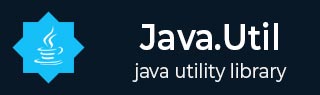
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
java.util.Collections.newSetFromMap() Method
Description
The newSetFromMap(Map<, Boolean>) method is used to return a set backed by the specified map.
Declaration
Following is the declaration for java.util.Collections.newSetFromMap() method.
public static <E> Set<E> newSetFromMap(Map<E, Boolean> map)
Parameters
map − The backing map
Return Value
The method call returns the set backed by the map.
Exception
IllegalArgumentException − This is thrown if map is not empty.
Example
The following example shows the usage of java.util.Collections.newSetFromMap()
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create map Map<String, Boolean> map = new WeakHashMap<String, Boolean>(); // create a set from map Set<String> set = Collections.newSetFromMap(map); // add values in set set.add("Java"); set.add("C"); set.add("C++"); // set and map values are System.out.println("Set is: " + set); System.out.println("Map is: " + map); } }
Let us compile and run the above program, this will produce the following result.
Set is: [Java, C++, C] Map is: {Java=true, C++=true, C=true}
java_util_collections.htm
Advertisements