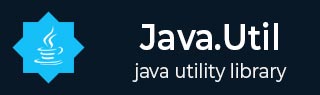
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Vector insertElementAt() Method
Description
The Java Vector insertElementAt(E obj,int index) method is used to insert the specified object as a component in this vector at the specified index. Each component in this vector with an index greater or equal to the specified index is shifted upward to have an index one greater than the value it had previously.
Declaration
Following is the declaration for java.util.Vector.insertElementAt() method
public void insertElementAt(E obj,int index)
Parameters
obj − This is the component to insert
index − This is the position where to insert the new component.
Return Value
NA
Exception
ArrayIndexOutOfBoundsException − This exception is thrown if the index is invalid.
Inserting Element at a Particular Index in a Vector of Integer Example
The following example shows the usage of Java Vector insertElementAt(Object element int index) method to insert the specified object as a component in this vector at the specified index. We're adding couple of Integer to the Vector object using add() method calls per element, printed the vector and using insertElementAt(Object element, index) method, we're inserting element at index of 1 and printing the updated vector.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Integer> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add(4); vec.add(3); vec.add(2); vec.add(1); // let us print all the elements available in vector System.out.println("Added numbers are: " + vec); // let us insert element at 1st position vec.insertElementAt(133, 1); System.out.println("Added numbers after insertion :" + vec); } }
Output
Let us compile and run the above program, this will produce the following result.
Added numbers are: [4, 3, 2, 1] Added numbers after insertion :[4, 133, 3, 2, 1]
Inserting Element at a Particular Index in a Vector of String Example
The following example shows the usage of Java Vector insertElementAt(Object element int index) method to insert the specified object as a component in this vector at the specified index. We're adding couple of String to the Vector object using add() method calls per element, printed the vector and using insertElementAt(Object element, index) method, we're inserting element at index of 1 and printing the updated vector.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<String> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add("D"); vec.add("C"); vec.add("B"); vec.add("A"); // let us print all the elements available in vector System.out.println("Added numbers are: " + vec); // let us insert element at 1st position vec.insertElementAt("F", 1); System.out.println("Added numbers after insertion :" + vec); } }
Output
Let us compile and run the above program, this will produce the following result.
Added numbers are: [D, C, B, A] Added numbers after insertion :[D, F, C, B, A]
Inserting Element at a Particular Index in a Vector of Object Example
The following example shows the usage of Java Vector insertElementAt(Object element int index) method to insert the specified object as a component in this vector at the specified index. We're adding couple of Student objects to the Vector object using add() method calls per element, printed the vector and using insertElementAt(Object element, index) method, we're inserting element at index of 1 and printing the updated vector.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty Vector vec with an initial capacity of 4 Vector<Student> vec = new Vector<>(4); // use add() method to add elements in the vector vec.add(new Student(1, "Julie")); vec.add(new Student(2, "Robert")); vec.add(new Student(3, "Adam")); // let us print all the elements available in vector System.out.println("Added numbers are: " + vec); // let us insert element at 1st position vec.insertElementAt(new Student(4, "Jane"), 1); System.out.println("Added numbers after insertion :" + vec); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Added numbers are: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Added numbers after insertion :[[ 1, Julie ], [ 4, Jane ], [ 2, Robert ], [ 3, Adam ]]