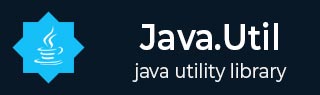
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections frequency() Method
Description
The Java Collections frequency(Collection<?>, Object) method is used to get the number of elements in the specified collection equal to the specified object.
Declaration
Following is the declaration for java.util.Collections.frequency() method.
public static int frequency(Collection<?> c, Object o)
Parameters
c − This is the collection in which to determine the frequency of o.
o − This is the object whose frequency is to be determined.
Return Value
NA
Exception
NullPointerException − This is thrown if c is null.
Counting occurence of an Element in a List of Integers Example
The following example shows the usage of Java Collection frequency(Collection,Object) method. We've created a List object with some integers, printed the original list. Using frequency(Collection, T) method, we've printed the count of a particular element.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list = new ArrayList<>(Arrays.asList(1,2,3,3,5,3)); System.out.println("Initial collection value: " + list); // print the frequency of 3. int result = Collections.frequency(list, 3); System.out.println("3 is present: "+result + " times."); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [1, 2, 3, 3, 5, 3] 3 is present: 3 times.
Counting occurence of an Element in a List of Strings Example
The following example shows the usage of Java Collection frequency(Collection,Object) method. We've created a List object with some strings, printed the original list. Using frequency(Collection, T) method, we've printed the count of a particular element.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> list = new ArrayList<>(Arrays.asList("A","B","C","D","C","C")); System.out.println("Initial collection value: " + list); // print the frequency of C. int result = Collections.frequency(list, "C"); System.out.println("C is present: "+result + " times."); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [A, B, C, D, C, C] C is present: 3 times.
Counting occurence of an Element in a List of Objects Example
The following example shows the usage of Java Collection frequency(Collection,Object) method. We've created a List object with some strings, printed the original list. Using frequency(Collection, T) method, we've printed the count of a particular element.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Student> list = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"),new Student(1, "Julie"))); System.out.println("Initial collection value: " + list); // print the frequency of Julie. int result = Collections.frequency(list, new Student(1, "Julie")); System.out.println("Julie is present: "+result + " times."); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ], [ 1, Julie ]] Julie is present: 2 times.