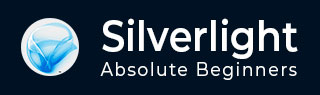
- Silverlight Tutorial
- Silverlight - Home
- Silverlight - Overview
- Silverlight - Environment Setup
- Silverlight - Getting Started
- Silverlight - XAML Overview
- Silverlight - Project Types
- Silverlight - Fixed Layouts
- Silverlight - Dynamic Layout
- Constrained vs. Unconstrained
- Silverlight - CSS
- Silverlight - Controls
- Silverlight - Buttons
- Silverlight - Content Model
- Silverlight - ListBox
- Silverlight - Templates
- Silverlight - Visual State
- Silverlight - Data Binding
- Silverlight - Browser Integration
- Silverlight - Out-of-Browser
- Silverlight - Applications, Resources
- Silverlight - File Access
- Silverlight - View Model
- Silverlight - Input Handling
- Silverlight - Isolated Storage
- Silverlight - Text
- Silverlight - Animation
- Silverlight - Video and Audio
- Silverlight - Printing
- Silverlight Useful Resources
- Silverlight - Quick Guide
- Silverlight - Useful Resources
- Silverlight - Discussion
Silverlight - Video & Audio
In this chapter, we will see how Silverlight facilities are playing video and audio. The MediaElement is the heart of all video and audio in Silverlight. This allows you to integrate audio and video in your application. The MediaElement class works in a similar way like as Image class. You just point it at the media and it renders audio and video.
The main difference is it will be a moving image, but if you point it to the file that contains just audio and no video such as an MP3, it will play that without showing anything on the screen.
MediaElement as UI Element
MediaElement derives from framework element, which is the base class of all Silverlight user interface elements. This means it offers all the standard properties, so you can modify its opacity, you can set the clip, or transform it and so.
Let us have a look at a simple example of MediaElement.
Open Microsoft Blend for Visual Studio and create a new Silverlight Application project.
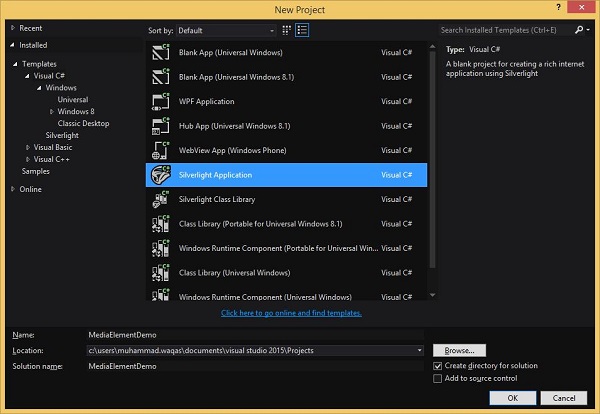
Now drag and video or audio file into Blend design surface.
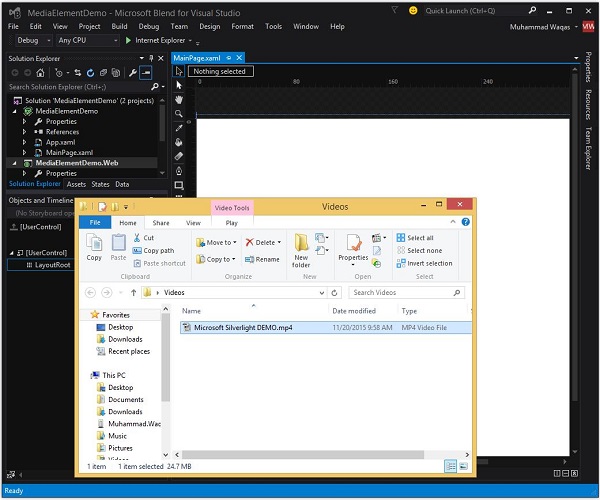
It will add a MediaElement to the surface and also add a copy of the video file in your project. You can see it in Solution explorer.
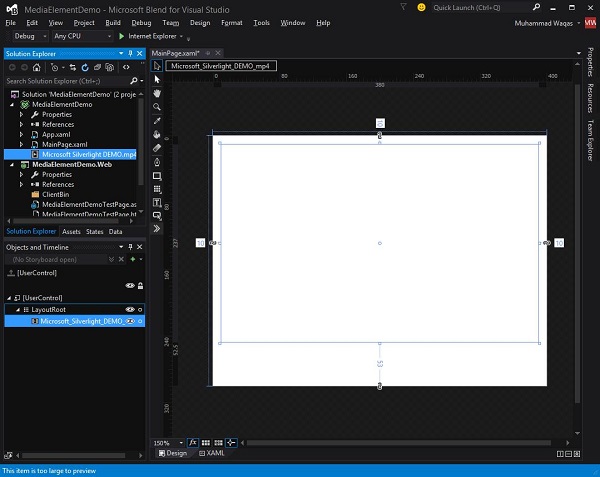
You can move it around, change its size, you can do things like applying a rotation etc.
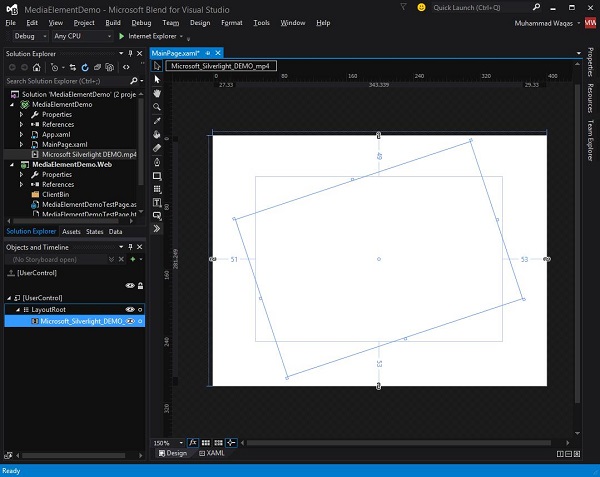
Now, it will generate the related XAML for you in MainPage.xaml file like shown below.
