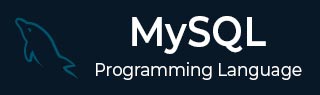
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - PERIOD_ADD() Function
The MySQL PERIOD_ADD() function is used to add a certain number of months to a period. In MySQL, a period is defined as a combination of year and month; and it will be represented in the format, YYMM or YYYYMM.
This MySQL function accepts a period and a numerical value representing the number of months as its arguments; and adds the given number of months to the given period. The result of this function will be in the form of YYYYMM.
Syntax
Following is the syntax of MySQL PERIOD_ADD() function −
PERIOD_ADD(P,N);
Parameters
This method accepts two parameters. The same is described below −
P is the value period value. The format is "YYMM" or "YYYYMM"
N is the number of months you need to add to the period.
Return value
This function returns a new period value after adding the specified number of months.
Example
Following example demonstrates the usage of the PERIOD_ADD() function −
SELECT PERIOD_ADD('202309', 12) As Result;
Output
This will produce the following result −
Result |
---|
202409 |
Example
We can also pass negative values as arguments to this function −
SELECT PERIOD_ADD('202308', -09) As Result;
Output
Following is the output −
Result |
---|
202211 |
Example
In this example, we have created a table named PLAYERS using the following CREATE TABLE query
CREATE TABLE PLAYERS( ID int, NAME varchar(255), DATE_OF_BIRTH date, DOB_PERIOD varchar(30), Country varchar(255), PRIMARY KEY (ID) );
Now, insert the following records into the ORDERS table using the INSERT statement −
INSERT INTO PLAYERS VALUES (1, 'Shikhar Dhawan', DATE('1981-12-05'), 198112, 'India'), (2, 'Jonathan Trott', DATE('1981-04-22'), 198104, 'SouthAfrica'), (3, 'Kumara Sangakkara', DATE('1977-10-27'), 197710, 'Srilanka'), (4, 'Virat Kohli', DATE('1988-11-05'), 198811, 'India'), (5, 'Rohit Sharma', DATE('1987-04-30'), 198704, 'India'), (6, 'Ravindra Jadeja', DATE('1988-12-06'), 198812, 'India'), (7, 'James Anderson', DATE('1982-06-30'), 198206, 'England');
Execute the below query to fetch all the inserted records in the above-created table −
Select * From PLAYERS;
Following is the PLAYERS table −
ID | NAME | DATE_OF_BIRTH | DOB_PERIOD | COUNTRY |
---|---|---|---|---|
1 | Shikhar Dhawan | 1981-12-05 | 198112 | India |
2 | Jonathan Trott | 1981-04-22 | 198104 | SouthAfrica |
3 | Kumara Sangakkara | 1977-10-27 | 197710 | Srilanka |
4 | Virat Kohli | 1988-11-05 | 198811 | India |
5 | Rohit Sharma | 1987-04-30 | 198704 | India |
6 | Ravindra Jadeja | 1988-12-06 | 198812 | India |
7 | James Anderson | 1982-06-30 | 198206 | England |
Here, we are using the MySQL PERIOD_ADD() function to add 12 months to "DOB_PERIOD" from the "PLAYERS" table −
SELECT ID, NAME, DATE_OF_BIRTH, DOB_PERIOD, PERIOD_ADD(DOB_PERIOD, 12) As Result From PLAYERS;
Output
The output is displayed as follows −
ID | NAME | DATE_OF_BIRTH | DOB_PERIOD | Result |
---|---|---|---|---|
1 | Shikhar Dhawan | 1981-12-05 | 198112 | 198212 |
2 | Jonathan Trott | 1981-04-22 | 198104 | 198204 |
3 | Kumara Sangakkara | 1977-10-27 | 197710 | 197810 |
4 | Virat Kohli | 1988-11-05 | 198811 | 198911 |
5 | Rohit Sharma | 1987-04-30 | 198704 | 198804 |
6 | Ravindra Jadeja | 1988-12-06 | 198812 | 198912 |
7 | James Anderson | 1982-06-30 | 198206 | 198306 |