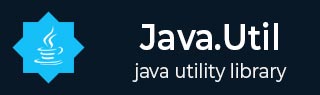
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java Calendar getLeastMaximum() Method
Description
The Java Calendar getLeastMaximum(int field) method returns the lowest maximum value for the given calendar field of this Calendar instance.
Declaration
Following is the declaration for Java.util.Calendar.getLeastMaximum(int field) method
public abstract int getLeastMaximum(int field)
Parameters
field − the given calendar field.
Return Value
Returns lowest maximum value of the given calendar field.
Exception
NA
Getting Least Maximum Year from a Current Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar getLeastMaximum() method. We're creating an instance of a GregorianCalendar of current date. Then we're getting least maximum value of Year using getLeastMaximum() method and printing it.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(); // print the least maximum for year field int result = cal.getLeastMaximum(Calendar.YEAR); System.out.println("The least maximum is: " + result); } }
Output
Let us compile and run the above program, this will produce the following result −
The least maximum is: 292269054
Getting Least Maximum Month from a Current Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar getLeastMaximum() method. We're creating an instance of a GregorianCalendar of current date. Then we're getting least maximum value of Month using getLeastMaximum() method and printing it.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(); // print the least maximum for month field int result = cal.getLeastMaximum(Calendar.MONTH); System.out.println("The least maximum is: " + result); } }
Output
Let us compile and run the above program, this will produce the following result −
The least maximum is: 11
Getting Least Maximum Day from a Current Dated GregorianCalendar Instance Example
The following example shows the usage of Java Calendar getLeastMaximum() method. We're creating an instance of a GregorianCalendar of current date. Then we're getting least maximum value of Day using getLeastMaximum() method and printing it.
package com.tutorialspoint; import java.util.Calendar; import java.util.GregorianCalendar; public class CalendarDemo { public static void main(String[] args) { // create a calendar Calendar cal = new GregorianCalendar(); // print the least maximum for day field int result = cal.getLeastMaximum(Calendar.DATE); System.out.println("The least maximum is: " + result); } }
Output
Let us compile and run the above program, this will produce the following result −
The least maximum is: 28