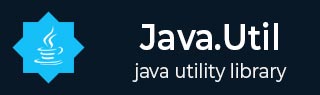
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java BitSet stream() Method
Description
The Java BitSet stream() method returns a stream of indices for which this BitSet contains a set bit. Indices maintains a order, from lowest to highest. Stream size is equal to the value returned by the cardinality() method.
Declaration
Following is the declaration for java.util.BitSet.stream() method
public IntStream stream()
Parameters
NA
Return Value
This method returns a stream of integers representing set indices.
Exception
NA
Getting Stream of Bits from a BitSet Example
The following example shows the usage of Java BitSet stream() method. We're creating two BitSets. We're setting true values at given indexes to the BitSet objects using set() method calls per index and using stream() method we're printing the indices of set bits of bitsets.
package com.tutorialspoint; import java.util.BitSet; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = new BitSet(); BitSet bitset2 = new BitSet(); // assign values to bitset1 bitset1.set(0, 6, true); // assign values to bitset2 bitset2.set(2); bitset2.set(4); bitset2.set(6); bitset2.set(8); bitset2.set(10); bitset1.stream().forEach(i -> System.out.print(i + " ")); System.out.println(); bitset2.stream().forEach(i -> System.out.print(i + " ")); } }
Output
Let us compile and run the above program, this will produce the following result −
0 1 2 3 4 5 2 4 6 8 10
Getting Stream of Bits from a BitSet of Bytes Example
The following example shows the usage of Java BitSet stream() method. We're creating two BitSets using byte[] and using stream() method we're printing the indices of the bitsets.
package com.tutorialspoint; import java.util.BitSet; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = BitSet.valueOf(new byte[] { 0, 1, 2, 3, 4, 5 }); BitSet bitset2 = BitSet.valueOf(new byte[] { 2, 4, 6, 8, 10 }); bitset1.stream().forEach(i -> System.out.print(i + " ")); System.out.println(); bitset2.stream().forEach(i -> System.out.print(i + " ")); } }
Output
Let us compile and run the above program, this will produce the following result −
8 17 24 25 34 40 42 1 10 17 18 27 33 35
Getting Stream of Bits from a BitSet of Longs Example
The following example shows the usage of Java BitSet stream() method. We're creating two BitSets using long[] and using stream() method we're printing the indices of the bitsets.
package com.tutorialspoint; import java.util.BitSet; public class BitSetDemo { public static void main(String[] args) { // create 2 bitsets BitSet bitset1 = BitSet.valueOf(new long[] { 0, 1, 2, 3, 4, 5 }); BitSet bitset2 = BitSet.valueOf(new long[] { 2, 4, 6, 8, 10 }); bitset1.stream().forEach(i -> System.out.print(i + " ")); System.out.println(); bitset2.stream().forEach(i -> System.out.print(i + " ")); } }
Output
Let us compile and run the above program, this will produce the following result −
64 129 192 193 258 320 322 1 66 129 130 195 257 259