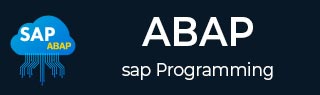
- SAP ABAP Tutorial
- SAP ABAP - Home
- SAP ABAP - Overview
- SAP ABAP - Environment
- SAP ABAP - Screen Navigation
- SAP ABAP - Basic Syntax
- SAP ABAP - Data Types
- SAP ABAP - Variables
- SAP ABAP - Constants & Literals
- SAP ABAP - Operators
- SAP ABAP - Loop Control
- SAP ABAP - Decisions
- SAP ABAP - Strings
- SAP ABAP - Date & Time
- SAP ABAP - Formatting Data
- SAP ABAP - Exception Handling
- SAP ABAP - Dictionary
- SAP ABAP - Domains
- SAP ABAP - Data Elements
- SAP ABAP - Tables
- SAP ABAP - Structures
- SAP ABAP - Views
- SAP ABAP - Search Help
- SAP ABAP - Lock Objects
- SAP ABAP - Modularization
- SAP ABAP - Subroutines
- SAP ABAP - Macros
- SAP ABAP - Function Modules
- SAP ABAP - Include Programs
- SAP ABAP - Open SQL Overview
- SAP ABAP - Native SQL Overview
- SAP ABAP - Internal Tables
- SAP ABAP - Creating Internal Tables
- ABAP - Populating Internal Tables
- SAP ABAP - Copying Internal Tables
- SAP ABAP - Reading Internal Tables
- SAP ABAP - Deleting Internal Tables
- SAP ABAP - Object Orientation
- SAP ABAP - Objects
- SAP ABAP - Classes
- SAP ABAP - Inheritance
- SAP ABAP - Polymorphism
- SAP ABAP - Encapsulation
- SAP ABAP - Interfaces
- SAP ABAP - Object Events
- SAP ABAP - Report Programming
- SAP ABAP - Dialog Programming
- SAP ABAP - Smart Forms
- SAP ABAP - SAPscripts
- SAP ABAP - Customer Exits
- SAP ABAP - User Exits
- SAP ABAP - Business Add-Ins
- SAP ABAP - Web Dynpro
- SAP ABAP Useful Resources
- SAP ABAP - Questions Answers
- SAP ABAP - Quick Guide
- SAP ABAP - Useful Resources
- SAP ABAP - Discussion
SAP ABAP - Encapsulation
Encapsulation is an Object Oriented Programming (OOP) concept that binds together data and functions that manipulate the data, and keeps both safe from outside interference and misuse. Data encapsulation led to the important OOP concept of data hiding. Encapsulation is a mechanism of bundling the data and the functions that use them, and data abstraction is a mechanism of exposing only the interfaces and hiding the implementation details from the user.
ABAP supports the properties of encapsulation and data hiding through the creation of user-defined types called classes. As discussed earlier, a class can contain private, protected and public members. By default, all items defined in a class are private.
Encapsulation by Interface
Encapsulation actually means one attribute and method could be modified in different classes. Hence data and method can have different form and logic that can be hidden to separate class.
Let's consider encapsulation by interface. Interface is used when we need to create one method with different functionality in different classes. Here the name of the method need not be changed. The same method will have to be implemented in different class implementations.
Example
The following program contains an Interface inter_1. We have declared attribute and a method method1. We have also defined two classes like Class1 and Class2. So we have to implement the method ‘method1’ in both of the class implementations. We have implemented the method ‘method1’ differently in different classes. In the start-ofselection, we create two objects Object1 and Object2 for two classes. Then, we call the method by different objects to get the function declared in separate classes.
Report ZEncap1. Interface inter_1. Data text1 Type char35. Methods method1. EndInterface. CLASS Class1 Definition. PUBLIC Section. Interfaces inter_1. ENDCLASS. CLASS Class2 Definition. PUBLIC Section. Interfaces inter_1. ENDCLASS. CLASS Class1 Implementation. Method inter_1~method1. inter_1~text1 = 'Class 1 Interface method'. Write / inter_1~text1. EndMethod. ENDCLASS. CLASS Class2 Implementation. Method inter_1~method1. inter_1~text1 = 'Class 2 Interface method'. Write / inter_1~text1. EndMethod. ENDCLASS. Start-Of-Selection. Data: Object1 Type Ref To Class1, Object2 Type Ref To Class2. Create Object: Object1, Object2. CALL Method: Object1→inter_1~method1, Object2→inter_1~method1.
The above code produces the following output −
Class 1 Interface method Class 2 Interface method
Encapsulated classes do not have a lot of dependencies on the outside world. Moreover, the interactions that they do have with external clients are controlled through a stabilized public interface. That is, an encapsulated class and its clients are loosely coupled. For the most part, classes with well-defined interfaces can be plugged into another context. When designed correctly, encapsulated classes become reusable software assets.
Designing Strategy
Most of us have learned through bitter experience to make class members private by default unless we really need to expose them. That is just good encapsulation. This wisdom is applied most frequently to data members and it also applies equally to all members.