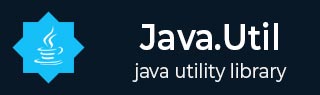
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Collections addAll() Method
Description
The addAll(Collection<? super T>, T..) method is used to add all of the specified elements to the specified collection. It helps in adding multiple elements to a collection in one go.
Declaration
Following is the declaration for java.util.Collections.addAll() method.
public static <T> boolean addAll(Collection<? super T> c, T.. a)
Parameters
c − This is the collection into which elements are to be inserted.
a − This is the elements to insert into c
Return Value
The method call returns 'true' if the collection changed as a result of the call
Exception
UnsupportedOperationException − This is thrown if c does not support the add method.
NullPointerException − This is thrown if elements contains one or more null values and c does not support null elements.
IllegalArgumentException − This is thrown if some aspect of a value in elements prevents it from being added to c.
Adding Multiple Values to a Collection of Integers Example
The following example shows the usage of Java Collection addAll(Collection,T... ) method to add a collection of Integers. We've created a List object with some integers, printed the original list. Using addAll(collection, T...) method, we've added few more elements to the list and then printed the updated list.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list = new ArrayList<>(Arrays.asList(1,2,3,4,5)); System.out.println("Initial collection value: " + list); // add values to this collection Collections.addAll(list, 6, 7, 8); System.out.println("Final collection value: "+list); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [1, 2, 3, 4, 5] Final collection value: [1, 2, 3, 4, 5, 6, 7, 8]
Adding Multiple Values to a Collection of Strings Example
The following example shows the usage of Java Collection addAll(Collection,T... ) method to add a collection of Strings. We've created a List object with some strings, printed the original list. Using addAll(collection, T...) method, we've added few more elements to the list and then printed the updated list.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<String> list = new ArrayList<>(Arrays.asList("Welcome","to","Tutorialspoint")); System.out.println("Initial collection value: " + list); // add values to this collection Collections.addAll(list, "Simply","Easy","Learning"); System.out.println("Final collection value: "+list); } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [Welcome, to, Tutorialspoint] Final collection value: [Welcome, to, Tutorialspoint, Simply, Easy, Learning]
Adding Multiple Values to a Collection of Objects Example
The following example shows the usage of Java Collection addAll(Collection,T... ) method to add a collection of Student objects. We've created a List object with some students, printed the original list. Using addAll(collection, T...) method, we've added few more elements to the list and then printed the updated list.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Student> list = new ArrayList<>(Arrays.asList(new Student(1, "Julie"), new Student(2, "Robert"), new Student(3, "Adam"))); System.out.println("Initial collection value: " + list); // add values to this collection Collections.addAll(list, new Student(4, "Jene"), new Student(5, "John")); System.out.println("Final collection value: "+list); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
Initial collection value: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Final collection value: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ], [ 4, Jene ], [ 5, John ]]