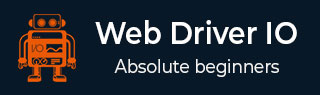
- WebdriverIO Tutorial
- WebdriverIO - Home
- WebdriverIO - Introduction
- WebdriverIO - Prerequisite
- WebdriverIO - Architecture
- WebdriverIO - Getting Started with NodeJS
- WebdriverIO - Installation of NPM
- WebdriverIO - VS Code Installation
- WebdriverIO - Package.json
- WebdriverIO - Mocha Installation
- Selenium Standalone Server Installation
- WebdriverIO - Configuration File generation
- WebdriverIO - VS Code Intellisense
- WebdriverIO - Wdio.conf.js file
- WebdriverIO - Xpath Locator
- WebdriverIO - CSS Locator
- WebdriverIO - Link Text Locator
- WebdriverIO - ID Locator
- WebdriverIO - Tag Name Locator
- WebdriverIO - Class Name Locator
- WebdriverIO - Name Locator
- Expect Statement for Assertions
- WebdriverIO - Happy Path Flow
- WebdriverIO - General Browser Commands
- WebdriverIO - Handling Browser Size
- WebdriverIO - Browser Navigation Commands
- Handling Checkboxes & Dropdowns
- WebdriverIO - Mouse Operations
- Handling Child Windows/Pop ups
- WebdriverIO - Hidden Elements
- WebdriverIO - Frames
- WebdriverIO - Drag & Drop
- WebdriverIO - Double Click
- WebdriverIO - Cookies
- WebdriverIO - Handling Radio Buttons
- Chai Assertions on webelements
- WebdriverIO - Multiple Windows/Tabs
- WebdriverIO - Scrolling Operations
- WebdriverIO - Alerts
- WebdriverIO - Debugging Code
- WebdriverIO - Capturing Screenshots
- WebdriverIO - JavaScript Executor
- WebdriverIO - Waits
- WebdriverIO - Running Tests in Parallel
- WebdriverIO - Data Driven Testing
- Running Tests from command-line parameters
- Execute Tests with Mocha Options
- Generate HTML reports from Allure
- WebdriverIO Useful Resources
- WebdriverIO - Quick Guide
- WebdriverIO - Useful Resources
- WebdriverIO - Discussion
WebdriverIO - Expect Statement for Assertions
To use WebdriverIO as an automation testing tool, we need to have checkpoints which will help us to conclude if our test has passed or failed. There are various assertions available in WebdriverIO with which we can verify if the test has successfully validated a step.
In assertion, we can compare an expected result of a test with an actual. If both are similar, a test should pass, else it should fail. The expect statement in WebdriverIO can be applied on the browser, a mock object, or an element.
We have to add a NodeJS library called Chai. Chai library contains the expect statement that is used for the Assertion.
We have to add the below statement in our code to implement the Chai Assertion −
const e = require('chai').expect
Assertions applied to browsers
These assertions are listed below −
toHaveUrl
It checks whether the browser has opened a particular page.The syntax is as follows −
expect(browser).toHaveUrl('https://www.tutorialspoint.com/index.htm')
toHaveUrlContaining
It checks whether the URL of a page has a particular value.
Syntax
The syntax is as follows −
expect(browser).toHaveUrlContaining('tutorialspoint')
toHaveUrl
It checks whether the page has a particular title.
Syntax
The syntax is as follows −
expect(browser).toHaveTitle('Terms of Use - Tutorialspoint')
Assertions applied on elements
These assertions are listed below −
toBeDisplayed
It checks whether an element is displayed.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBeDisplayed()
toExist
It checks whether an element exists.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toExist()
toBePresent
It checks whether an element is present.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBePresent()
toBeExisting
It is similar to toExist.
toBeFocussed
It checks whether an element is focused or not.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBeFocussed()
toHaveAttribute
It checks whether an element attribute has a particular value.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveAttribute('name', 'search')
toHaveAttr
It is similar to toExist.
toHaveAttributeContaining
It checks whether an element attribute contains a particular value.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveAttributeContaining('name', 'srch')
toHaveElementClass
It checks whether an element has a particular class name.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveElementClass('name', { message: 'Not available!', })
toHaveElementClassContaining
It checks whether an element class name contains a particular value.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveElementClassContaining('nam')
toHaveElementProperty
It checks whether an element has a particular property.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveElementProperty('width', 15) //verify negative scenario expect(e).not.toHaveElementProperty('width', 20)
toHaveValue
It checks whether an input element has a particular value.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveValue('Selenium', { ignoreCase: false})
toHaveValueContaining
It checks whether an input element contains a particular value
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveValueContaining('srch')
toBeClickable
It checks whether an element is clickable.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBeClickable()
toBeDisabled
It checks whether an element is disabled.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBeDisabled() //verify negative scenario expect(e).not.toBeEnabled()
toBeEnabled
It checks whether an element is enabled.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBeEnabled()
toBeSelected
It is the same as toBeEnabled.
toBeChecked
It is the same as toBeEnabled.
toHaveHref
It checks whether a link element has a particular link target.
