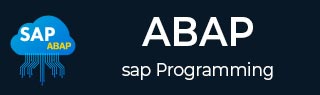
- SAP ABAP Tutorial
- SAP ABAP - Home
- SAP ABAP - Overview
- SAP ABAP - Environment
- SAP ABAP - Screen Navigation
- SAP ABAP - Basic Syntax
- SAP ABAP - Data Types
- SAP ABAP - Variables
- SAP ABAP - Constants & Literals
- SAP ABAP - Operators
- SAP ABAP - Loop Control
- SAP ABAP - Decisions
- SAP ABAP - Strings
- SAP ABAP - Date & Time
- SAP ABAP - Formatting Data
- SAP ABAP - Exception Handling
- SAP ABAP - Dictionary
- SAP ABAP - Domains
- SAP ABAP - Data Elements
- SAP ABAP - Tables
- SAP ABAP - Structures
- SAP ABAP - Views
- SAP ABAP - Search Help
- SAP ABAP - Lock Objects
- SAP ABAP - Modularization
- SAP ABAP - Subroutines
- SAP ABAP - Macros
- SAP ABAP - Function Modules
- SAP ABAP - Include Programs
- SAP ABAP - Open SQL Overview
- SAP ABAP - Native SQL Overview
- SAP ABAP - Internal Tables
- SAP ABAP - Creating Internal Tables
- ABAP - Populating Internal Tables
- SAP ABAP - Copying Internal Tables
- SAP ABAP - Reading Internal Tables
- SAP ABAP - Deleting Internal Tables
- SAP ABAP - Object Orientation
- SAP ABAP - Objects
- SAP ABAP - Classes
- SAP ABAP - Inheritance
- SAP ABAP - Polymorphism
- SAP ABAP - Encapsulation
- SAP ABAP - Interfaces
- SAP ABAP - Object Events
- SAP ABAP - Report Programming
- SAP ABAP - Dialog Programming
- SAP ABAP - Smart Forms
- SAP ABAP - SAPscripts
- SAP ABAP - Customer Exits
- SAP ABAP - User Exits
- SAP ABAP - Business Add-Ins
- SAP ABAP - Web Dynpro
- SAP ABAP Useful Resources
- SAP ABAP - Questions Answers
- SAP ABAP - Quick Guide
- SAP ABAP - Useful Resources
- SAP ABAP - Discussion
SAP ABAP - Operators
ABAP provides a rich set of operators to manipulate variables. All ABAP operators are classified into four categories −
- Arithmetic Operators
- Comparison Operators
- Bitwise Operators
- Character String Operators
Arithmetic Operators
Arithmetic operators are used in mathematical expressions in the same way that they are used in algebra. The following list describes arithmetic operators. Assume integer variable A holds 20 and variable B holds 40.
S.No. | Arithmetic Operator & Description |
---|---|
1 | + (Addition) Adds values on either side of the operator. Example: A + B will give 60. |
2 | − (Subtraction) Subtracts right hand operand from left hand operand. Example: A − B will give -20. |
3 | * (Multiplication) Multiplies values on either side of the operator. Example: A * B will give 800. |
4 | / (Division) Divides left hand operand by right hand operand. Example: B / A will give 2. |
5 | MOD (Modulus) Divides left hand operand by right hand operand and returns the remainder. Example: B MOD A will give 0. |
Example
REPORT YS_SEP_08. DATA: A TYPE I VALUE 150, B TYPE I VALUE 50, Result TYPE I. Result = A / B. WRITE / Result.
The above code produces the following output −
3
Comparison Operators
Let’s discuss the various types of comparison operators for different operands.
