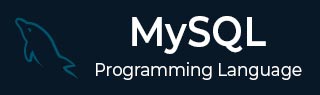
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Vertical Partitioning
The MySQL Partitioning is used to divide large tables into smaller partitions that are stored in different physical locations and are treated as separate tables. Even though the smaller partitions are managed individually, they are still part of the main table.
There are two forms of partitioning in MySQL: Horizontal Partitioning and Vertical Partitioning.
The MySQL Vertical Partitioning
The MySQL Vertical partitioning divides the table into multiple tables based on columns, rather than rows.
There are two main types of vertical partitioning in MySQL, each serving specific purposes −
- RANGE Columns Partitioning
- LIST Columns Partitioning
Both Range Columns Partitioning and List Columns Partitioning support various data types, including integer types (TINYINT, SMALLINT, MEDIUMINT, INT, and BIGINT), string types (CHAR, VARCHAR, BINARY, and VARBINARY), as well as DATE and DATETIME data types.
Range Columns Partitioning
The MySQL Range Columns partitioning uses one or more columns as partition keys to divide the data into partitions based on a defined range of column values.
The values in these columns are compared to predefined ranges, and each row is assigned to the partition that encompasses the range containing its column values.
Example
In the following query, we are creating a table named INVENTORY and dividing it into three partitions based on "product_quantity" and "product_price" columns. Rows with specific values in these columns are stored in their corresponding partitions −
CREATE TABLE INVENTORY ( id INT, product_name VARCHAR(50), product_quantity INT, product_price int ) PARTITION BY RANGE COLUMNS(product_quantity, product_price) ( PARTITION P_low_stock VALUES LESS THAN (10, 100), PARTITION P_medium_stock VALUES LESS THAN (50, 500), PARTITION P_high_stock VALUES LESS THAN (200, 1200) );
Here, we are inserting rows into the above-created table −
INSERT INTO INVENTORY VALUES (1, 'Headphones', 5, 50), (2, 'Mouse', 15, 200), (3, 'Monitor', 30, 300), (4, 'Keyboard', 60, 600), (5, 'CPU', 100, 1000);
Following is the INVENTORY table obtained −
id | product_name | product_quantity | product_price |
---|---|---|---|
1 | Headphones | 5 | 50 |
2 | Mouse | 15 | 200 |
3 | Monitor | 30 | 300 |
4 | Keyboard | 60 | 600 |
5 | CPU | 100 | 1000 |
Now that we have some data in the INVENTORY table, we can display the partition status to see how the data is distributed among the partitions using the following query −
SELECT PARTITION_NAME, TABLE_ROWS FROM INFORMATION_SCHEMA.PARTITIONS WHERE TABLE_NAME='inventory';
You will see in the output below that the respective columns are assigned to their respective partitions based on the defined range values −
PARTITION_NAME | TABLE_ROWS |
---|---|
P_high_stock | 2 |
P_low_stock | 1 |
P_medium_stock | 2 |
Displaying Partitions −
We can also display data from specific partitions using the PARTITION clause. For instance, to retrieve data from partition P_high_stock, we use the following query −
SELECT * FROM inventory PARTITION (P_high_stock);
It will display all the records in partition P_high_stock −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
4 | Keyboard | 60 | 600 | |
5 | CPU | 100 | 1000 |
Similarly, we can display other partitions using the same syntax.
List Columns Partitioning
The MySQL List columns partitioning uses one or more columns as partition keys and assigns records to partitions based on specific values in those columns. This method is handy when you want to group data into partitions based on discrete values or categories.
Example
Let us create a table named "EMPLOYEES" and partition it using LIST COLUMNS partitioning based on the "department" column −
CREATE TABLE EMPLOYEES ( id INT, first_name VARCHAR(50), last_name VARCHAR(50), hiring_date DATE, department VARCHAR(50) ) PARTITION BY LIST COLUMNS(department) ( PARTITION p_sales VALUES IN ('Sales', 'Marketing'), PARTITION p_engineering VALUES IN ('Engineering', 'Research'), PARTITION p_operations VALUES IN ('Operations') );
Here, we are inserting records into above-created table −
INSERT INTO EMPLOYEES VALUES (1, 'John', 'Doe', '2020-01-01', 'Sales'), (2, 'Jane', 'Doe', '2020-02-01', 'Marketing'), (3, 'Bob', 'Smith', '2020-03-01', 'Engineering'), (4, 'Alice', 'Johnson', '2020-04-01', 'Research'), (5, 'Mike', 'Brown', '2020-05-01', 'Operations');
Following is the EMPLOYEES table obtained −
id | first_name | last_name | hiring_date | department |
---|---|---|---|---|
1 | John | Doe | 2020-01-01 | Sales |
2 | Jane | Doe | 2020-02-01 | Marketing |
3 | Bob | Smith | 2020-03-01 | Engineering |
4 | Alice | Johnson | 2020-04-01 | Research |
5 | Mike | Brown | 2020-05-01 | Operations |
We can display the partition status of the EMPLOYEES table to see how the data is distributed among partitions using the following query −
SELECT PARTITION_NAME, TABLE_ROWS FROM INFORMATION_SCHEMA.PARTITIONS WHERE TABLE_NAME='EMPLOYEES';
It will display the partitions and the number of rows in each partition based on the department values −
PARTITION_NAME | TABLE_ROWS |
---|---|
p_engineering | 2 |
p_operations | 1 |
p_sales | 2 |