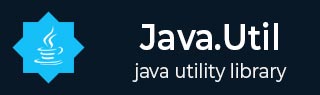
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java TreeMap putAll() Method
Description
The Java TreeMap putAll(Map<? extends K,? extends V> map) method is used to copy all of the mappings from the specified map to this map. These mappings replace any mappings that this map had for any of the keys currently in the specified map.
Declaration
Following is the declaration for java.util.TreeMap.putAll() method.
public void putAll(Map<? extends K,? extends V> map)
Parameters
map − This is the mappings to be stored in this map.
Return Value
NA
Exception
ClassCastException − This exception is thrown if the class of a key or value in the specified map prevents it from being stored in this map.
NullPointerException − This exception is thrown if the specified map is null or the specified map contains a null key and this map does not permit null keys.
Adding Multiple Key-Value Entries to a TreeMap of Integer,Integer Pair Example
The following example shows the usage of Java TreeMap putAll() method to add/update multiple key-value mappings in this map in single statement. We've created two TreeMap objects of Integer,Integer pairs. Then few entries are added using put() method to both maps, first map is printed then using putAll() method we're adding all entries of second map to first map and map is printed to validate the changes.
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree maps TreeMap<Integer, Integer> treemap = new TreeMap<>(); TreeMap<Integer, Integer> treemap_putall = new TreeMap<>(); // populating tree map treemap.put(2, 2); treemap.put(1, 1); treemap.put(3, 3); treemap.put(6, 6); treemap.put(5, 5); treemap_putall.put(1, 111); treemap_putall.put(2, 222); treemap_putall.put(7, 777); System.out.println("Value before modification: "+ treemap); // Putting 2nd map in 1st map treemap.putAll(treemap_putall); System.out.println("Value after modification: "+ treemap); } }
Output
Let us compile and run the above program, this will produce the following result.
Value before modification: {1=1, 2=2, 3=3, 5=5, 6=6} Value after modification: {1=111, 2=222, 3=3, 5=5, 6=6, 7=777}
Adding Multiple Key-Value Entries to a TreeMap of Integer,String Pair Example
The following example shows the usage of Java TreeMap putAll() method to add/update multiple key-value mappings in this map in single statement. We've created two TreeMap objects of Integer,String pairs. Then few entries are added using put() method to both maps, first map is printed then using putAll() method we're adding all entries of second map to first map and map is printed to validate the changes.
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree maps TreeMap<Integer, String> treemap = new TreeMap<>(); TreeMap<Integer, String> treemap_putall = new TreeMap<>(); // populating tree map treemap.put(2, "two"); treemap.put(1, "one"); treemap.put(3, "three"); treemap.put(6, "six"); treemap.put(5, "five"); treemap_putall.put(1, "111"); treemap_putall.put(2, "222"); treemap_putall.put(7, "777"); System.out.println("Value before modification: "+ treemap); // Putting 2nd map in 1st map treemap.putAll(treemap_putall); System.out.println("Value after modification: "+ treemap); } }
Output
Let us compile and run the above program, this will produce the following result.
Value before modification: {1=one, 2=two, 3=three, 5=five, 6=six} Value after modification: {1=111, 2=222, 3=three, 5=five, 6=six, 7=777}
Adding Multiple Key-Value Entries to a TreeMap of Integer,Object Pair Example
The following example shows the usage of Java TreeMap putAll() method to add/update multiple key-value mappings in this map in single statement. We've created two TreeMap objects of Integer,Student pairs. Then few entries are added using put() method to both maps, first map is printed then using putAll() method we're adding all entries of second map to first map and map is printed to validate the changes.
package com.tutorialspoint; import java.util.TreeMap; public class TreeMapDemo { public static void main(String[] args) { // creating tree maps TreeMap<Integer, Student> treemap = new TreeMap<>(); TreeMap<Integer, Student> treemap_putall = new TreeMap<>(); // populating tree map treemap.put(2, new Student(2, "Robert")); treemap.put(1, new Student(1, "Julie")); treemap.put(3, new Student(3, "Adam")); treemap.put(6, new Student(6, "Julia")); treemap.put(5, new Student(5, "Tom")); treemap_putall.put(1, new Student(111, "Julie")); treemap_putall.put(2, new Student(222, "Robert")); treemap_putall.put(7, new Student(777, "Jose")); System.out.println("Value before modification: "+ treemap); // Putting 2nd map in 1st map treemap.putAll(treemap_putall); System.out.println("Value after modification: "+ treemap); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
Output
Let us compile and run the above program, this will produce the following result.
Value before modification: {1=[ 1, Julie ], 2=[ 2, Robert ], 3=[ 3, Adam ], 5=[ 5, Tom ], 6=[ 6, Julia ]} Value after modification: {1=[ 111, Julie ], 2=[ 222, Robert ], 3=[ 3, Adam ], 5=[ 5, Tom ], 6=[ 6, Julia ], 7=[ 777, Jose ]}