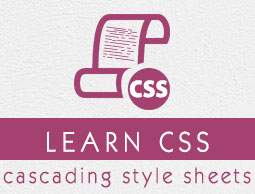
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS Data Type - <calc-constant>
CSS <calc-constant> data type defines predefined mathematical constants that can be used in CSS calculations such as e and pi. For convenience, some of these mathematical constants are provided directly by CSS, helping the authors avoid typing out many digits or compute them manually.
Possible Values
e − The mathematical constant known as the natural logarithm, which is approximately equal to 2.7182818284590452354
pi − It represents the ratio of a circle's circumference to its diameter. It is approximately equal to 3.1415926535897932
infinity & -infinity − Infinite value. It shows the biggest or smallest possible value.
NaN − A value that stands for "Not a Number" canonical casing.
Syntax
<calc()> = e | pi | infinity | -infinity | NaN;
Notes
When serializing arguments within calc(), it follows the IEEE-754 standard for floating-point math, considering cases involving constants such as infinity and NaN.
An infinity value will be returned when divided by zero. Depending on the value of numerator, it will be either positive or negative infinity.
If you add, subtract, or multiply infinity with anything, the result will be infinity unless you get NaN.
Any operation that includes at least one NaN argument, such as 0/0, infinity/infinity, 0*infinity, infinity + (-infinity), and infinity - infinity, will result in NaN.
Possible values include positive and negative zero (0⁺ and 0⁻). This causes the following effects:
Multiplication or division resulting in 0 with a single negative argument (e.g., -5 * 0 or 1 / (-infinity)) or negative results from other math functions will result in 0⁻.
0⁻ + 0⁻ or 0⁻ - 0 will result in 0⁻. Any additions or subtractions that result in a zero result in 0⁺.
Multiplying or dividing 0⁻ with a positive number (including 0⁺) results in a negative result (either 0⁻ or -infinity), but multiplying or dividing 0⁻ with a negative number results in a positive result.
Except for NaN, all constants are case-insensitive, therefore calc(Pi), calc(E), and calc(InFiNiTy) are valid.
e -e E pi -pi Pi infinity -infinity InFiNiTy NaN
Following are all invalid constant:
nan Nan NAN
CSS <calc-constant> - Use e and pi in calc()
The following example demonstrates the use of e in calc() for exponential rotation and use of pi in sin() function −
<html> <head> <style> .container { display: flex; flex-direction: row; justify-content: space-evenly; } .box { display: flex; flex-direction: column; align-items: left; width: 200px; } .box > div { width: 100px; height: 100px; margin: 10px; } span { font-family: monospace; font-size: 0.8em; } .e-box { background-color: red; } .pi-box { background-color: red; } </style> </head> <body> <div class="container"> <div class="box"> <label for="e-slider">e:</label> <input type="range" min="0" max="5" step="0.01" value="0" class="e-slider" /> <span class="e-value"></span> <div class="e-box"></div> </div> <div class="box"> <label for="pi-slider">pi:</label> <input type="range" min="0" max="1" step="0.01" value="0" class="pi-slider" /> <span class="pi-value"></span> <div class="pi-box"></div> </div> </div> <script> const eSliderElements = document.querySelectorAll(".e-slider"); const piSliderElements = document.querySelectorAll(".pi-slider"); const eValueElements = document.querySelectorAll(".e-value"); const piValueElements = document.querySelectorAll(".pi-value"); eSliderElements.forEach(function (eSlider, index) { eSlider.addEventListener("input", function () { document.querySelectorAll(".e-box")[index].style.transform = "rotate(calc(1deg * pow(" + this.value + ", e)))"; eValueElements[index].textContent = document.querySelectorAll(".e-box")[index].style.transform; }); }); piSliderElements.forEach(function (piSlider, index) { piSlider.addEventListener("input", function () { document.querySelectorAll(".pi-box")[index].style.rotate = "calc(sin(" + this.value + " * pi) * 100deg)"; piValueElements[index].textContent = document.querySelectorAll(".pi-box")[index].style.rotate; }); }); </script> </body> </html>
CSS <calc-constant> - Infinity, NaN, and division by zero
The following example demonstrates the calculated width value when dividing by zero and how different calc() constants appear when serialized in the console −
<html> <head> <style> div { height: 100px; background-color: blue; width: calc(1px / 0); } </style> </head> <body> <div></div> <script> const divElement = document.querySelector("div"); document.write(divElement.offsetWidth); // infinity clamped to largest value for width document.write("<br><br>"); // Function to set the width, const logSerializedWidth = value => { divElement.style.width = value; // Set the width of the div using the provided value document.write(divElement.style.width); // Display the serialized width document.write("<br><br>"); }; // Call the function with different calculations logSerializedWidth("calc(1px / 0)"); // calc(infinity * 1px) logSerializedWidth("calc(1px / -0)"); // calc(-infinity * 1px) logSerializedWidth("calc(1px -infinity -infinity)"); // calc(infinity * 1px) logSerializedWidth("calc(1px -infinity infinity)"); // calc(-infinity * 1px) logSerializedWidth("calc(1px (NaN + 1))"); // calc(NaN 1px) </script> </body> </html>
To Continue Learning Please Login