Syntax
The syntax is as follows −
const e = $('<a>') expect(e).toHaveHref('https://www.tutorialspoint.com/index.htm')
toHaveLink
It is same as toHaveHref.
toHaveHrefContaining
It checks whether a link element contains a particular link target.
Syntax
The syntax is as follows −
const e = $('<a>') expect(e).toHaveHrefContaining('tutorialspoint.com')
toHaveLinkContaining
It is the same as HaveHrefContaining.
toHaveId
It checks whether an element has a particular id attribute value.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveId('loc')
toHaveText
It checks whether an element has a particular text.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveText('Learning WebdriverIO')
toHaveTextContaining
It checks whether an element contains a particular text.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toHaveTextContaining('Learning WebdriverIO')
toBeDisplayedInViewpoint
It checks whether an element is within the viewpoint.
Syntax
The syntax is as follows −
const e = $('#loc') expect(e).toBeDisplayedInViewpoint()
Assertions applied to mock objects
The assertions are listed below −
toBeRequested
It checks whether a mock was called.
Syntax
The syntax is as follows −
const m = browser.mock('**/api/list*') expect(m).toBeRequested()
toBeRequestedTimes
It checks whether a mock was called for an expected number of times.
Syntax
The syntax is as follows −
const m = browser.mock('**/api/list*') expect(m).toBeRequestedTimes(2)
To begin, follow the steps 1 to 5 from the Chapter titled Happy path flow with webdriverIO which are as follows −
Step 1 − Install NodeJS. The details on how to perform this installation are given in detail in the Chapter titled Getting Started with NodeJS.
Step 2 − Install NPM. The details on how to perform this installation are given in detail in the Chapter titled Installation of NPM.
Step 3 − Install VS Code. The details on how to perform this installation are given in detail in the Chapter titled VS Code Installation.
Step 4 − Create the Configuration file. The details on how to perform this installation are given in detail in the Chapter titled Configuration File generation.
Step 5 − Create a spec file. The details on how to perform this installation are given in the Chapter titled Mocha Installation.
Step 6 − Add the below code within the Mocha spec file created.
// test suite name describe('Tutorialspoint application', function(){ //test case it('Assertion with expect', function(){ // launch url browser.url('https://www.tutorialspoint.com/about/about_careers.htm') //identify element with link text then click $("=Terms of Use").click() browser.pause(1000) //verify page title with assertion expect(browser).toHaveTitleContaining('Terms of Use - Tuter') }); });
Run the Configuration file - wdio.conf.js file with the command −
npx wdio run wdio.conf.js
The details on how to create a Configuration file are discussed in detail in the Chapter titled Wdio.conf.js file and Chapter titled Configuration File generation.
The following screen will appear on your computer −
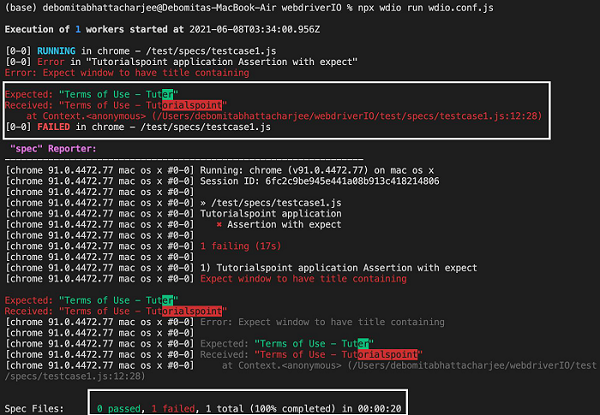
After the command has been executed successfully, we find the result as 1 failed. Since the Expected: is Terms of Use - Tuter and the Received: output is Terms of Use - Tutorialspoint.
Also, the WebdriverIO expect statement has highlighted the part of the text where the Expected: and the Received: texts are not matching.