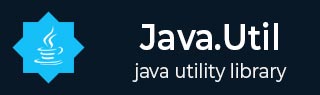
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Scanner remove() Method
Description
The Java Scanner remove() method is not supported by this implementation of Iterator.
Declaration
Following is the declaration for java.util.Scanner.remove() method
public void remove()
Parameters
NA
Return Value
This method does not return a value.
Exception
UnsupportedOperationException − if this method is invoked.
Checking Remove Method on Scanner on String Example
The following example shows the usage of Java Scanner remove() method showcasing that this method is not supported by this object. We've created a scanner object using a given string. Then we printed the string using nextLine() method and remove method is called which throws exception then scanner is closed using close() method.
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { String s = "Hello World! 3 + 3.0 = 6"; // create a new scanner with the specified String Object Scanner scanner = new Scanner(s); // print the next line of the string System.out.println(scanner.nextLine()); try { // invoking remove method will cause exception scanner.remove(); } catch(UnsupportedOperationException e){ System.out.println("Remove method is not supported."); } scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result −
Hello World! 3 + 3.0 = 6 Remove method is not supported.
Checking Remove Method on Scanner on User Input Example
The following example shows the usage of Java Scanner remove() method showcase that this method is not supported by this object. We've created a scanner object using a System.in. Then we printed the string using nextLine() method and remove method is called which throws exception then scanner is closed using close() method.
package com.tutorialspoint; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) { // create a new scanner with the system input Scanner scanner = new Scanner(System.in); // print the next line of the string System.out.println(scanner.nextLine()); try { // invoking remove method will cause exception scanner.remove(); } catch(UnsupportedOperationException e){ System.out.println("Remove method is not supported."); } scanner.close(); } }
Output
Let us compile and run the above program, this will produce the following result − (where we've entered Hello World and pressed enter key.)
Hello World Hello World Remove method is not supported.
Checking Remove Method on Scanner on Properties File Example
The following example shows the usage of Java Scanner remove() method showcase that this method is not supported by this object. We've created a scanner object using a File properties.txt. Then we printed the string using nextLine() method and remove method is called which throws exception then scanner is closed using close() method.
package com.tutorialspoint; import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class ScannerDemo { public static void main(String[] args) throws FileNotFoundException { // create a new scanner with a file as input Scanner scanner = new Scanner(new File("properties.txt")); // print the next line of the string System.out.println(scanner.nextLine()); try { // invoking remove method will cause exception scanner.remove(); } catch(UnsupportedOperationException e){ System.out.println("Remove method is not supported."); } scanner.close(); } }
Assuming we have a file properties.txt available in your CLASSPATH, with the following content. This file will be used as an input for our example program −
Hello World! 3 + 3.0 = 6
Output
Let us compile and run the above program, this will produce the following result −.
Hello World! 3 + 3.0 = 6 Remove method is not supported.