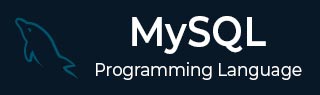
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Change Password
MySQL provides an account to each user which is authenticated with a username and a password. The default account in MySQL is a root with no password (One can however set a password to the root using a statement). Similarly, other user-defined accounts can have passwords set explicitly using an SQL statement or can have it system generated by MySQL.
MySQL Change User Password
Just like any other authenticated accounts, MySQL has a provision to change the user password. But one must make sure that there is currently no application being used by the user. If the password is reset without disconnecting the application, the application cannot connect to the server through this user again.
We can change the password for a MySQL user account using the following three SQL statements −
UPDATE statement
SET PASSWORD statement
ALTER USER statement
The UPDATE Statement
The most basic way to change a user's password in MySQL is by using the UPDATE statement. This statement is used to update account details, including the account password, from the 'root' account. But, once the modifications are done using this statement, you must use the FLUSH PRIVILEGES statement to reload privileges from the grant table of the MySQL database.
Syntax
Following is the syntax to change password using the UPDATE statement −
UPDATE mysql.user SET authentication_string = PASSWORD(password_string) WHERE User = user_name AND Host = host_name FLUSH PRIVILEGES;
Example
Following example demonstrates how to change the password of a user account using the UPDATE statement. Firstly, we are creating a user account "sample" with a password '123456' −
CREATE USER 'sample'@'localhost' IDENTIFIED BY '123456';
Following is the output obtained −
Query OK, 0 rows affected (0.02 sec)
Now, you can verify the list of users using the following query −
SELECT User FROM mysql.user;
The table will be displayed as shown below −
User |
---|
mysql.infoschema |
mysql.session |
mysql.sys |
root |
sample |
If you have the MySQL version 5.7.6 and later, you can directly modify the mysql.user table with the following query −
UPDATE user SET authentication_string = PASSWORD('xxxxxx') WHERE User = 'sample' AND Host = 'localhost';
After executing the above code, we get the following output −
Query OK, 1 row affected (0.02 sec) Rows matched: 1 Changed: 1 Warnings: 0
After making changes to user accounts, you need to use the FLUSH PRIVILEGES statement to apply these changes immediately −
FLUSH PRIVILEGES;
The output obtained is as shown below −
Query OK, 0 rows affected (0.01 sec)
The SET PASSWORD statement
The SET PASSWORD statement is used to set a password for a MySQL account. It contains a "password-verification" clause which lets the system know that the current user password needs to be replaced by another.
Syntax
Following is the syntax for the SET PASSWORD statement −
SET PASSWORD FOR username@localhost = password_string;
You can also change the password using SET PASSWORD without using the FOR clause. To use this syntax however, you must already be logged in on the user account you wish to change the password of −
SET PASSWORD = password_string;
Example
Now, using the SET PASSWORD statement, we are changing the password to 'hello' −
SET PASSWORD = 'hello';
Output
Following is the output of the above code −
Query OK, 0 rows affected (0.01 sec)
The ALTER USER Statement
To alter anything regarding a user account in MySQL, including changing passwords, ALTER USER statement is more preferable than SET PASSWORD statement. This statement is not used alone, instead is followed by the IDENTIFIED BY clause to authenticate the new password.
Note that the user must be connected to the MySQL server for this statement to work.
Syntax
Following is the syntax to change the password using the ALTER USER statement −
ALTER USER username IDENTIFIED BY 'password';
Example
Here, we are changing the password of the sample@localhost account to '000000' using the ALTER USER query given below −
ALTER USER sample@localhost IDENTIFIED BY '000000';
Output
Output of the above code is shown below −
Query OK, 0 rows affected (0.01 sec)
The password is now changed. To verify, log in to the sample account again using the new password −
C:\Windows\System32> mysql -u sample -p Enter password: ****** mysql>
Changing User password Using a Client Program
Besides using MySQL queries to change the user password in MySQL, we can also use client programs like Node.js, PHP, Java, and Python to achieve the same result.
Syntax
Following are the syntaxes −
To change the user's password MySQL database, we need to execute the ALTER USER statement using this function as −
$sql = "ALTER USER 'root'@'localhost' IDENTIFIED BY 'new_password'"; $mysqli->query($sql);
To change the user's password MySQL, we need to execute the ALTER USER statement using the function named query() as −
sql= "ALTER USER username IDENTIFIED BY 'new_password'"; con.query(sql, function (err, result) { if (err) throw err; console.log(result); });
To change the user's password into MySQL database, we need to execute the ALTER USER statement using the JDBC execute() function as −
String sql = "ALTER USER 'USER_NAME'@'LOCALHOST' IDENTIFIED BY 'NEW_PASSWORD'"; statement.execute(sql);
The MySQL Connector/Python provides a function named execute() to execute an SQL query in the MySQL database. To change the user's password MySQL database, we need to execute the ALTER USER statement using this function as −
sql = f"ALTER USER '{username_to_change}'@'localhost' IDENTIFIED BY '{new_password}'"; cursorObj.execute(sql);
Example
Following are the client programs to change the user password in MySQL −
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass); if($mysqli->connect_errno ) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } //printf('Connected successfully.
'); $sql = "ALTER USER 'root'@'localhost' IDENTIFIED BY 'password1'"; if($mysqli->query($sql)){ printf("User password has been changed successfully...!"); } if($mysqli->error){ printf("Failed..!" , $mysqli->error); } $mysqli->close();
Output
The output obtained is as follows −
Your password has been changed successfully...!
var mysql = require('mysql2'); var con = mysql.createConnection({ host: "localhost", user: "root", password: "Nr5a0204@123"}); //Connecting to MySQL con.connect(function (err) { if (err) throw err; console.log("Connected!"); console.log("--------------------------"); //Listing the users sql = "SELECT USER FROM mysql.user;" con.query(sql, function(err, result){ if (err) throw err console.log("**List of Users:**") console.log(result) console.log("--------------------------"); }); sql = "ALTER USER 'sample'@'localhost' IDENTIFIED BY 'tutorials';"; con.query(sql, function(err){ if (err) throw err; console.log("Password changed Successfully..."); }); sql = "FLUSH PRIVILEGES;" con.query(sql); });
Output
The output produced is as follows −
Connected! -------------------------- **List of Users:** [ { USER: 'mysql.infoschema' }, { USER: 'mysql.session' }, { USER: 'mysql.sys' }, { USER: 'root' }, { USER: 'sample' } ] -------------------------- Password changed Successfully...
import java.sql.Connection; import java.sql.DriverManager; import java.sql.Statement; public class ChangePassword { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/TUTORIALS"; String user = "root"; String password = "password"; try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection con = DriverManager.getConnection(url, user, password); Statement st = con.createStatement(); //System.out.println("Database connected successfully...!"); String sql = "ALTER USER 'root'@'localhost' IDENTIFIED BY 'password1'"; st.execute(sql); System.out.println("User 'root' password changed successfully...!"); }catch(Exception e) { e.printStackTrace(); } } }
Output
The output obtained is as shown below −
User 'root' password changed successfully...!
import mysql.connector # creating the connection object connection = mysql.connector.connect( host='localhost', user='root', password='password' ) username_to_change = 'newUser' new_password = 'passwordSet!' # Create a cursor object for the connection cursorObj = connection.cursor() cursorObj.execute(f"ALTER USER '{username_to_change}'@'localhost' IDENTIFIED BY '{new_password}'") print(f"Password for user '{username_to_change}' changed successfully.") cursorObj.close() connection.close()
Output
Following is the output of the above code −
Password for user 'newUser' changed successfully.