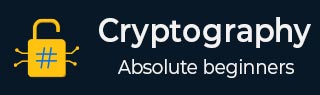
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Symmetric Algorithms
- Data Encryption Standard
- Triple DES
- Advanced Encryption Standard
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Feistel Block Cipher
A framework or design model called the Feistel cipher which is used to create different symmetric block ciphers, including DES. Components of this design framework can either be self-invertible, non-invertible, or invertible. The encryption and decryption algorithms are also the same as those used by the Feistel block cipher.
The Feistel structure demonstrates the implementation processes of confusion and diffusion and is based on the Shannon structure that was first described in 1945. Using a substitution method, confusion creates a complex relationship between the encryption key and the ciphertext. But diffusion uses a permutation process for creating a complex link between plaintext and cipher text.
The framework for implementing substitution and permutation alternately was proposed by the Feistel cipher. Substitution uses ciphertext to take the place of plaintext elements. Instead of having one element replace another as is done with substitution, permutation rearranges the elements of plaintext.
Algorithm
Make a list of every character in plaintext.
After converting the plaintext to ascii, format it in 8-bit binary.
Separate the binary plaintext string into its left (L1) and right (R1) parts.
For each of the two rounds, generate two random binary keys (K1 and K2), each of equal length to the plaintext.
Encryption
There are multiple rounds of processing plaintext in the Feistel cipher encryption process. The substitution step and the permutation step are included in every round. Take a look at the example that following, which describes the encryption structure used in this design framework.

Step 1 − The plaintext is split up into fixed-size blocks, and only one block is handled at a time in this initial phase. Two inputs for the encryption technique are a block of plaintext and a key K.
Step 2 − Split the block of plaintext into two parts. The plaintext block will have two distinct representations: LE0 for the left half of the block and RE0 for the right half. To create the ciphertext block, the two parts of the plaintext block (LE0 and RE0) will undergo multiple rounds of plaintext processing.
The encryption function is applied to the key Ki as well as the right half REi of the plaintext block for each round. Next, the left half of LEj is XORed with the function results. In cryptography, the logical operator XOR is used to compare two input bits and generate one output bit. For the following round, RE i+1, the output of the XOR function becomes the new right half. For the next round, the left half LEi+1 replaces the prior right half REi.
The same function, which implements a substitution function by applying the round function to the right half of the plaintext block, will be executed on each round. The left half of the block is used to XOR the function's output. After that, the two halves are switched using a permutation function. The next round's permutation results are given. Actually, the Feistel cipher model resembles the previously discussed Shannon structure in that it uses the substitution and permutation processes in an alternating manner.
Feistel Cipher Design Features
When using block ciphers, the following Feistel cipher design features are taken into account −
Block size − Larger block sizes are considered to make block ciphers more secure. Larger block sizes, but it slow down how quickly the encryption and decryption processes execute. Block ciphers typically contain 64-bit blocks, while more recent versions, such as AES (Advanced Encryption Standard), have 128-bit blocks.
Simple analysis − By making block ciphers simple to analyze, cryptanalytic vulnerabilities can be found and fixed, leading to the development of strong algorithms.
Key size − Similar to block size, higher key sizes are considered to be more secure, but they can additionally cause the encryption and decryption process to take time to complete. The previous 64-bit key has been replaced by a 128−bit key in modern ciphers.
The quantity of rounds − The quantity of rounds has an effect on a block cipher's security as well. More rounds boost security, but they also make the encryption harder to crack. The number of rounds therefore depends on the kind of data protection that a firm wants.
Round function − An complex round function increases the security of the block cipher.
Subkey generation function − Expert cryptanalysts find it more challenging to decrypt ciphers with more complex subkey generating functions.
Fast software encryption and decryption − It is advantageous to use software that may boost block ciphers' rate of execution.
