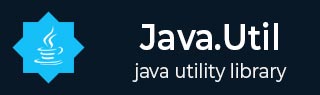
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Vector ensureCapacity() Method
Description
The ensureCapacity(int minCapacity) method is used to increases the capacity of this vector, if necessary.This is to ensure that the vector can hold at least the number of components specified by the minimum capacity argument.If the current capacity of this vector is less than minCapacity, then its capacity is increased by replacing its internal data array, kept in the field elementData, with a larger one. The size of the new data array will be the old size plus capacityIncrement.If the value of capacityIncrement is less than or equal to zero then the new capacity will be twice the old capacity.But if this new size is still smaller than minCapacity, then the new capacity will be minCapacity.
Declaration
Following is the declaration for java.util.Vector.ensureCapacity() method
public void ensureCapacity(int minCapacity)
Parameters
minCapacity − This is the desired minimum capacity.
Return Value
It returns void.
Exception
NA
Ensuring capacity of Vector of Integers Example
The following example shows the usage of Java Vector ensureCapacity(E) method to add Integers. We're adding couple of Integers to the Vector object using add() method calls per element and using ensureCapacity(), we're increasing its capacity to hold more items then print each element to show the elements added.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<Integer> vector = new Vector<>(); // use add() method to add elements in the vector vector.add(20); vector.add(30); vector.add(20); vector.add(30); vector.add(15); vector.add(22); vector.add(11); // this will increase the capacity of the Vector to 15 elements vector.ensureCapacity(15); // let us print all the elements available in vector System.out.println("Vector = " + vector); } }
Output
Let us compile and run the above program, this will produce the following result −
Vector = [20, 30, 20, 30, 15, 22, 11]
Ensuring capacity of Vector of Strings Example
The following example shows the usage of Java Vector ensureCapacity(E) method to add Strings. We're adding couple of Strings to the Vector object using add() method calls per element and using ensureCapacity(), we're increasing its capacity to hold more items then print each element to show the elements added.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<String> vector = new Vector<>(); // use add() method to add elements in the vector vector.add("Welcome"); vector.add("To"); vector.add("Tutorialspoint"); // this will increase the capacity of the Vector to 15 elements vector.ensureCapacity(15); System.out.println("Vector = " + vector); } }
Output
Let us compile and run the above program, this will produce the following result −
Vector = [Welcome, To, Tutorialspoint]
Ensuring capacity of Vector of Objects Example
The following example shows the usage of Java Vector ensureCapacity(E) method to add Student objects. We're adding couple of Student objects to the Vector object using add() method calls per element and using ensureCapacity(), we're increasing its capacity to hold more items then print each element to show the elements added.
package com.tutorialspoint; import java.util.Vector; public class VectorDemo { public static void main(String[] args) { // create an empty vector Vector<Student> vector = new Vector<>(); // use add() method to add elements in the vector vector.add(new Student(1, "Julie")); vector.add(new Student(2, "Robert")); vector.add(new Student(3, "Adam")); // this will increase the capacity of the Vector to 15 elements vector.ensureCapacity(15); System.out.println("Vector = " + vector); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
Vector = [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]]