<UserControl x:Class = "MediaElementDemo.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d" d:DesignHeight = "300" d:DesignWidth = "400"> <Grid x:Name = "LayoutRoot" Background = "White"> <MediaElement x:Name = "Microsoft_Silverlight_DEMO_mp4" Margin = "51,49,53,53" Source = "/Microsoft Silverlight DEMO.mp4" Stretch = "Fill" RenderTransformOrigin = "0.5,0.5"> <MediaElement.RenderTransform> <CompositeTransform Rotation = "-18.384"/> </MediaElement.RenderTransform> </MediaElement> </Grid> </UserControl>
When the above application is compiled and executed, you will see that the video is playing on your web page.
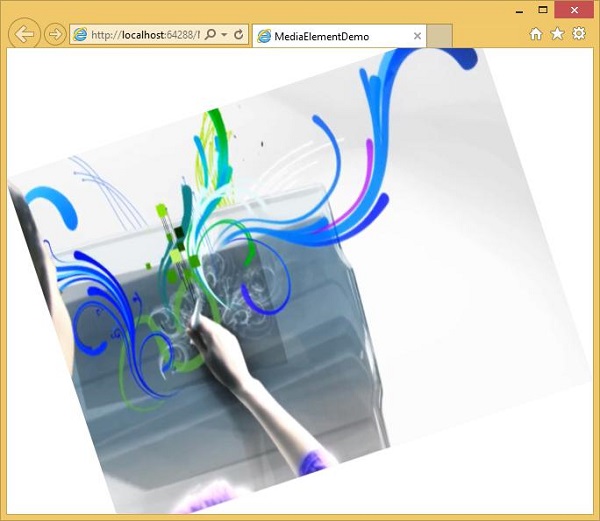
Controlling
The MediaElement just presents the media. It does not offer any standard player controls. It starts playing automatically and stops when it reaches the end, and there is nothing a user can do to pause or otherwise control it. So in practice most applications will want to provide the user with a bit more control than that.
You can disable the automatic playback by setting AutoPlay to False. This means the media player will not play anything until you ask it.
<MediaElement x:Name = "Microsoft_Silverlight_DEMO_mp4" AutoPlay = "False" Margin = "51,49,53,53" Source = "/Microsoft Silverlight DEMO.mp4" Stretch = "Fill" RenderTransformOrigin = "0.5,0.5">
So when you want to play the video, you can just call the MediaElement Play() method. It also offers stop and pause methods.
Let us have a look at the same example again and modify it little bit to allow a bit of control. Attach the MouseLeftButtonDown handler in MediaElement as shown in the XAML code below.
<UserControl x:Class = "MediaElementDemo.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d" d:DesignHeight = "300" d:DesignWidth = "400"> <Grid x:Name = "LayoutRoot" Background = "White"> <MediaElement x:Name = "Microsoft_Silverlight_DEMO_mp4" AutoPlay = "False" MouseLeftButtonDown = "Microsoft_Silverlight_DEMO_mp4_MouseLeftButtonDown" Margin = "51,49,53,53" Source = "/Microsoft Silverlight DEMO.mp4" Stretch = "Fill" RenderTransformOrigin = "0.5,0.5"> </MediaElement> </Grid> </UserControl>
Here is the implementation on the MouseLeftButtonDown event handler in which it will check that if the current state of the media element is plating then it will pause the video otherwise it will start playing the video.
using System.Windows.Controls; using System.Windows.Input; using System.Windows.Media; namespace MediaElementDemo { public partial class MainPage : UserControl { public MainPage() { InitializeComponent(); } private void Microsoft_Silverlight_DEMO_mp4_MouseLeftButtonDown (object sender, MouseButtonEventArgs e) { if (Microsoft_Silverlight_DEMO_mp4.CurrentState == MediaElementState.Playing) { Microsoft_Silverlight_DEMO_mp4.Pause(); } else { Microsoft_Silverlight_DEMO_mp4.Play(); } } } }
When the above code is compiled and executed, you will see the blank web page because we have set the AutoPlay property to False. When you click the web page, it will start the video.
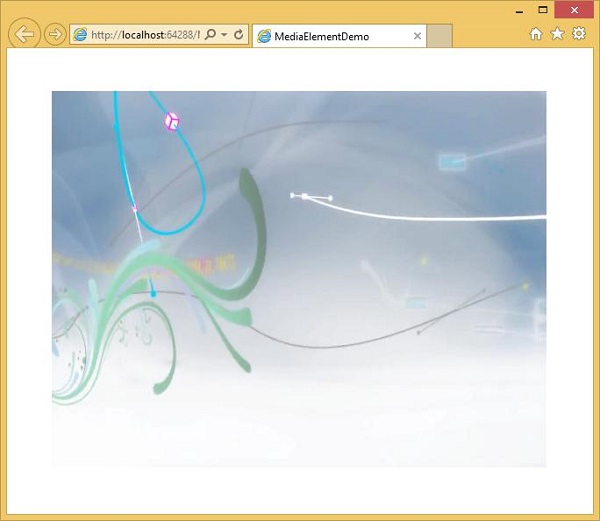
When you click the web page again, it will pause the video.