Decryption
The fact that the Feistel cipher model uses the same algorithm for both encryption and decryption may surprise you. A few important guidelines to keep in mind when decrypting are as follows −
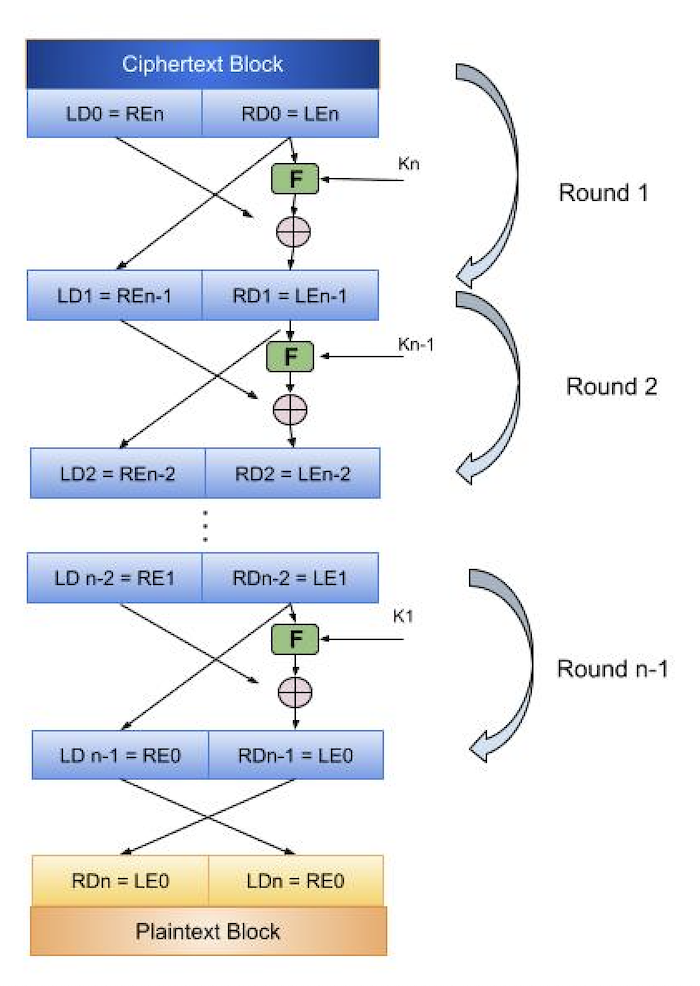
The encrypted text block is divided into two parts, the left (LD0) and the right (RD0), as seen in the above picture.
The round function is used with the key Kn-1 to operate on the right half of the cipher block, just like the encryption algorithm. The left half of the ciphertext block is XORed with the function's result. The output of the XOR function becomes the new right half (RD1), and RD0 swaps places with LD0 for the subsequent cycle. In fact, the identical function is used in each round, and the plaintext block is reached after a certain number of rounds are completed.
Implementation in Python
Let us implement the Feistel Block Cipher with the help of Python's binascii and random modules −
The Feistel Cipher algorithm for encryption and decryption is shown using this Python program. To recover the original plaintext, it first encrypts the input, which it then decrypts.
Example
import binascii import random def random_key(p): key = "" p = int(p) for _ in range(p): temp = random.randint(0, 1) temp = str(temp) key = key + temp return key def exor_func(a, b): temp = "" for i in range(len(a)): if a[i] == b[i]: temp += "0" else: temp += "1" return temp def convert_bin_to_dec(binary): string = int(binary, 2) return string plaintext = "Hello Everyone" print("Plain Text is:", plaintext) plaintext_Ascii = [ord(x) for x in plaintext] plaintext_Bin = [format(y, '08b') for y in plaintext_Ascii] plaintext_Bin = "".join(plaintext_Bin) n = len(plaintext_Bin) // 2 L1 = plaintext_Bin[0:n] R1 = plaintext_Bin[n::] m = len(R1) K1 = random_key(m) K2 = random_key(m) f1 = exor_func(R1, K1) R2 = exor_func(f1, L1) L2 = R1 f2 = exor_func(R2, K2) R3 = exor_func(f2, L2) L3 = R2 bin_data = L3 + R3 str_data = '' for i in range(0, len(bin_data), 7): temp_data = bin_data[i:i + 7] decimal_data = convert_bin_to_dec(temp_data) str_data = str_data + chr(decimal_data) print("Cipher Text:", str_data) L4 = L3 R4 = R3 f3 = exor_func(L4, K2) L5 = exor_func(R4, f3) R5 = L4 f4 = exor_func(L5, K1) L6 = exor_func(R5, f4) R6 = L5 plaintext1 = L6 + R6 plaintext1 = int(plaintext1, 2) Rplaintext = binascii.unhexlify('%x' % plaintext1) print("Decrypted Plain Text is: ", Rplaintext)
Output
Plain Text is: Hello Everyone Cipher Text: '$Mau*ALLd;7B Decrypted Plain Text is: b'Hello Everyone'
Summary
Organisations can use the popular encryption design concept known as the Feistel cipher to help secure their sensitive data. A robust encryption cipher should keep a hacker from deciphering the cipher plain text without the key or sets of keys, even if they are aware of the cipher algorithm. Businesses should implement a layered cybersecurity approach in addition to this cipher model to help stop attackers from stealing or disclosing their confidential data.
To Continue Learning Please Login