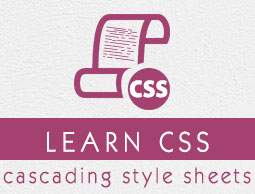
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Pseudo-class :hover Property
The :hover pseudo-class in CSS is used to target an element when the user hovers over it with the mouse cursor. Its purpose is to apply styles or trigger specific behaviors to enhance the user experience or provide additional visual feedback.
:hover helps make interactive elements more dynamic and engaging without requiring any user input beyond moving the mouse.
Syntax
:hover { /* ... */ }
CSS :hover Example
Here is an example of changing the background color of a link on hover:
<html> <head> <style> div { padding: 1rem; } a { background-color: rgb(238, 135, 9); font-size: 1rem; padding: 5px; } a:hover { background-color: rgb(235, 235, 169); } </style> </head> <body> <div> <h3>Hover example - link</h3> <a href="#">Hover over to see the color change!!!</a> </div> </body> </html>
Here is an example of changing the background color of a button on hover:
<html> <head> <style> div { padding: 1rem; } button { background-color: greenyellow; font-size: large; } button:hover { background-color: gold; } </style> </head> <body> <h3>Hover example - button</h3> <button>Hover me!!!</button> </div> </body> </html>
Here is an example, where border of the link is changing on hover:
<html> <head> <style> .link { color: blue; text-transform: uppercase; padding: 20px; border: 4px solid blue; border-radius: 10px; display: inline-block; } .link:hover { color: #494949; border-radius: 45px; border-color: #494949; } </style> </head> <body> <h3>Hover effect on border of the link</h3> <div class="button"><a class="link" href="#">Check my borders on hover</a></div> </body> </html>
Here is an example, where box-shadow is added to the link on hover:
<html> <head> <style> a { font-size: 2rem; color: #071402; margin: 0 .25rem; padding: 0 .25rem; transition: color .3s ease-in-out, box-shadow .3s ease-in-out; } a:hover { box-shadow: inset 100px 0 0 100px #9ce2cc; color: rgb(240, 32, 32); } </style> </head> <body> <div> <a href="#">Hover over me!!</a> </div> </body> </html>
Here is an example, where the background grows over a link on hover:
<html> <head> <style> a { text-decoration-line: underline; color: #18272F; font-size: 2rem; position: relative; } a::before { content: ''; background-color: rgba(118, 213, 83, 0.75); font-size: 2rem; position: absolute; left: 0; bottom: 3px; width: 100%; height: 10px; z-index: -1; transition: all .5s ease-in-out; } a:hover::before { bottom: 0; height: 100%; } </style> </head> <body> <div> <a href="#">Hover over me!!</a> </div> </body> </html>
Here is an example, where the underline of the link gives a rainbow color effect on hover:
<html> <head> <style> a { color: black; text-decoration: none; } a { background: linear-gradient( to right, rgb(179, 232, 168), rgb(185, 162, 215) ), linear-gradient( to right, rgba(255, 0, 0, 1), rgba(255, 0, 180, 1), rgba(0, 200, 50, 1) ); background-size: 100% 5px, 0 5px; background-position: 100% 100%, 0 100%; background-repeat: no-repeat; transition: background-size 700ms; } a:hover { background-size: 0 5px, 100% 5px; } body { display: grid; font-family: Verdana, Geneva, Tahoma, sans-serif; font-size: 2rem; height: 100vh; } </style> </head> <body> <div> <a href="#">Hover over me!!</a> </div> </body> </html>
Here is an example, where the a shadow effect is given to a button on hover:
<html> <head> <style> body { display: grid; justify-content: center; align-items: center; } .glow { padding: 10px; width: 250px; height: 50px; border: none; outline: none; color: #fff; background: #111; cursor: pointer; position: relative; z-index: 0; border-radius: 20px; } .glow:before { content: ''; background: linear-gradient(60deg, #ff0000, #ff7300, #fffb00, #48ff00, #00ffd5, #002bff, #7a00ff, #ff00c8, #ff0000); position: absolute; top: -4px; left:-4px; background-size: 400%; z-index: -1; filter: blur(15px); width: calc(100% + 6px); height: calc(100% + 6px); animation: glowing 20s linear infinite; opacity: 0; transition: opacity .3s ease-in-out; border-radius: 10px; } .glow:active { color: rgb(246, 235, 235) } .glow:active:after { background: transparent; } .glow:hover:before { opacity: 1; } .glow:after { z-index: -1; content: ''; position: absolute; width: 100%; height: 100%; background: #111; left: 0; top: 0; border-radius: 10px; } @keyframes glowing { 0% { background-position: 0 0; } 50% { background-position: 400% 0; } 100% { background-position: 0 0; } } </style> </head> <body> <button class="glow" type="button">HOVER OVER & CLICK!</button> </body> </html>
To Continue Learning Please Login