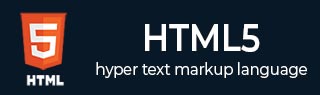
- HTML5 Tutorial
- HTML5 - Home
- HTML5 - Overview
- HTML5 - Syntax
- HTML5 - Attributes
- HTML5 - Events
- HTML5 - Web Forms 2.0
- HTML5 - SVG
- HTML5 - MathML
- HTML5 - Web Storage
- HTML5 - Web SQL Database
- HTML5 - Server-Sent Events
- HTML5 - WebSocket
- HTML5 - Canvas
- HTML5 - Audio & Video
- HTML5 - Geolocation
- HTML5 - Microdata
- HTML5 - Drag & drop
- HTML5 - Web Workers
- HTML5 - IndexedDB
- HTML5 - Web Messaging
- HTML5 - Web CORS
- HTML5 - Web RTC
- HTML5 Demo
- HTML5 - Web Storage
- HTML5 - Server Sent Events
- HTML5 - Canvas
- HTML5 - Audio Players
- HTML5 - Video Players
- HTML5 - Geo-Location
- HTML5 - Drag and Drop
- HTML5 - Web Worker
- HTML5 - Web slide Desk
- HTML5 Tools
- HTML5 - SVG Generator
- HTML5 - MathML
- HTML5 - Velocity Draw
- HTML5 - QR Code
- HTML5 - Validator.nu Validation
- HTML5 - Modernizr
- HTML5 - Validation
- HTML5 - Online Editor
- HTML5 - Color Code Builder
- HTML5 Useful References
- HTML5 - Quick Guide
- HTML5 - Color Names
- HTML5 - Fonts Reference
- HTML5 - URL Encoding
- HTML5 - Entities
- HTML5 - Char Encodings
- HTML5 Tag Reference
- HTML5 - Question and Answers
- HTML5 - Tags Reference
- HTML5 - Deprecated Tags
- HTML5 - New Tags
- HTML5 Resources
- HTML5 - Useful Resources
- HTML5 - Discussion
HTML5 - Canvas
HTML5 element <canvas> gives you an easy and powerful way to draw graphics using JavaScript. It can be used to draw graphs, make photo compositions or do simple (and not so simple) animations.
Here is a simple <canvas> element which has only two specific attributes width and height plus all the core HTML5 attributes like id, name and class, etc.
<canvas id = "mycanvas" width = "100" height = "100"></canvas>
You can easily find that <canvas> element in the DOM using getElementById() method as follows −
var canvas = document.getElementById("mycanvas");
Let us see a simple example on using <canvas> element in HTML5 document.
<!DOCTYPE HTML> <html> <head> <style> #mycanvas{border:1px solid red;} </style> </head> <body> <canvas id = "mycanvas" width = "100" height = "100"></canvas> </body> </html>
This will produce the following result −
The Rendering Context
The <canvas> is initially blank, and to display something, a script first needs to access the rendering context and draw on it.
The canvas element has a DOM method called getContext, used to obtain the rendering context and its drawing functions. This function takes one parameter, the type of context2d.
Following is the code to get required context along with a check if your browser supports <canvas> element −
var canvas = document.getElementById("mycanvas"); if (canvas.getContext) { var ctx = canvas.getContext('2d'); // drawing code here } else { // canvas-unsupported code here }
Browser Support
The latest versions of Firefox, Safari, Chrome and Opera all support for HTML5 Canvas but IE8 does not support canvas natively.
You can use ExplorerCanvas to have canvas support through Internet Explorer. You just need to include this JavaScript as follows −
<!--[if IE]><script src = "excanvas.js"></script><![endif]-->
HTML5 Canvas Examples
This tutorial covers the following examples related to HTML5 <canvas> element.
Sr.No. | Examples & Description |
---|---|
1 | Drawing Rectangles
Learn how to draw rectangle using HTML5 <canvas> element |
2 | Drawing Paths
Learn how to make shapes using paths in HTML5 <canvas> element |
3 | Drawing Lines
Learn how to draw lines using HTML5 <canvas> element |
4 | Drawing Bezier
Learn how to draw Bezier curve using HTML5 <canvas> element |
5 | Drawing Quadratic
Learn how to draw quadratic curve using HTML5 <canvas> element |
6 | Using Images
Learn how to use images with HTML5 <canvas> element |
7 | Create Gradients
Learn how to create gradients using HTML5 <canvas> element |
8 | Styles and Colors
Learn how to apply styles and colors using HTML5 <canvas> element |
9 | Text and Fonts
Learn how to draw amazing text using different fonts and their size. |
10 | Pattern and Shadow
Learn how to draw different patterns and drop shadows. |
11 | Canvas States
Learn how to save and restore canvas states while doing complex drawings on a canvas. |
12 | Canvas Translation
This method is used to move the canvas and its origin to a different point in the grid. |
13 | Canvas Rotation
This method is used to rotate the canvas around the current origin. |
14 | Canvas Scaling
This method is used to increase or decrease the units in a canvas grid. |
15 | Canvas Transform
These methods allow modifications directly to the transformation matrix. |
16 | Canvas Composition
This method is used to mask off certain areas or clear sections from the canvas. |
17 | Canvas Animation
Learn how to create basic animation using HTML5 canvas and JavaScript. |