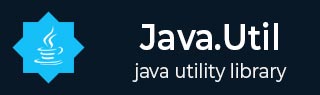
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Vector subList() Method
Description
The Java Vector subList() method returns a view of the portion of this list using the fromIndex(inclusive), and toIndex(exclusive). If fromIndex and toIndex are equal, it will return empty list. Any non-structural changes in the returned list are reflected in the original list as well, and vice-versa. The returned list supports list operations as well.
Declaration
Following is the declaration for java.util.Vector.subList() method
public List<E> subList(int fromIndex, int toIndex)
Parameters
fromIndex − The index of the start element (inclusive).
toIndex − The index of the end element (exclusive) .
Return Value
This method returns a view of the specified range within this list.
Exception
IndexOutOfBoundsException − if an endpoint index value is out of range (fromIndex < 0 || toIndex > size).
IllegalArgumentException − if the endpoint indices are out of order (fromIndex > toIndex).
Getting sublist from a Vector of Integer Example
The following example shows the usage of Java Vector subList() method. We're creating a Vector of Integers. We're adding couple of Integers to the Vector object using add() method calls per element and using subList() method, we're getting a portion of the list and printing that portion in the end.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<Integer> vector = new Vector<>(); // use add() method to add elements in the vector vector.add(0); vector.add(1); vector.add(2); vector.add(3); vector.add(4); vector.add(5); vector.add(6); // get and print a sublist System.out.println(vector.subList(1,3)); } }
Output
Let us compile and run the above program, this will produce the following result −
[1, 2]
Getting sublist from a Vector of String Example
The following example shows the usage of Java Vector subList() method. We're creating a Vector of Strings. We're adding couple of Strings to the Vector object using add() method calls per element and using subList() method, we're getting a portion of the list and printing that portion in the end.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<String> vector = new Vector<>(); // use add() method to add elements in the vector vector.add("A"); vector.add("B"); vector.add("C"); vector.add("D"); vector.add("E"); vector.add("F"); // get and print a sublist System.out.println(vector.subList(1,3)); } }
Output
Let us compile and run the above program, this will produce the following result −
[B, C]
Getting sublist from a Vector of Object Example
The following example shows the usage of Java Vector subList() method. We're creating a Vector of Student objects. We're adding couple of Student objects to the Vector object using add() method calls per element and using subList() method, we're getting a portion of the list and printing that portion in the end.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<Student> vector = new Vector<>(); // use add() method to add elements in the vector vector.add(new Student(1, "Julie")); vector.add(new Student(2, "Robert")); vector.add(new Student(3, "Adam")); // get and print a sublist System.out.println(vector.subList(1,3)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
Output
Let us compile and run the above program, this will produce the following result −
[[ 2, Robert ], [ 3, Adam ]]