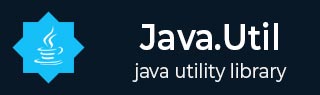
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java ArrayDeque spliterator() Method
Description
The Java ArrayDeque spliterator() method creates a late-binding and fail-fast Spliterator for the elements in this deque. Late binding means the spliterator binds to the source of elements at the time of traversal and not when it is created. Spliterator is a better iterator and provides more controls over items during traversal.
Declaration
Following is the declaration for java.util.ArrayDeque.spliterator() method
public Spliterator<E> spliterator()
Parameters
NA
Return Value
This method returns a Spliterator over the elements in this deque.
Exception
NA
Example 1
The following example shows the usage of Java ArrayDeque spliterator() method with Integers. We're creating an ArrayDeque of Integers, adding some elements, and then Spliterator is created using spliterator() method. The iterator is then used to traverse the items and to print them.
package com.tutorialspoint; import java.util.ArrayDeque; import java.util.Spliterator; public class ArrayDequeDemo { public static void main(String[] args) { // create an empty array deque ArrayDeque<Integer> deque = new ArrayDeque<>(); // use add() method to add elements in the deque deque.add(25); deque.add(30); deque.add(20); deque.add(18); // get the spliterator Spliterator<Integer> spliterator = deque.spliterator(); // use the spliterator to print each item spliterator.forEachRemaining(i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result −
25 30 20 18
Example 2
The following example shows the usage of Java ArrayDeque spliterator() method with Strings. We're creating an ArrayDeque of Strings, adding some elements, and then Spliterator is created using spliterator() method. The iterator is then used to traverse the items and to print them.
package com.tutorialspoint; import java.util.ArrayDeque; import java.util.Spliterator; public class ArrayDequeDemo { public static void main(String[] args) { // create an empty array deque ArrayDeque<String> deque = new ArrayDeque<>(); // use add() method to add elements in the deque deque.add("A"); deque.add("B"); deque.add("C"); deque.add("D"); // get the spliterator Spliterator<String> spliterator = deque.spliterator(); // use the spliterator to print each item spliterator.forEachRemaining(i -> System.out.println(i)); } }
Output
Let us compile and run the above program, this will produce the following result −
A B C D
Example 3
The following example shows the usage of Java ArrayDeque spliterator() method with Student objects. We're creating an ArrayDeque of Student objects, adding some elements, and then Spliterator is created using spliterator() method. The iterator is then used to traverse the items and to print them.
package com.tutorialspoint; import java.util.ArrayDeque; import java.util.Spliterator; public class ArrayDequeDemo { public static void main(String[] args) { // create an empty array deque ArrayDeque<Student> deque = new ArrayDeque<>(); // use add() method to add elements in the deque deque.add(new Student(1, "Julie")); deque.add(new Student(2, "Robert")); deque.add(new Student(3, "Adam")); // get the spliterator Spliterator<Student> spliterator = deque.spliterator(); // use the spliterator to print each item spliterator.forEachRemaining(i -> System.out.println(i)); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } }
Output
Let us compile and run the above program, this will produce the following result −
[ 1, Julie ] [ 2, Robert ] [ 3, Adam ]