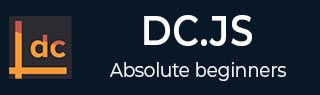
- DC.js Tutorial
- DC.js - Home
- DC.js - Introduction
- DC.js - Installation
- DC.js - Concepts
- Introduction to Crossfilter
- Introduction to D3.js
- DC.js - Mixins
- DC.js - baseMixin
- DC.js - capMixin
- DC.js - colorMixin
- DC.js - marginMixin
- DC.js - coordinateGridMixin
- DC.js - Pie Chart
- DC.js - Line Chart
- DC.js - Bar Chart
- DC.js - Composite Chart
- DC.js - Series Chart
- DC.js - Scatter Plot
- DC.js - Bubble Chart
- DC.js - Heat Map
- DC.js - Data Count
- DC.js - Data Table
- DC.js - Data Grid
- DC.js - Legend
- DC.js - Dashboard Working Example
- DC.js Useful Resources
- DC.js - Quick Guide
- DC.js - Useful Resources
- DC.js - Discussion
DC.js - Introduction to Crossfilter
Crossfilter is a multi-dimensional dataset. It supports extremely fast interaction with datasets containing a million or more records.
Basic Concepts
Crossfilter is defined under the crossfilter namespace. It uses semantic versioning. Consider a crossfilter object loaded with a collection of fruits that is defined below −
var fruits = crossfilter ([ { name: “Apple”, type: “fruit”, count: 20 }, { name: “Orange”, type: "fruit”, count: 10 }, { name: “Grapes”, type: “fruit”, count: 50 }, { name: “Mango”, type: “fruit”, count: 40 } ]);
If we need to perform the total records in a group, we can use the following function −
var count = fruits.groupAll().reduceCount().value();
If we want to filter by a specific type −
var filtering = fruits.dimension(function(d) { return d.type; }); filtering.filter(“Grapes”)
Similarly, we can perform grouping with Crossfilter. To do this, we can use the following function −
var grouping = filtering.group().reduceCount(); var first = grouping.top(2);
Hence, Crossfilter is built to be extremely fast. If you want to recalculate groups as filters are applied, it calculates incrementally. Crossfilter dimensions are very expensive.
Crossfilter API
Let us go through the notable Crossfilter APIs in detail.
crossfilter([records]) − It is used to construct a new crossfilter. If the record is specified, then it simultaneously adds the specified records. Records can be any array of JavaScript objects or primitives.
crossfilter.add(records) − Adds the specified records to the crossfilter.
crossfilter.remove() − Removes all records that match the current filters from the crossfilter.
crossfilter.size() − Returns the number of records in the crossfilter.
crossfilter.groupAll() − It is a function for grouping all records and reducing to a single value.
crossfilter.dimension(value) − It is used to construct a new dimension using the specified value accessor function.
dimension.filter(value) − It is used to filter records for dimension's match value, and returns the dimension.
dimension.filterRange(range) − Filters records for dimension's value that are greater than or equal to range[0], and less than range[1].
dimension.filterAll() − Clears any filters on this dimension.
dimension.top(k) − It is used to return a new array containing the top k records, according to the natural order of this dimension.
dimension.bottom(k) − It is used to return a new array containing the bottom k records, according to the natural order of this dimension.
dimension.dispose() − It is used to remove the dimension from the crossfilter.
In the next chapter, we will understand in brief about D3.js.