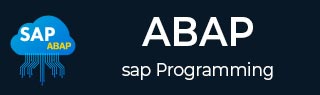
- SAP ABAP Tutorial
- SAP ABAP - Home
- SAP ABAP - Overview
- SAP ABAP - Environment
- SAP ABAP - Screen Navigation
- SAP ABAP - Basic Syntax
- SAP ABAP - Data Types
- SAP ABAP - Variables
- SAP ABAP - Constants & Literals
- SAP ABAP - Operators
- SAP ABAP - Loop Control
- SAP ABAP - Decisions
- SAP ABAP - Strings
- SAP ABAP - Date & Time
- SAP ABAP - Formatting Data
- SAP ABAP - Exception Handling
- SAP ABAP - Dictionary
- SAP ABAP - Domains
- SAP ABAP - Data Elements
- SAP ABAP - Tables
- SAP ABAP - Structures
- SAP ABAP - Views
- SAP ABAP - Search Help
- SAP ABAP - Lock Objects
- SAP ABAP - Modularization
- SAP ABAP - Subroutines
- SAP ABAP - Macros
- SAP ABAP - Function Modules
- SAP ABAP - Include Programs
- SAP ABAP - Open SQL Overview
- SAP ABAP - Native SQL Overview
- SAP ABAP - Internal Tables
- SAP ABAP - Creating Internal Tables
- ABAP - Populating Internal Tables
- SAP ABAP - Copying Internal Tables
- SAP ABAP - Reading Internal Tables
- SAP ABAP - Deleting Internal Tables
- SAP ABAP - Object Orientation
- SAP ABAP - Objects
- SAP ABAP - Classes
- SAP ABAP - Inheritance
- SAP ABAP - Polymorphism
- SAP ABAP - Encapsulation
- SAP ABAP - Interfaces
- SAP ABAP - Object Events
- SAP ABAP - Report Programming
- SAP ABAP - Dialog Programming
- SAP ABAP - Smart Forms
- SAP ABAP - SAPscripts
- SAP ABAP - Customer Exits
- SAP ABAP - User Exits
- SAP ABAP - Business Add-Ins
- SAP ABAP - Web Dynpro
- SAP ABAP Useful Resources
- SAP ABAP - Questions Answers
- SAP ABAP - Quick Guide
- SAP ABAP - Useful Resources
- SAP ABAP - Discussion
SAP ABAP - Constants & Literals
Literals are unnamed data objects that you create within the source code of a program. They are fully defined by their value. You can’t change the value of a literal. Constants are named data objects created statically by using declarative statements. A constant is declared by assigning a value to it that is stored in the program's memory area. The value assigned to a constant can’t be changed during the execution of the program. These fixed values can also be considered as literals. There are two types of literals − numeric and character.
Numeric Literals
Number literals are sequences of digits which can have a prefixed sign. In number literals, there are no decimal separators and no notation with mantissa and exponent.
Following are some examples of numeric literals −
183. -97. +326.
Character Literals
Character literals are sequences of alphanumeric characters in the source code of an ABAP program enclosed in single quotation marks. Character literals enclosed in quotation marks have the predefined ABAP type C and are described as text field literals. Literals enclosed in “back quotes” have the ABAP type STRING and are described as string literals. The field length is defined by the number of characters.
Note − In text field literals, trailing blanks are ignored, but in string literals they are taken into account.
Following are some examples of character literals.
Text field literals
REPORT YR_SEP_12. Write 'Tutorials Point'. Write / 'ABAP Tutorial'.
String field literals
REPORT YR_SEP_12. Write `Tutorials Point `. Write / `ABAP Tutorial `.
The output is same in both the above cases −
Tutorials Point ABAP Tutorial
Note − When we try to change the value of the constant, a syntax or run-time error may occur. Constants that you declare in the declaration part of a class or an interface belong to the static attributes of that class or interface.
CONSTANTS Statement
We can declare the named data objects with the help of CONSTANTS statement.
Following is the syntax −
CONSTANTS <f> TYPE <type> VALUE <val>.
The CONSTANTS statement is similar to the DATA statement.
<f> specifies a name for the constant. TYPE <type> represents a constant named <f>, which inherits the same technical attributes as the existing data type <type>. VALUE <val> assigns an initial value to the declared constant name <f>.
Note − We should use the VALUE clause in the CONSTANTS statement. The clause ‘VALUE’ is used to assign an initial value to the constant during its declaration.
We have 3 types of constants such as elementary, complex and reference constants. The following statement shows how to define constants by using the CONSTANTS statement −
REPORT YR_SEP_12. CONSTANTS PQR TYPE P DECIMALS 4 VALUE '1.2356'. Write: / 'The value of PQR is:', PQR.
The output is −
The value of PQR is: 1.2356
Here it refers to elementary data type and is known as elementary constant.
Following is an example for complex constants −
BEGIN OF EMPLOYEE, Name(25) TYPE C VALUE 'Management Team', Organization(40) TYPE C VALUE 'Tutorials Point Ltd', Place(10) TYPE C VALUE 'India', END OF EMPLOYEE.
In the above code snippet, EMPLOYEE is a complex constant that is composed of the Name, Organization and Place fields.
The following statement declares a constant reference −
CONSTANTS null_pointer TYPE REF TO object VALUE IS INITIAL.
We can use the constant reference in comparisons or we may pass it on to procedures.