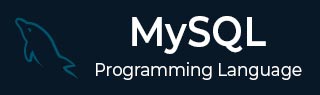
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Unlock User Account
Account Locking and Unlocking in MySQL is introduced to increase security of the database by preventing unauthorized transactions or suspicious activities.
MySQL Unlock User Account
To check whether an account is unlocked or not, MySQL provides the 'account_locked' attribute in the 'mysql.user' table that will hold either 'Y' or 'N' values respectively. If the attribute holds the 'N' value, then the account is said to be in the unlock mode.
By default, all the new user accounts created in MySQL are unlocked.
Unlocking New Accounts
You can use the CREATE USER... ACCOUNT UNLOCK statement to unlock new accounts created in MySQL. By default, the newly created accounts are always unlocked unless specified otherwise. However, the ACCOUNT UNLOCK clause is mostly used when an account is in the locked state.
Syntax
Following is the syntax of CREATE USER... ACCOUNT UNLOCK statement −
CREATE USER username@hostname IDENTIFIED BY 'new_password' ACCOUNT UNLOCK;
Example
In the following query, we are creating a new user account in MySQL using the CREATE USER statement −
CREATE USER testuser@localhost IDENTIFIED BY 'qwerty' ACCOUNT UNLOCK;
Output
Following is the output of the above code −
Query OK, 0 rows affected (0.02 sec)
Verification
We can verify whether the account of the 'testuser' is unlocked or not using the following SELECT statement −
SELECT User, Host, account_locked FROM mysql.user WHERE User = 'testuser';
Output of the above code is as shown below −
User | Host | account_locked |
---|---|---|
testuser | localhost | N |
Example
As we have learned above, the newly created user accounts are unlocked by default. Look at the example below −
CREATE USER demo@localhost IDENTIFIED BY '000000';
Output
The result produced is as follows −
Query OK, 0 rows affected (0.02 sec)
Verification
We can verify whether the newly created account is unlocked by default using the following SELECT statement −
SELECT User, Host, account_locked FROM mysql.user WHERE User = 'demo';
The output obtained is as follows −
User | Host | account_locked |
---|---|---|
demo | localhost | N |
Unlocking Existing Accounts
We can use the ALTER USER... ACCOUNT UNLOCK statement unlock existing accounts in MySQL that are locked beforehand.
Syntax
Following is the syntax of ALTER USER... ACCOUNT UNLOCK statement −
ALTER USER username@hostname ACCOUNT UNLOCK;
Example
We are first retrieving the information of the existing account 'sample', including its username, host, and the status of its account lock −
SELECT User, Host, account_locked FROM mysql.user WHERE User = 'sample';
We can see in the output below that the user account is locked −
User | Host | account_locked |
---|---|---|
test | localhost | Y |
Now, we will unlock the existing account 'sample' using the ALTER USER statement −
ALTER USER sample@localhost ACCOUNT UNLOCK;
Output
Following is the output of the above query −
Query OK, 0 rows affected (0.00 sec)
Verification
We can verify whether the account is unlocked or not using the following SELECT query −
SELECT User, Host, account_locked FROM mysql.user WHERE User = 'sample';
As we can see in the below output, the 'sample@localhost' account is now unlocked and can be accessed according to its privileges −
User | Host | account_locked |
---|---|---|
sample | localhost | N |
Unlock User Account Using a Client Program
Now, in this section let us discuss how to unlock a MySQL user using various client programs.
Syntax
Following are the syntaxes −
Following is the syntax to unlock a MySQL user account using PHP −
$sql = "ALTER USER user_name ACCOUNT UNLOCK"; $mysqli->query($sql);
Following is the syntax to unlock a MySQL user account using JavaScript −
sql= "CREATE USER username@hostname IDENTIFIED BY 'new_password' ACCOUNT UNLOCK"; con.query(sql, function (err, result) { if (err) throw err; console.log(result); });
Following is the syntax to unlock a MySQL user account using Java −
String sql = "ALTER USER USER_NAME@LOCALHOST ACCOUNT UNLOCK"; statement.execute(sql);
Following is the syntax to unlock a MySQL user account using Python −
sql = f"ALTER USER '{username_to_unlock}'@'localhost' ACCOUNT UNLOCK"; cursorObj.execute(sql);
Example
Following are the programs to unlock users in various programming languages −
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass); if($mysqli->connect_errno ) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } //printf('Connected successfully.
'); $sql = "ALTER USER Sarika ACCOUNT UNLOCK"; if($mysqli->query($sql)){ printf("User has been unlocked successfully..!"); } if($mysqli->error){ printf("Failed..!" , $mysqli->error); } $mysqli->close();
Output
The output obtained is as follows −
User has been unlocked successfully..!
var mysql = require('mysql2'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "Nr5a0204@123" }); //Connecting to MySQL con.connect(function (err) { if (err) throw err; console.log("Connected!"); console.log("--------------------------"); sql = "CREATE USER testuser@localhost IDENTIFIED BY 'qwerty' ACCOUNT UNLOCK;" con.query(sql); sql = "SELECT User, Host, account_locked FROM mysql.user WHERE User = 'testuser';"; con.query(sql, function(err, result){ if (err) throw err; console.log(result); }); });
Output
The output produced is as follows −
Connected! -------------------------- [ { User: 'testuser', Host: 'localhost', account_locked: 'N' } ]
import java.sql.Connection; import java.sql.DriverManager; import java.sql.Statement; public class UnlockUserAccount { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/TUTORIALS"; String user = "root"; String password = "password"; try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection(url, user, password); Statement st = con.createStatement(); //System.out.println("Database connected successfully...!"); String sql = "ALTER USER Vivek@localhost ACCOUNT UNLOCK"; st.execute(sql); System.out.println("User 'Vivek' account unlocked successfully...!"); }catch(Exception e) { e.printStackTrace(); } } }
Output
The output obtained is as shown below −
User 'Vivek' account unlocked successfully...!
import mysql.connector # creating the connection object connection = mysql.connector.connect( host='localhost', user='root', password='password' ) username_to_unlock = 'newUser' # Create a cursor object for the connection cursorObj = connection.cursor() cursorObj.execute(f"ALTER USER '{username_to_unlock}'@'localhost' ACCOUNT UNLOCK") print(f"User '{username_to_unlock}' account is unlocked successfully.") cursorObj.close() connection.close()
Output
Following is the output of the above code −
User 'newUser' account is unlocked successfully.