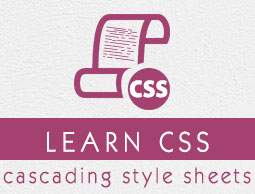
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS Function - calc()
The CSS function calc() allows values to be calculated when defining CSS properties. It is applicable to the values <length>, <frequency>, <angle>, <time>, <percentage>, <number> or <integer>.
The function calc() accepts a single expression as parameter and the result of this expression is used as value.
The expression may consist of different operators, and follow the standard rule for operator precedence.
+ Addition.
- Subtraction
* Multiplication. At least one of the arguments must be a <number>.
/ Division. The right-hand side must be a <number>.
Points to remember:
The + and - operators within calc() must be separated by spaces to avoid confusion.
The * and / operators in calc() do not require a space, although it is advisable to include it for consistency.
Mathematical expressions with percentages for widths and heights on the table columns, column groups, rows, row groups, and cells in both auto and fixed layout tables may be treated as if auto has been specified.
Nesting of calc() functions is allowed, treating the inner functions as simple parentheses.
The calc() function cannot directly replace numeric values with percentage values.
In the case of lengths, you cannot use 0 to represent 0px or any other length unit. It is necessary to specify the unit version.
Syntax
calc( <calc-sum> )
Usage with Integers
When calc() is used in a context where a <integer> is expected, the value is rounded to the nearest integer. For example
.modal { z-index: calc(6 / 4); }
This will give .modal a final z-index value of 2.
CSS calc() - Positioning An Object
The following example demonstrates the usage of calc(). calc() is used to calculate the box's height and width, resulting in a responsive layout with a constant 50px margin all around the box.
<html> <head> <style> .container { width: 100%; height: 100vh; display: flex; justify-content: center; align-items: center; } .box { width: calc(100% - 99px); height: calc(100% - 99px); margin: 50px; background-color: gray; text-align: center; } </style> </head> <body> <div class="container"> <div class="box"> <h2>This is an example of calc()</h2> </div> </div> </body> </html>
CSS calc() - Automatic Sizing Of Form Fields.
Calc() can also be used to ensure that form fields fit into the available space without extending beyond the edge of their container, while maintaining a reasonable margin.
The following examples shows sizing of form fields using calc()
<html> <head> <style> form { display: flex; flex-direction: column; align-items: center; background-color: lightblue; } input[type="text"], textarea { width: calc(100% - 50px); padding: 10px; margin: 10px; border-radius: 5px; border: 1px solid #ccc; resize: none; } input[type="submit"] { padding: 10px 20px; margin: 10px; border-radius: 5px; border: none; background-color: #4CAF50; color: #fff; font-size: 16px; cursor: pointer; } </style> </head> <body> <form> <label for="name">Name:</label> <input type="text" id="name" name="name" placeholder="Enter your name"> <label for="email">Email:</label> <input type="text" id="email" name="email" placeholder="Enter your email"> <label for="message">Message:</label> <textarea id="message" name="message" placeholder="Enter your message"></textarea> <input type="submit" value="Submit"> </form> </body> </html>
CSS calc() - Nesting of calc() With CSS Variables
The calc() can be utilized in conjunction with CSS variables as well. The following examples shows calc() with CSS variables
<html> <head> <style> :root { --width: 200px; --padding: 20px; } .container { width: calc(var(--width) + var(--padding)); background-color: lightgray; padding: var(--padding); } .box { width: calc(var(--width) / 2); height: calc(var(--width) / 2); background-color: black; margin: 0 auto; } </style> </head> <body> <div class="container"> <div class="box"></div> </div> </body> </html>
To Continue Learning Please Login