S.No. | Comparison Operator & Description |
---|---|
1 | = (equality test). Alternate form is EQ. Checks if the values of two operands are equal or not, if yes then condition becomes true. Example (A = B) is not true. |
2 | <> (Inequality test). Alternate form is NE. Checks if the values of two operands are equal or not. If the values are not equal then the condition becomes true. Example (A <> B) is true. |
3 | > (Greater than test). Alternate form is GT. Checks if the value of left operand is greater than the value of right operand. If yes then condition becomes true. Example (A > B) is not true. |
4 | < (Less than test). Alternate form is LT. Checks if the value of left operand is less than the value of right operand. If yes, then condition becomes true. Example (A < B) is true. |
5 | >= (Greater than or equals) Alternate form is GE. Checks if the value of left operand is greater than or equal to the value of right Operand. If yes, then condition becomes true. Example (A >= B) is not true. |
6 | <= (Less than or equals test). Alternate form is LE. Checks if the value of left operand is less than or equal to the value of right operand. If yes, then condition becomes true. Example (A <= B) is true. |
7 | a1 BETWEEN a2 AND a3 (Interval test) Checks whether a1 lies in between a2 and a3 (inclusive). If yes, then the condition becomes true. Example (A BETWEEN B AND C) is true. |
8 | IS INITIAL The condition becomes true if the contents of the variable have not changed and it has been automatically assigned its initial value. Example (A IS INITIAL) is not true |
9 | IS NOT INITIAL The condition becomes true if the contents of the variable have changed. Example (A IS NOT INITIAL) is true. |
Note − If the data type or length of the variables does not match then automatic conversion is performed. Automatic type adjustment is performed for either one or both of the values while comparing two values of different data types. The conversion type is decided by the data type and the preference order of the data type.
Following is the order of preference −
If one field is of type I, then the other is converted to type I.
If one field is of type P, then the other is converted to type P.
If one field is of type D, then the other is converted to type D. But C and N types are not converted and they are compared directly. Similar is the case with type T.
If one field is of type N and the other is of type C or X, both the fields are converted to type P.
If one field is of type C and the other is of type X, the X type is converted to type C.
Example 1
REPORT YS_SEP_08. DATA: A TYPE I VALUE 115, B TYPE I VALUE 119. IF A LT B. WRITE: / 'A is less than B'. ENDIF
The above code produces the following output −
A is less than B
Example 2
REPORT YS_SEP_08. DATA: A TYPE I. IF A IS INITIAL. WRITE: / 'A is assigned'. ENDIF.
The above code produces the following output −
A is assigned.
Bitwise Operators
ABAP also provides a series of bitwise logical operators that can be used to build Boolean algebraic expressions. The bitwise operators can be combined in complex expressions using parentheses and so on.
S.No. | Bitwise Operator & Description |
---|---|
1 | BIT-NOT Unary operator that flips all the bits in a hexadecimal number to the opposite value. For instance, applying this operator to a hexadecimal number having the bit level value 10101010 (e.g. 'AA') would give 01010101. |
2 | BIT-AND This binary operator compares each field bit by bit using the Boolean AND operator. |
3 | BIT-XOR Binary operator that compares each field bit by bit using the Boolean XOR (exclusive OR) operator. |
4 | BIT-OR Binary operator that compares each field bit by bit using the Boolean OR operator. |
For example, following is the truth table that shows the values generated when applying the Boolean AND, OR, or XOR operators against the two bit values contained in field A and field B.
Field A | Field B | AND | OR | XOR |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
Character String Operators
Following is a list of character string operators −
S.No. | Character String Operator & Description |
---|---|
1 | CO (Contains Only) Checks whether A is solely composed of the characters in B. |
2 | CN (Not Contains ONLY) Checks whether A contains characters that are not in B. |
3 | CA (Contains ANY) Checks whether A contains at least one character of B. |
4 | NA (NOT Contains Any) Checks whether A does not contain any character of B. |
5 | CS (Contains a String) Checks whether A contains the character string B. |
6 | NS (NOT Contains a String) Checks whether A does not contain the character string B. |
7 | CP (Contains a Pattern) It checks whether A contains the pattern in B. |
8 | NP (NOT Contains a Pattern) It checks whether A does not contain the pattern in B. |
Example
REPORT YS_SEP_08. DATA: P(10) TYPE C VALUE 'APPLE', Q(10) TYPE C VALUE 'CHAIR'. IF P CA Q. WRITE: / 'P contains at least one character of Q'. ENDIF.
The above code produces the following output −
P contains at least one character of Q.