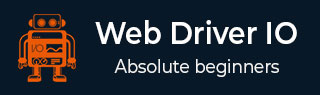
- WebdriverIO Tutorial
- WebdriverIO - Home
- WebdriverIO - Introduction
- WebdriverIO - Prerequisite
- WebdriverIO - Architecture
- WebdriverIO - Getting Started with NodeJS
- WebdriverIO - Installation of NPM
- WebdriverIO - VS Code Installation
- WebdriverIO - Package.json
- WebdriverIO - Mocha Installation
- Selenium Standalone Server Installation
- WebdriverIO - Configuration File generation
- WebdriverIO - VS Code Intellisense
- WebdriverIO - Wdio.conf.js file
- WebdriverIO - Xpath Locator
- WebdriverIO - CSS Locator
- WebdriverIO - Link Text Locator
- WebdriverIO - ID Locator
- WebdriverIO - Tag Name Locator
- WebdriverIO - Class Name Locator
- WebdriverIO - Name Locator
- Expect Statement for Assertions
- WebdriverIO - Happy Path Flow
- WebdriverIO - General Browser Commands
- WebdriverIO - Handling Browser Size
- WebdriverIO - Browser Navigation Commands
- Handling Checkboxes & Dropdowns
- WebdriverIO - Mouse Operations
- Handling Child Windows/Pop ups
- WebdriverIO - Hidden Elements
- WebdriverIO - Frames
- WebdriverIO - Drag & Drop
- WebdriverIO - Double Click
- WebdriverIO - Cookies
- WebdriverIO - Handling Radio Buttons
- Chai Assertions on webelements
- WebdriverIO - Multiple Windows/Tabs
- WebdriverIO - Scrolling Operations
- WebdriverIO - Alerts
- WebdriverIO - Debugging Code
- WebdriverIO - Capturing Screenshots
- WebdriverIO - JavaScript Executor
- WebdriverIO - Waits
- WebdriverIO - Running Tests in Parallel
- WebdriverIO - Data Driven Testing
- Running Tests from command-line parameters
- Execute Tests with Mocha Options
- Generate HTML reports from Allure
- WebdriverIO Useful Resources
- WebdriverIO - Quick Guide
- WebdriverIO - Useful Resources
- WebdriverIO - Discussion
WebdriverIO - Xpath Locator
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. We can create an xpath for an element for its identification. The rules to create a xpath expression are discussed below −
The syntax of xpath is
//tagname[@attribute='value']
Here, the tagname is optional.
For example,
//img[@alt='tutorialspoint']
Let us see the html code of the highlighted link - Home. The following screen will appear on your computer −
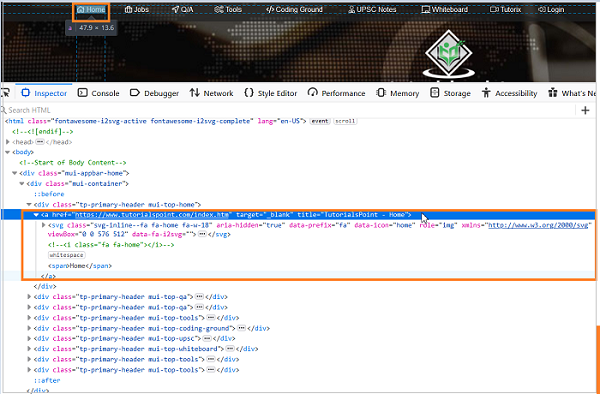
The xpath for element Home shall be as follows −
//a[@title='TutorialsPoint - Home'].
The following screen will appear on your computer −
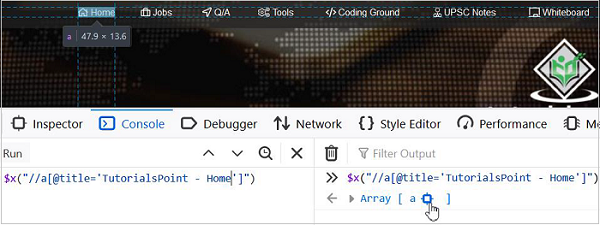
In the WebdriverIO code, we can specify the xpath expression of an element in the below format −
$('value of the xpath expression')
Or, we can store this expression in a variable −
const p = $('value of the xpath expression')
Let us identify the text highlighted in the below image and obtain its text −
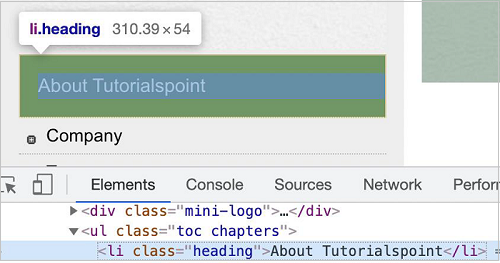
The xpath of the above highlighted element should be as follows −
//li[@class='heading']
To begin, follow Steps 1 to 5 from the Chapter titled Happy path flow with WebdriverIO which are as follows −
Step 1 − Install NodeJS. The details on how to perform this installation are given in detail in the Chapter titled Getting Started with NodeJS.
