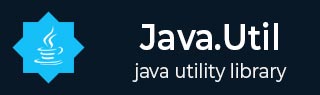
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java LinkedList addAll() Method
Description
The Java LinkedList addAll(Collection<? extends E> c) method inserts all the elements the specified collection c at the end of the linkedList. This method is equivalent to calling addLast(i) for each item of the collection c. Insertion order is maintained while adding the collection to the LinkedList and we can check the same by printing each element one by one.
Declaration
Following is the declaration for java.util.LinkedList.addAll(Collection<? extends E> c) method
public boolean addAll(Collection<? extends E> c)
Parameters
c − The collection of elements to be added in the linkedList.
Return Value
This method returns true if linkedList is changed as a result of this call, otherwise it returns false.
Exception
NullPointerException − if the specified collection or any of its element is null.
Java LinkedList addAll(index, collection) Method
Description
The Java LinkedList addAll(int index, Collection<? extends E> c) method inserts all the elements the specified collection c at a specified index of the linkedList. This operation shifts the element currently at that position if present and any subsequent elements to the right by increasing the indices. Insertion order is maintained while adding the collection to the LinkedList and we can check the same by printing each element one by one.
Declaration
Following is the declaration for java.util.LinkedList.addAll(int index, Collection<? extends E> c) method
public boolean addAll(int index, Collection<? extends E> c)
Parameters
index − index at which to insert the first element from the specified collection.
c − The collection of elements to be added in the linkedList.
Return Value
This method returns true if linkedList is changed as a result of this call, otherwise it returns false.
Exception
IndexOutOfBoundsException − if the index is out of range (index < 0 || index > size()).
NullPointerException − if the specified collection or any of its element is null.
Adding Multiple Elements to a LinkedList of Integer Example
The following example shows the usage of Java LinkedList addAll(collection) method to add a collection of Integers. We're adding couple of Integers to the LinkedList object using addAll(collection) method in single statement and then print each element to show the elements added.
package com.tutorialspoint; import java.util.LinkedList; import java.util.Arrays; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Integer> linkedList = new LinkedList<>(); // use addAll() method to add multiple elements in the linkedList linkedList.addAll(Arrays.asList(20,30,20,30,15,22,11)); System.out.println("LinkedList = " + linkedList); } }
Output
Let us compile and run the above program, this will produce the following result −
LinkedList = [20, 30, 20, 30, 15, 22, 11]
Adding Multiple Elements to a LinkedList of String Example
The following example shows the usage of Java LinkedList addAll(index, collection) method to add a collection of Strings. We're adding couple of strings to the LinkedList object using addAll(index, collection) method using single statement and then printing the LinkedList using its toString() method.
package com.tutorialspoint; import java.util.LinkedList; import java.util.Arrays; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<String> linkedList = new LinkedList<>(); // use addAll() method to add multiple elements in the linkedList linkedList.addAll(Arrays.asList("A","B","C","G")); // use addAll() method to add multiple elements in the linkedList at 4th position linkedList.addAll(3, Arrays.asList("D","E","F")); System.out.println("LinkedList = " + linkedList); } }
Output
Let us compile and run the above program, this will produce the following result −
LinkedList = [A, B, C, D, E, F, G]
Adding Multiple Elements to a LinkedList of Object Example
The following example shows the usage of Java LinkedList addAll(collection) method to add a collection of Student objects. We're adding couple of Student objects to the LinkedList object using addAll(collection) method in a single statement and then printing the LinkedList using its toString() method.
package com.tutorialspoint; import java.util.LinkedList; import java.util.List; import java.util.Arrays; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Student> linkedList = new LinkedList<Student>(); List<Student> students = Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam")); // use addAll() method to add multiple elements in the linkedList linkedList.addAll(students); System.out.println("LinkedList = " + linkedList); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
LinkedList = [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]]