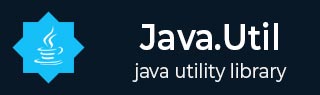
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java EnumSet clone() Method
Description
The Java EnumSet clone() method returns a copy of this set.
Declaration
Following is the declaration for java.util.EnumSet.clone() method
public EnumSet<E> clone()
Parameters
NA
Return Value
This method returns a copy of this set.
Exception
NA
Creating a Clone of EnumSet Example
The following example shows the usage of Java EnumSet clone() method to clone the EnumSet instance. We've created a enum Numbers. Then a EnumSet instance is created. Using allOf() method, enumSet is populated with values of enum and resulted enumSet is printed. Then using clone() method, its clone is created and printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Numbers { ONE, TWO, THREE, FOUR, FIVE }; public static void main(String[] args) { // create an EnumSet that has all elements from Numbers EnumSet<Numbers> set1 = EnumSet.allOf(Numbers.class); // print the set System.out.println("Set1:" + set1); // create a set2 which is a copy of set1 EnumSet<Numbers> set2 = set1.clone(); // print the updated set System.out.println("Set2:" + set2); } }
Output
Let us compile and run the above program, this will produce the following result −
Set1:[ONE, TWO, THREE, FOUR, FIVE] Set2:[ONE, TWO, THREE, FOUR, FIVE]
Creating a Clone of EnumSet of Enum with Values Example
The following example shows the usage of Java EnumSet clone() method to clone the EnumSet instance. We've created a enum Numbers with assigned values. Then a EnumSet instance is created. Using allOf() method, enumSet is populated with values of enum and resulted enumSet is printed. Then using clone() method, its clone is created and printed.
package com.tutorialspoint; import java.util.EnumSet; public class EnumSetDemo { // create an enum public enum Number { ONE("1"), TWO("2"), THREE("3"), FOUR("4"), FIVE("5"); // instance variable for enum private String value; public String getValue() { return this.value; } // constructor should be private or protected private Number(String value) { this.value = value; } @Override public String toString() { return this.name() +"(" + this.value + ")"; } }; public static void main(String[] args) { // create an EnumSet that has all elements from Number EnumSet<Number> set1 = EnumSet.allOf(Number.class); // print the set System.out.println("Set1:" + set1); // create a set2 which is a copy of set1 EnumSet<Number> set2 = set1.clone(); // print the updated set System.out.println("Set2:" + set2); } }
Output
Let us compile and run the above program, this will produce the following result −
Set1:[ONE(1), TWO(2), THREE(3), FOUR(4), FIVE(5)] Set2:[ONE(1), TWO(2), THREE(3), FOUR(4), FIVE(5)]