Step 2 − Install NPM. The details on how to perform this installation are given in detail in the Chapter titled Installation of NPM.
Step 3 − Install VS Code. The details on how to perform this installation are given in detail in the Chapter titled VS Code Installation.
Step 4 − Create the Configuration file. The details on how to perform this installation are given in detail in the Chapter titled Configuration File generation.
Step 5 − Create a spec file. The details on how to perform this installation are given in the Chapter titled Mocha Installation.
Step 6 − Add the below code within the Mocha spec file created.
// test suite name describe('Tutorialspoint application', function(){ //test case it('Identify element with Xpath', function(){ // launch url browser.url('https://www.tutorialspoint.com/about/about_careers.htm') //identify element with xpath then obtain text console.log($("//li[@class='heading']").getText() + " - is the text.") }); });
Run the Configuration file - wdio.conf.js file with the following command −
npx wdio run wdio.conf.js.
The details on how to create a Configuration file are discussed in detail in the Chapter titled Wdio.conf.js file and Chapter titled Configuration File generation
The following screen will appear on your computer −
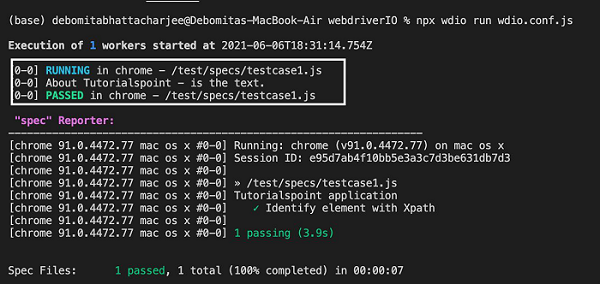
After the command has been executed successfully, the text of the element - About Tutorialspoint is printed in the console.
Xpath Locator with Text
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
We can create an xpath for an element for its identification. However, there are scenarios where there are no HTML attributes or tagname available to uniquely identify an element.
In such a situation, we can create an xpath for an element with the help of the text visible on the page by using the text function. The text function is case-sensitive.
The rule to create a xpath expression with visible text is discussed below −
The syntax of xpath is as follows −
//tagname[text()='displayed text'].
For example,
//li[text()='WebdriverIO']
Let us identify the element highlighted in the below image with the help of the visible text in xpath −
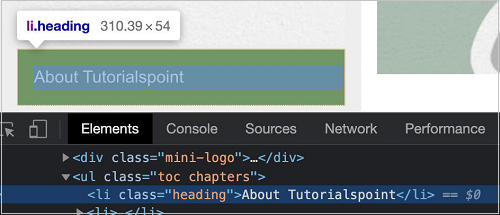
The xpath of the above highlighted element using the text() function shall be as follows −
//li[text()='About Tutorialspoint']
To begin, follow Steps 1 to 5 from the Chapter - Happy path flow with WebdriverIO which are as follows −
Step 1 − Install NodeJS. The details on how to perform this installation are given in detail in the Chapter titled Getting Started with NodeJS.
Step 2 − Install NPM. The details on how to perform this installation are given in detail in the Chapter titled Installation of NPM.
Step 3 − Install VS Code. The details on how to perform this installation are given in detail in the Chapter titled VS Code Installation.
Step 4 − Create the Configuration file. The details on how to perform this installation are given in detail in the Chapter titled Configuration File generation.
Step 5 − Create a spec file. The details on how to perform this installation are given in the Chapter titled Mocha Installation.
Step 6 − Add the below code within the Mocha spec file created.
// test suite name describe('Tutorialspoint application', function(){ //test case it('Identify element with Xpath - text()', function(){ // launch url browser.url('https://www.tutorialspoint.com/about/about_careers.htm') //identify element with xpath - visible text then obtain text console.log($("//li[text()='About Tutorialspoint']").getText() + " - is the text.") }); });
Run the Configuration file - wdio.conf.js file with the command −
npx wdio run wdio.conf.js.
The details on how to create a Configuration file are discussed in detail in the Chapter titledWdio.conf.js file and Chapter titled Configuration File generation.
The following screen will appear on your computer −
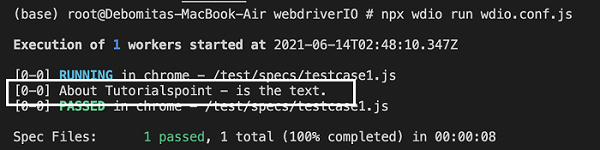
After the command has been executed successfully, the text of the element - About Tutorialspoint is printed in the console.