Yii - Sessions
- Yii - Using Flash Data
- Yii - Cookies
- Yii - Using Cookies
- Yii - Files Upload
- Yii - Formatting
- Yii - Pagination
- Yii - Sorting
- Yii - Properties
- Yii - Data Providers
- Yii - Data Widgets
- Yii - ListView Widget
- Yii - GridView Widget
- Yii - Events
- Yii - Creating Event
- Yii - Behaviors
- Yii - Creating a Behavior
- Yii - Configurations
- Yii - Dependency Injection
- Yii - Database Access
- Yii - Data Access Objects
- Yii - Query Builder
- Yii - Active Record
- Yii - Database Migration
- Yii - Theming
- Yii - RESTful APIs
- Yii - RESTful APIs in Action
- Yii - Fields
- Yii - Testing
- Yii - Caching
- Yii - Fragment Caching
- Yii - Aliases
- Yii - Logging
- Yii - Error Handling
- Yii - Authentication
- Yii - Authorization
- Yii - Localization
- Yii - Gii
- Gii – Creating a Model
- Gii – Generating Controller
- Gii – Generating Module
- Yii Useful Resources
- Yii - Quick Guide
- Yii - Useful Resources
- Yii - Discussion
Yii - Sessions
Sessions make data accessible across various pages. A session creates a file on the server in a temporary directory where all session variables are stored. This data is available to all the pages of your web site during the visit of that particular user.
When a session starts, the following happens −
PHP creates a unique ID for that particular session.
A cookie called PHPSESSID is sent on the client side (to the browser).
The server creates a file in the temporary folder where all session variables are saved.
When a server wants to retrieve the value from a session variable, PHP automatically gets the unique session ID from the PHPSESSID cookie. Then, it looks in its temporary directory for the needed file.
To start a session, you should call the session_start() function. All session variables are stored in the $_SESSION global variable. You can also use the isset() function to check whether the session variable is set −
<?php session_start(); if( isset( $_SESSION['number'] ) ) { $_SESSION['number'] += 1; }else { $_SESSION['number'] = 1; } $msg = "This page was visited ". $_SESSION['number']; $msg .= "in this session."; echo $msg; ?>
To destroy a session, you should call the session_destroy() function. To destroy a single session variable, call the unset() function −
<?php unset($_SESSION['number']); session_destroy(); ?>
Using Sessions in Yii
Sessions allow data to be persisted across user requests. In PHP, you may access them through the $_SESSION variable. In Yii, you can get access to sessions via the session application component.
Step 1 − Add the actionOpenAndCloseSession method to the SiteController.
public function actionOpenAndCloseSession() { $session = Yii::$app->session; // open a session $session->open(); // check if a session is already opened if ($session->isActive) echo "session is active"; // close a session $session->close(); // destroys all data registered to a session $session->destroy(); }
In the above code, we get the session application component, open a session, check whether it is active, close the session, and finally destroy it.
Step 2 − Type http://localhost:8080/index.php?r=site/open-and-close-session in the address bar of the web browser, you will see the following.
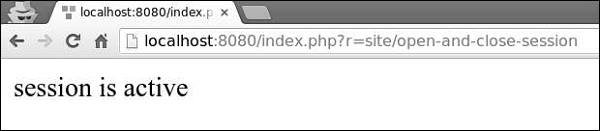
To access session variables, you may use set() and get() methods.
Step 3 − Add an actionAccessSession method to the SiteController.
public function actionAccessSession() { $session = Yii::$app->session; // set a session variable $session->set('language', 'ru-RU'); // get a session variable $language = $session->get('language'); var_dump($language); // remove a session variable $session->remove('language'); // check if a session variable exists if (!$session->has('language')) echo "language is not set"; $session['captcha'] = [ 'value' => 'aSBS23', 'lifetime' => 7200, ]; var_dump($session['captcha']); }
Step 4 − Go to http://localhost:8080/index.php?r=site/access-session, you will see the following.
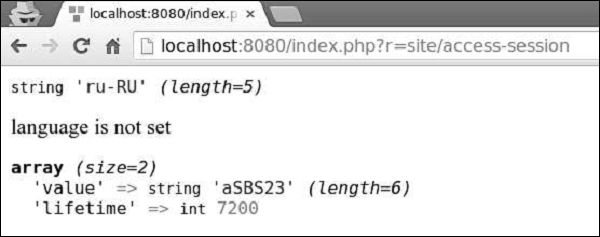