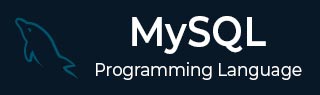
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - Cursor DECLARE Statement
A cursor in database is a construct which allows you to iterate/traversal the records of a table. In MySQL you can use cursors with in a stored program such as procedures, functions etc.
In other words, you can iterate though the records of a table from a MySQL stored program using the cursors. The cursors provided by MySQL are embedded cursors. They are −
READ ONLY − Using these cursors you cannot update any table.
Non-Scrollable − Using these cursors you can retrieve records from a table in one direction i.e., from top to bottom.
Asensitive − These cursors are insensitive to the changes that are made in the table i.e. the modifications done in the table are not reflected in the cursor.
Which means if we have created a cursor holding all the records in a table and, meanwhile if we add some more records to the table, these recent changes will not be reflected in the cursor we previously obtained.
While Declaring cursors in a stored program you need to make sure these (cursor declarations) always follow the variable and condition declarations.
To use a cursor, you need to follow the steps given below (in the same order)
- Declare the cursor using the DECLARE Statement.
- Declare variables and conditions.
- Open the declared cursor using the OPEN Statement.
- Retrieve the desired records from a table using the FETCH Statement.
- Finally close the cursor using the CLOSEstatement.
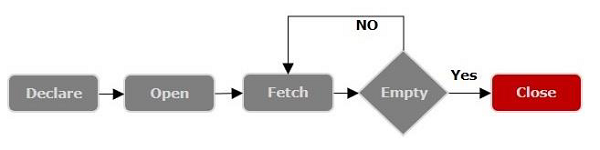
The DECLARE Statement
Using the DECLARE statement you can declare a cursor and associate It with the SELECT statement which fetches the desired records from a table. This SELECT statement associated with a cursor does not allow INTO clause.
Once you declare a cursor you can retrieve records from it using the FETCH statement. You need to make sure the cursor declaration precedes handler declarations. You can create use cursors in a single stored program.
Syntax
Following is the syntax of the MySQL Cursor DECLARE Statement −
DECLARE cursor_name CURSOR FOR select_statement;
Example
Assume we have created a table with name tutorials in MySQL database using CREATE statement as shown below −
CREATE TABLE tutorials ( ID INT PRIMARY KEY, TITLE VARCHAR(100), AUTHOR VARCHAR(40), DATE VARCHAR(40) );
Now, we will insert 5 records in tutorials table using INSERT statements −
Insert into tutorials values (1, 'Java', 'Krishna', '2019-09-01'), (2, 'JFreeCharts', 'Satish', '2019-05-01'), (3, 'JavaSprings', 'Amit', '2019-05-01'), (4, 'Android', 'Ram', '2019-03-01'), (5, 'Cassandra', 'Pruthvi', '2019-04-06');
Let us create another table to back up the data −
CREATE TABLE backup ( ID INT, TITLE VARCHAR(100), AUTHOR VARCHAR(40), DATE VARCHAR(40) );
Following procedure backups the contents of the tutorials table to the backup table using cursors −
DELIMITER // CREATE PROCEDURE ExampleProc() BEGIN DECLARE done INT DEFAULT 0; DECLARE tutorialID INTEGER; DECLARE tutorialTitle, tutorialAuthor, tutorialDate VARCHAR(20); DECLARE cur CURSOR FOR SELECT * FROM tutorials; DECLARE CONTINUE HANDLER FOR NOT FOUND SET done = 1; OPEN cur; label: LOOP FETCH cur INTO tutorialID, tutorialTitle, tutorialAuthor, tutorialDate; INSERT INTO backup VALUES(tutorialID, tutorialTitle, tutorialAuthor, tutorialDate); IF done = 1 THEN LEAVE label; END IF; END LOOP; CLOSE cur; END// DELIMITER ;
You can call the above procedure as shown below −
CALL ExampleProc;
If you verify the contents of the backup table you can see the inserted records as shown below −
select * from backup;
Output
The above query produces the following output −
ID | TITLE | AUTHOR | DATE |
---|---|---|---|
1 | Java | Krishna | 2019-09-01 |
2 | JFreeCharts | Satish | 2019-05-01 |
3 | JavaSprings | Amit | 2019-05-01 |
4 | Android | Ram | 2019-03-01 |
5 | Cassandra | Pruthvi | 2019-04-06 |