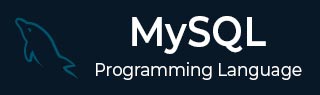
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Upsert
The MySQL UPSERT Operation
The MySQL UPSERT operation combines INSERT and UPDATE into a single statement, allowing you to insert a new row into a table or update an existing row if it already exists. We can understand in the name (UPSERT) itself, where UP stands for UPDATE and SERT stands for INSERT.
This tutorial covers three common methods to perform UPSERT operations in MySQL: INSERT IGNORE, REPLACE, and INSERT with ON DUPLICATE KEY UPDATE.
UPSERT Using INSERT IGNORE
The INSERT IGNORE statement in MySQL allows you to insert a new record into a table. If a record with the same primary key already exists, it ignores the error and doesn't insert the new record.
Example
First, let us create a table with the name COURSES using the following query −
CREATE TABLE COURSES( ID int, COURSE varchar(50) primary key, COST int );
Here, we are inserting records into the COURSES table −
INSERT INTO COURSES VALUES (1, "HTML", 3000), (2, "CSS", 4000), (3, "JavaScript", 6000), (4, "Node.js", 10000), (5, "React.js", 12000), (6, "Angular", 8000), (7, "Php", 9000);
The COURSES table obtained is as follows −
ID | COURSE | COST |
---|---|---|
6 | Angular | 8000 |
2 | CSS | 4000 |
1 | HTML | 3000 |
3 | JavaScript | 6000 |
4 | Node.js | 10000 |
7 | Php | 9000 |
5 | React.js | 12000 |
Now, we attempt to insert a duplicate record using the INSERT INTO statement in the following query −
INSERT INTO COURSES VALUES (6, 'Angular', 9000);
This results in an error because a duplicate record cannot be inserted −
ERROR 1062 (23000): Duplicate entry 'Angular' for key 'courses.PRIMARY'
Using INSERT IGNORE −
Now, let us perform the same operation using INSERT IGNORE statement −
INSERT IGNORE INTO COURSES VALUES (6, 'Angular', 9000);
Output
As we can see in the output below, the INSERT IGNORE statement ignores the error −
Query OK, 0 rows affected, 1 warning (0.00 sec)
Verification
We can verify the COURSES table to see that the error was ignored using the following SELECT query −
SELECT * FROM COURSES;
The table obtained is as follows −
ID | COURSE | COST |
---|---|---|
6 | Angular | 8000 |
2 | CSS | 4000 |
1 | HTML | 3000 |
3 | JavaScript | 6000 |
4 | Node.js | 10000 |
7 | Php | 9000 |
5 | React.js | 12000 |
UPSERT Using REPLACE
The MySQL REPLACE statement first attempts to delete the existing row if it exists and then inserts the new row with the same primary key. If the row does not exist, it simply inserts the new row.
Example
Let us replace or update a row in the COURSES table. If a row with COURSE "Angular" already exists, it will update its values for ID and COST with the new values provided. Else, a new row will be inserted with the specified values in the query −
REPLACE INTO COURSES VALUES (6, 'Angular', 9000);
Output
The output for the query above produced is as given below −
Query OK, 2 rows affected (0.01 sec)
Verification
Now, let us verify the COURSES table using the following SELECT query −
SELECT * FROM COURSES;
We can see in the following table, the REPLACE statement added a new row after deleting the duplicate row −
ID | COURSE | COST |
---|---|---|
6 | Angular | 9000 |
2 | CSS | 4000 |
1 | HTML | 3000 |
3 | JavaScript | 6000 |
4 | Node.js | 10000 |
7 | Php | 9000 |
5 | React.js | 12000 |
UPSERT Using INSERT with ON DUPLICATE KEY UPDATE
The INSERT ... ON DUPLICATE KEY UPDATE statement in MySQL attempts to insert a new row. If the row already exists, it updates the existing row with the new values specified in the statement.
Example
Here, we are updating the duplicate record using the following query −
INSERT INTO COURSES VALUES (6, 'Angular', 9000) ON DUPLICATE KEY UPDATE ID = 6, COURSE = 'Angular', COST = 20000;
Output
As we can see in the output below, no error is generated and the duplicate row gets updated.
Query OK, 2 rows affected (0.01 sec)
Verification
Let us verify the COURSES table using the following SELECT query −
SELECT * FROM COURSES;
As we can see the table below, the INSERT INTO... ON DUPLICATE KEY UPDATE statement updated the duplicate record −
ID | COURSE | COST |
---|---|---|
6 | Angular | 20000 |
2 | CSS | 4000 |
1 | HTML | 3000 |
3 | JavaScript | 6000 |
4 | Node.js | 10000 |
7 | Php | 9000 |
5 | React.js | 12000 |