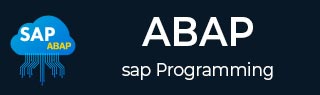
- SAP ABAP Tutorial
- SAP ABAP - Home
- SAP ABAP - Overview
- SAP ABAP - Environment
- SAP ABAP - Screen Navigation
- SAP ABAP - Basic Syntax
- SAP ABAP - Data Types
- SAP ABAP - Variables
- SAP ABAP - Constants & Literals
- SAP ABAP - Operators
- SAP ABAP - Loop Control
- SAP ABAP - Decisions
- SAP ABAP - Strings
- SAP ABAP - Date & Time
- SAP ABAP - Formatting Data
- SAP ABAP - Exception Handling
- SAP ABAP - Dictionary
- SAP ABAP - Domains
- SAP ABAP - Data Elements
- SAP ABAP - Tables
- SAP ABAP - Structures
- SAP ABAP - Views
- SAP ABAP - Search Help
- SAP ABAP - Lock Objects
- SAP ABAP - Modularization
- SAP ABAP - Subroutines
- SAP ABAP - Macros
- SAP ABAP - Function Modules
- SAP ABAP - Include Programs
- SAP ABAP - Open SQL Overview
- SAP ABAP - Native SQL Overview
- SAP ABAP - Internal Tables
- SAP ABAP - Creating Internal Tables
- ABAP - Populating Internal Tables
- SAP ABAP - Copying Internal Tables
- SAP ABAP - Reading Internal Tables
- SAP ABAP - Deleting Internal Tables
- SAP ABAP - Object Orientation
- SAP ABAP - Objects
- SAP ABAP - Classes
- SAP ABAP - Inheritance
- SAP ABAP - Polymorphism
- SAP ABAP - Encapsulation
- SAP ABAP - Interfaces
- SAP ABAP - Object Events
- SAP ABAP - Report Programming
- SAP ABAP - Dialog Programming
- SAP ABAP - Smart Forms
- SAP ABAP - SAPscripts
- SAP ABAP - Customer Exits
- SAP ABAP - User Exits
- SAP ABAP - Business Add-Ins
- SAP ABAP - Web Dynpro
- SAP ABAP Useful Resources
- SAP ABAP - Questions Answers
- SAP ABAP - Quick Guide
- SAP ABAP - Useful Resources
- SAP ABAP - Discussion
SAP ABAP - Interfaces
Similar to classes in ABAP, interfaces act as data types for objects. The components of interfaces are same as the components of classes. Unlike the declaration of classes, the declaration of an interface does not include the visibility sections. This is because the components defined in the declaration of an interface are always integrated in the public visibility section of the classes.
Interfaces are used when two similar classes have a method with the same name, but the functionalities are different from each other. Interfaces might appear similar to classes, but the functions defined in an interface are implemented in a class to extend the scope of that class. Interfaces along with the inheritance feature provide a base for polymorphism. This is because a method defined in an interface can behave differently in different classes.
Following is the general format to create an interface −
INTERFACE <intf_name>. DATA..... CLASS-DATA..... METHODS..... CLASS-METHODS..... ENDINTERFACE.
In this syntax, <intf_name> represents the name of an interface. The DATA and CLASSDATA statements can be used to define the instance and static attributes of the interface respectively. The METHODS and CLASS-METHODS statements can be used to define the instance and static methods of the interface respectively. As the definition of an interface does not include the implementation class, it is not necessary to add the DEFINITION clause in the declaration of an interface.
Note − All the methods of an interface are abstract. They are fully declared including their parameter interface, but not implemented in the interface. All the classes that want to use an interface must implement all the methods of the interface. Otherwise, the class becomes an abstract class.
We use the following syntax in the implementation part of the class −
INTERFACE <intf_name>.
In this syntax, <intf_name> represents the name of an interface. Note that this syntax must be used in the public section of the class.
The following syntax is used to implement the methods of an interface inside the implementation of a class −
METHOD <intf_name~method_m>. <statements>. ENDMETHOD.
In this syntax, <intf_name~method_m> represents the fully declared name of a method of the <intf_name> interface.
Example
Report ZINTERFACE1. INTERFACE my_interface1. Methods msg. ENDINTERFACE. CLASS num_counter Definition. PUBLIC Section. INTERFACES my_interface1. Methods add_number. PRIVATE Section. Data num Type I. ENDCLASS. CLASS num_counter Implementation. Method my_interface1~msg. Write: / 'The number is', num. EndMethod. Method add_number. ADD 7 TO num. EndMethod. ENDCLASS. CLASS drive1 Definition. PUBLIC Section. INTERFACES my_interface1. Methods speed1. PRIVATE Section. Data wheel1 Type I. ENDCLASS. CLASS drive1 Implementation. Method my_interface1~msg. Write: / 'Total number of wheels is', wheel1. EndMethod. Method speed1. Add 4 To wheel1. EndMethod. ENDCLASS. Start-Of-Selection. Data object1 Type Ref To num_counter. Create Object object1. CALL Method object1→add_number. CALL Method object1→my_interface1~msg. Data object2 Type Ref To drive1. Create Object object2. CALL Method object2→speed1. CALL Method object2→my_interface1~msg.
The above code produces the following output −
The number is 7 Total number of wheels is 4
In the above example, my_interface1 is the name of an interface that contains the 'msg' method. Next, two classes, num_counter and drive1 are defined and implemented. Both these classes implement the 'msg' method and also specific methods that define the behavior of their respective instances, such as the add_number and speed1 methods.
Note − The add_number and speed1 methods are specific to the respective classes.