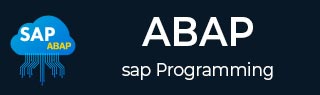
- SAP ABAP Tutorial
- SAP ABAP - Home
- SAP ABAP - Overview
- SAP ABAP - Environment
- SAP ABAP - Screen Navigation
- SAP ABAP - Basic Syntax
- SAP ABAP - Data Types
- SAP ABAP - Variables
- SAP ABAP - Constants & Literals
- SAP ABAP - Operators
- SAP ABAP - Loop Control
- SAP ABAP - Decisions
- SAP ABAP - Strings
- SAP ABAP - Date & Time
- SAP ABAP - Formatting Data
- SAP ABAP - Exception Handling
- SAP ABAP - Dictionary
- SAP ABAP - Domains
- SAP ABAP - Data Elements
- SAP ABAP - Tables
- SAP ABAP - Structures
- SAP ABAP - Views
- SAP ABAP - Search Help
- SAP ABAP - Lock Objects
- SAP ABAP - Modularization
- SAP ABAP - Subroutines
- SAP ABAP - Macros
- SAP ABAP - Function Modules
- SAP ABAP - Include Programs
- SAP ABAP - Open SQL Overview
- SAP ABAP - Native SQL Overview
- SAP ABAP - Internal Tables
- SAP ABAP - Creating Internal Tables
- ABAP - Populating Internal Tables
- SAP ABAP - Copying Internal Tables
- SAP ABAP - Reading Internal Tables
- SAP ABAP - Deleting Internal Tables
- SAP ABAP - Object Orientation
- SAP ABAP - Objects
- SAP ABAP - Classes
- SAP ABAP - Inheritance
- SAP ABAP - Polymorphism
- SAP ABAP - Encapsulation
- SAP ABAP - Interfaces
- SAP ABAP - Object Events
- SAP ABAP - Report Programming
- SAP ABAP - Dialog Programming
- SAP ABAP - Smart Forms
- SAP ABAP - SAPscripts
- SAP ABAP - Customer Exits
- SAP ABAP - User Exits
- SAP ABAP - Business Add-Ins
- SAP ABAP - Web Dynpro
- SAP ABAP Useful Resources
- SAP ABAP - Questions Answers
- SAP ABAP - Quick Guide
- SAP ABAP - Useful Resources
- SAP ABAP - Discussion
SAP ABAP - Polymorphism
The term polymorphism literally means ‘many forms’. From an object-oriented perspective, polymorphism works in conjunction with inheritance to make it possible for various types within an inheritance tree to be used interchangeably. That is, polymorphism occurs when there is a hierarchy of classes and they are related by inheritance. ABAP polymorphism means that a call to a method will cause a different method to be executed depending on the type of object that invokes the method.
The following program contains an abstract class 'class_prgm', 2 sub classes (class_procedural and class_OO), and a test driver class 'class_type_approach'. In this implementation, the class method 'start' allow us to display the type of programming and its approach. If you look closely at the signature of method 'start', you will observe that it receives an importing parameter of type class_prgm. However, in the Start-Of-Selection event, this method has been called at run-time with objects of type class_procedural and class_OO.
Example
Report ZPolymorphism1. CLASS class_prgm Definition Abstract. PUBLIC Section. Methods: prgm_type Abstract, approach1 Abstract. ENDCLASS. CLASS class_procedural Definition Inheriting From class_prgm. PUBLIC Section. Methods: prgm_type Redefinition, approach1 Redefinition. ENDCLASS. CLASS class_procedural Implementation. Method prgm_type. Write: 'Procedural programming'. EndMethod. Method approach1. Write: 'top-down approach'. EndMethod. ENDCLASS. CLASS class_OO Definition Inheriting From class_prgm. PUBLIC Section. Methods: prgm_type Redefinition, approach1 Redefinition. ENDCLASS. CLASS class_OO Implementation. Method prgm_type. Write: 'Object oriented programming'. EndMethod. Method approach1. Write: 'bottom-up approach'. EndMethod. ENDCLASS. CLASS class_type_approach Definition. PUBLIC Section. CLASS-METHODS: start Importing class1_prgm Type Ref To class_prgm. ENDCLASS. CLASS class_type_approach IMPLEMENTATION. Method start. CALL Method class1_prgm→prgm_type. Write: 'follows'. CALL Method class1_prgm→approach1. EndMethod. ENDCLASS. Start-Of-Selection. Data: class_1 Type Ref To class_procedural, class_2 Type Ref To class_OO. Create Object class_1. Create Object class_2. CALL Method class_type_approach⇒start Exporting class1_prgm = class_1. New-Line. CALL Method class_type_approach⇒start Exporting class1_prgm = class_2.
The above code produces the following output −
Procedural programming follows top-down approach Object oriented programming follows bottom-up approach
ABAP run-time environment performs an implicit narrowing cast during the assignment of the importing parameter class1_prgm. This feature helps the 'start' method to be implemented generically. The dynamic type information associated with an object reference variable allows the ABAP run-time environment to dynamically bind a method call with the implementation defined in the object pointed to by the object reference variable. For instance, the importing parameter 'class1_prgm' for method 'start' in the 'class_type_approach' class refers to an abstract type that could never be instantiated on its own.
Whenever the method is called with a concrete sub class implementation such as class_procedural or class_OO, the dynamic type of the class1_prgm reference parameter is bound to one of these concrete types. Therefore, the calls to methods 'prgm_type' and 'approach1' refer to the implementations provided in the class_procedural or class_OO sub classes rather than the undefined abstract implementations provided in class 'class_prgm'.