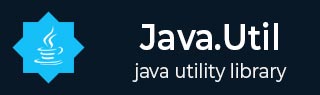
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections copy() Method
Description
The Java Collections copy(List<? super T>, List<? extends T>) method is used to copy all of the elements from one list into another.
Declaration
Following is the declaration for java.util.Collections.copy() method.
public static <T> void copy(List<? super T> dest,List<? extends T> src)
Parameters
dest − This is the destination list.
src − This is the source list.
Return Value
NA
Exception
IndexOutOfBoundsException − This is thrown if the destination list is too small to contain the entire source List.
UnsupportedOperationException − This is thrown if the destination list's list-iterator does not support the set operation.
Copying a List of Integers Example
The following example shows the usage of Java Collection copy(List,List) method to copy a list of Integers. We've created two lists with some integers. Using copy(List,List) method, we're copying content of one list to another while overriding the existing content.
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Integer> srclst = new ArrayList<>(); List<Integer> destlst = new ArrayList<>(); // populate two lists srclst.add(1); srclst.add(2); srclst.add(3); destlst.add(4); destlst.add(5); destlst.add(6); destlst.add(7); // copy into dest list Collections.copy(destlst, srclst); System.out.println("Value of source list: "+srclst); System.out.println("Value of destination list: "+destlst); } }
Output
Let us compile and run the above program, this will produce the following result.
Value of source list: [1, 2, 3] Value of destination list: [1, 2, 3, 7]
Copying a List of Strings Example
The following example shows the usage of Java Collection copy(List,List) method to copy a list of strings. We've created two lists with some strings. Using copy(List,List) method, we're copying content of one list to another while overriding the existing content.
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<String> srclst = new ArrayList<>(); List<String> destlst = new ArrayList<>(); // populate two lists srclst.add("A"); srclst.add("B"); srclst.add("C"); destlst.add("D"); destlst.add("E"); destlst.add("F"); destlst.add("G"); // copy into dest list Collections.copy(destlst, srclst); System.out.println("Value of source list: "+srclst); System.out.println("Value of destination list: "+destlst); } }
Output
Let us compile and run the above program, this will produce the following result.
Value of source list: [A, B, C] Value of destination list: [A, B, C, G]
Copying a List of Objects Example
The following example shows the usage of Java Collection copy(List,List) method to copy a list of Student objects. We've created two lists with some Student objects. Using copy(List,List) method, we're copying content of one list to another while overriding the existing content.
package com.tutorialspoint; import java.util.*; public class CollectionsDemo { public static void main(String args[]) { // create two lists List<Student> srclst = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); List<Student> destlst = new ArrayList<>(Arrays.asList(new Student(4, "Jene"), new Student(5, "Julie"), new Student(6, "Trus"))); // copy into dest list Collections.copy(destlst, srclst); System.out.println("Value of source list: "+srclst); System.out.println("Value of destination list: "+destlst); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Value of source list: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Value of destination list: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]]