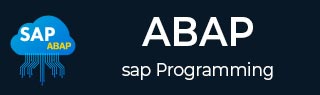
- SAP ABAP Tutorial
- SAP ABAP - Home
- SAP ABAP - Overview
- SAP ABAP - Environment
- SAP ABAP - Screen Navigation
- SAP ABAP - Basic Syntax
- SAP ABAP - Data Types
- SAP ABAP - Variables
- SAP ABAP - Constants & Literals
- SAP ABAP - Operators
- SAP ABAP - Loop Control
- SAP ABAP - Decisions
- SAP ABAP - Strings
- SAP ABAP - Date & Time
- SAP ABAP - Formatting Data
- SAP ABAP - Exception Handling
- SAP ABAP - Dictionary
- SAP ABAP - Domains
- SAP ABAP - Data Elements
- SAP ABAP - Tables
- SAP ABAP - Structures
- SAP ABAP - Views
- SAP ABAP - Search Help
- SAP ABAP - Lock Objects
- SAP ABAP - Modularization
- SAP ABAP - Subroutines
- SAP ABAP - Macros
- SAP ABAP - Function Modules
- SAP ABAP - Include Programs
- SAP ABAP - Open SQL Overview
- SAP ABAP - Native SQL Overview
- SAP ABAP - Internal Tables
- SAP ABAP - Creating Internal Tables
- ABAP - Populating Internal Tables
- SAP ABAP - Copying Internal Tables
- SAP ABAP - Reading Internal Tables
- SAP ABAP - Deleting Internal Tables
- SAP ABAP - Object Orientation
- SAP ABAP - Objects
- SAP ABAP - Classes
- SAP ABAP - Inheritance
- SAP ABAP - Polymorphism
- SAP ABAP - Encapsulation
- SAP ABAP - Interfaces
- SAP ABAP - Object Events
- SAP ABAP - Report Programming
- SAP ABAP - Dialog Programming
- SAP ABAP - Smart Forms
- SAP ABAP - SAPscripts
- SAP ABAP - Customer Exits
- SAP ABAP - User Exits
- SAP ABAP - Business Add-Ins
- SAP ABAP - Web Dynpro
- SAP ABAP Useful Resources
- SAP ABAP - Questions Answers
- SAP ABAP - Quick Guide
- SAP ABAP - Useful Resources
- SAP ABAP - Discussion
SAP ABAP - Populating Internal Tables
In internal tables, populating includes features such as selection, insertion and append. This chapter focuses on INSERT and APPEND statements.
INSERT Statement
INSERT statement is used to insert a single line or a group of lines into an internal table.
Following is the syntax to add a single line to an internal table −
INSERT <work_area_itab> INTO <internal_tab> INDEX <index_num>.
In this syntax, the INSERT statement inserts a new line in the internal_tab internal table. A new line can be inserted by using the work_area_itab INTO expression before the internal_tab parameter. When the work_area_itab INTO expression is used, the new line is taken from the work_area_itab work area and inserted into the internal_tab table. However, when the work_area_itab INTO expression is not used to insert a line, the line is taken from the header line of the internal_tab table.
When a new line is inserted in an internal table by using the INDEX clause, the index number of the lines after the inserted line is incremented by 1. If an internal table contains <index_num> - 1 lines, the new line is added at the end of the table. When the SAP system successfully adds a line to an internal table, the SY-SUBRC variable is set to 0.
Example
Following is a sample program that uses the insert statement.
REPORT ZCUSLIST1. DATA: BEGIN OF itable1 OCCURS 4, F1 LIKE SY-INDEX, END OF itable1. DO 4 TIMES. itable1-F1 = sy-index. APPEND itable1. ENDDO. itable1-F1 = -96. INSERT itable1 INDEX 2. LOOP AT itable1. Write / itable1-F1. ENDLOOP. LOOP AT itable1 Where F1 ≥ 3. itable1-F1 = -78. INSERT itable1. ENDLOOP. Skip. LOOP AT itable1. Write / itable1-F1. ENDLOOP.
The above code produces the following outp −
1 96- 2 3 4 1 96- 2 78- 3 78- 4
In the above example, the DO loop appends 4 rows containing the numbers 1 through 4 to it. The header line component itable1-F1 has been assigned a value of -96. Insert statement inserts the header line as new row into the body before row 3. The existing row 3 becomes row 4 after the insert. The LOOP AT statement retrieves those rows from the internal table that have an F1 value greater than or equal to 3. Before each row, Insert statement inserts a new row from the header line of it. Prior to the insert, the F1 component has been changed to contain -78.
After each insert statement is executed, the system re-indexes all rows below the one inserted. This introduces overhead when you insert rows near the top of a large internal table. If you need to insert a block of rows into a large internal table, prepare another table with the rows to be inserted and use insert lines instead.
When inserting a new row inside itable1 inside of a loop at itable1, it doesn’t affect the internal table instantly. It actually becomes effective on the next loop pass. While inserting a row after the current row, the table is re-indexed at the ENDLOOP. The sy-tabix is incremented and the next loop processes the row pointed to by sy-tabix. For instance, if you are in the second loop pass and you insert a record before row 3. When endloop is executed, the new row becomes row 3 and the old row 3 becomes row 4 and so on. Sy-tabix is incremented by 1, and the next loop pass processes the newly inserted record.
APPEND Statement
The APPEND statement is used to add a single row or line to an existing internal table. This statement copies a single line from a work area and inserts it after the last existing line in an internal table. The work area can be either a header line or any other field string with the same structure as a line of an internal table. Following is the syntax of the APPEND statement that is used to append a single line in an internal table −
APPEND <record_for_itab> TO <internal_tab>.
In this syntax, the <record_for_itab> expression can be represented by the <work_area_itab> work area, which is convertible to a line type or by the INITIAL LINE clause. If the user uses a <work_area_itab> work area, the SAP system adds a new line to the <internal_tab> internal table and populates it with the content of the work area. The INITIAL LINE clause appends a blank line that contains the initial value for each field of the table structure. After each APPEND statement, the SY-TABIX variable contains the index number of the appended line.
Appending lines to standard and sorted tables with a non-unique key works regardless of whether the lines with the same key already exist in the table. In other words, duplicate entries may occur. However, a run-time error occurs if the user attempts to add a duplicate entry to a sorted table with a unique key or if the user violates the sort order of a sorted table by appending the lines to it.
Example
REPORT ZCUSLIST1. DATA: BEGIN OF linv Occurs 0, Name(20) TYPE C, ID_Number TYPE I, END OF linv. DATA table1 LIKE TABLE OF linv. linv-Name = 'Melissa'. linv-ID_Number = 105467. APPEND linv TO table1. LOOP AT table1 INTO linv. Write: / linv-name, linv-ID_Number. ENDLOOP.
The above code produces the following output −
Melissa 105467