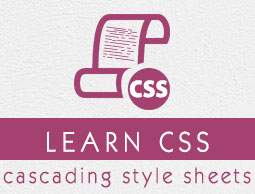
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS Function - round()
The CSS round() function returns a rounded number determined by a specific rounding method.
Authors can use a custom CSS property (such as --my-property) to specify the rounding value, interval, or both. If these values are already known, using the round() function becomes redundant.
Possible values
The round(<rounding-strategy>, valueToRound, roundingInterval) function takes three parameters. The first is the value you want to round, and the second is the precision number.
The valueToRound is then rounded to the nearest integer multiple of roundingInterval based on the specified ,rounding-strategy.
<rounding-strategy> - The rounding strategy can have one of the following values:
up - Round the valueToRound up to the nearest integer multiple of roundingInterval. If the value is negative, it will be adjusted to a more positive value. This operation is equivalent to using the JavaScript method Math.ceil().
down(default) - Round the valueToRound down to the nearest integer multiple of roundingInterval. If the value is negative, it is adjusted to a more negative value. This operation is equivalent to using the JavaScript method Math.floor().
nearest - Round the valueToRound to the nearest integer multiple of roundingInterval, which may be either above or below the value. If valueToRound is exactly halfway between the rounding targets above and below (neither of which is the nearest), rounding up occurs. This is similar to using the JavaScript method Math.round().
to-zero - Round valueToRound to the nearest integer multiple of roundingInterval that is closer to zero. This operation is similar to using the JavaScript Math.trunc() method.
valueToRound - The value to be rounded must be a <number>, <dimension>, or <percentage> or a mathematical expression that resolves to one of these types.
roundingInterval - The rounding interval may be a <number>, <dimension>, or <percentage> or a mathematical expression that resolves to one of these values.
Return Value
The value of valueToRound is rounded to the nearest lower or higher integer multiple of roundingInterval, based on the chosen rounding strategy.
If roundingInterval is 0, the outcome will be NaN(Not a Number).
When both valueToRound and roundingInterval are infinite, the result is NaN (Not a Number).
If valueToRound is infinite but roundingInterval is finite, the result remains as the same infinity value.
If valueToRound is finite but roundingInterval is infinite, the result is determined by the rounding strategy and the sign of valueToRound:
up - If valueToRound is positive (not zero), the result is +∞. If valueToRound is 0⁺, the result is 0⁺. Otherwise, the result is 0⁻.
down - If valueToRound is negative (not zero), the result is −∞. If valueToRound is 0⁻, the result is 0⁻. Otherwise, the result is 0⁺.
nearest, to-zero - If valueToRound is positive or 0⁺, the result is 0⁺. Otherwise, the result is 0⁻.
The argument calculations can result in <number>, <dimension>, or <percentage>, but they must have the same type.
If not, the function is invalid; the resulting value will have the same type as the arguments.
If valueToRound is an integer multiple of roundingInterval, round() resolves to valueToRound.
Otherwise, if valueToRound falls between two multiples of roundingInterval, it considers the lower multiple closer to −∞ and the upper multiple closer to +∞.
Syntax
round( <rounding-strategy>? , <calc-sum> , <calc-sum> )
The round() property is supported only by Firefox browser
CSS round() - Basic Example
The following example demonstrates how the round() function can modify the dimensions of elements based on different rounding strategies.
<html> <head> <style> body { justify-content: center; align-items: center; height: 100vh; margin: 0; } .box { width: 200px; height: 100px; margin: 20px; display: flex; justify-content: center; align-items: center; font-size: 20px; border: 2px solid black; background-color: lightblue; --rounding-interval: 20px; } .exact { width: calc(100px + 40px); } .up { width: round(up, 102px,var(--rounding-interval)); } .down { width: round(down, 119px, var(--rounding-interval)); } .to-zero { width: round(to-zero, 115px, var(--rounding-interval)); } .nearest { width: round(121px, 25px) } </style> </head> <body>Check the proper working on Firefox Browser
<div class="box exact">Exact Value 140px</div> <div class="box up">102px Rounded Up to 120px</div> <div class="box down">119px Rounded Down to 100px</div> <div class="box to-zero">115px Rounded to-zero to 100px</div> <div class="box nearest">121px Rounded to-nearest to 125px</div> </body> </html>
To Continue Learning Please Login