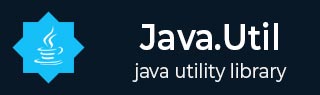
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java.util.LinkedList.addAll() Method
Description
The java.util.LinkedList.addAll(int index,Collection<? extends E> c) method inserts all of the elements in the specified collection into this list, starting at the specified position.
Declaration
Following is the declaration for java.util.LinkedList.addAll() method
public boolean addAll(int index,Collection<? extends E> c)
Parameters
index − index at which to insert the first element from the specified collection
c − collection containing elements to be added to this list
Return Value
This method returns true if this list changed as a result of the call
Exception
NullPointerException − if the specified collection is null
IndexOutOfBoundsException − if the index is out of range
Example
The following example shows the usage of java.util.LinkedList.addAll() method.
package com.tutorialspoint; import java.util.*; public class LinkedListDemo { public static void main(String[] args) { // create a LinkedList LinkedList list = new LinkedList(); // add some elements list.add("Hello"); list.add(2); list.add("Chocolate"); list.add("10"); // print the list System.out.println("LinkedList:" + list); // create a new collection and add some elements Collection collection = new ArrayList(); collection.add("One"); collection.add("Two"); collection.add("Three"); // add the collection in the LinkedList at index 2 list.addAll(2, collection); // print the new list System.out.println("LinkedList:" + list); } }
Let us compile and run the above program, this will produce the following result −
LinkedList:[Hello, 2, Chocolate, 10] LinkedList:[Hello, 2, One, Two, Three, Chocolate, 10]
java_util_linkedlist.htm
Advertisements