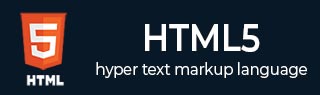
- HTML5 Tutorial
- HTML5 - Home
- HTML5 - Overview
- HTML5 - Syntax
- HTML5 - Attributes
- HTML5 - Events
- HTML5 - Web Forms 2.0
- HTML5 - SVG
- HTML5 - MathML
- HTML5 - Web Storage
- HTML5 - Web SQL Database
- HTML5 - Server-Sent Events
- HTML5 - WebSocket
- HTML5 - Canvas
- HTML5 - Audio & Video
- HTML5 - Geolocation
- HTML5 - Microdata
- HTML5 - Drag & drop
- HTML5 - Web Workers
- HTML5 - IndexedDB
- HTML5 - Web Messaging
- HTML5 - Web CORS
- HTML5 - Web RTC
- HTML5 Demo
- HTML5 - Web Storage
- HTML5 - Server Sent Events
- HTML5 - Canvas
- HTML5 - Audio Players
- HTML5 - Video Players
- HTML5 - Geo-Location
- HTML5 - Drag and Drop
- HTML5 - Web Worker
- HTML5 - Web slide Desk
- HTML5 Tools
- HTML5 - SVG Generator
- HTML5 - MathML
- HTML5 - Velocity Draw
- HTML5 - QR Code
- HTML5 - Validator.nu Validation
- HTML5 - Modernizr
- HTML5 - Validation
- HTML5 - Online Editor
- HTML5 - Color Code Builder
- HTML5 Useful References
- HTML5 - Quick Guide
- HTML5 - Color Names
- HTML5 - Fonts Reference
- HTML5 - URL Encoding
- HTML5 - Entities
- HTML5 - Char Encodings
- HTML5 Tag Reference
- HTML5 - Question and Answers
- HTML5 - Tags Reference
- HTML5 - Deprecated Tags
- HTML5 - New Tags
- HTML5 Resources
- HTML5 - Useful Resources
- HTML5 - Discussion
HTML5 - Server Sent Events
Conventional web applications generate events which are dispatched to the web server. For example, a simple click on a link requests a new page from the server.
The type of events which are flowing from web browser to the web server may be called client-sent events.
Along with HTML5, WHATWG Web Applications 1.0 introduces events which flow from web server to the web browsers and they are called Server-Sent Events (SSE). Using SSE you can push DOM events continuously from your web server to the visitor's browser.
The event streaming approach opens a persistent connection to the server, sending data to the client when new information is available, eliminating the need for continuous polling.
Server-sent events standardize how we stream data from the server to the client.
Web Application for SSE
To use Server-Sent Events in a web application, you would need to add an <eventsource> element to the document.
The src attribute of <eventsource> element should point to an URL which should provide a persistent HTTP connection that sends a data stream containing the events.
The URL would point to a PHP, PERL or any Python script which would take care of sending event data consistently. Following is a simple example of web application which would expect server time.
<!DOCTYPE HTML> <html> <head> <script type = "text/javascript"> /* Define event handling logic here */ </script> </head> <body> <div id = "sse"> <eventsource src = "/cgi-bin/ticker.cgi" /> </div> <div id = "ticker"> <TIME> </div> </body> </html>
Server Side Script for SSE
A server side script should send Content-type header specifying the type text/event-stream as follows.
print "Content-Type: text/event-stream\n\n";
After setting Content-Type, server side script would send an Event: tag followed by event name. Following example would send Server-Time as event name terminated by a new line character.
print "Event: server-time\n";
Final step is to send event data using Data: tag which would be followed by integer of string value terminated by a new line character as follows −
$time = localtime(); print "Data: $time\n";
Finally, following is complete ticker.cgi written in Perl −
#!/usr/bin/perl print "Content-Type: text/event-stream\n\n"; while(true) { print "Event: server-time\n"; $time = localtime(); print "Data: $time\n"; sleep(5); }
Handle Server-Sent Events
Let us modify our web application to handle server-sent events. Following is the final example.
<!DOCTYPE HTML> <html> <head> <script type = "text/javascript"> document.getElementsByTagName("eventsource")[0].addEventListener("server-time", eventHandler, false); function eventHandler(event) { // Alert time sent by the server document.querySelector('#ticker').innerHTML = event.data; } </script> </head> <body> <div id = "sse"> <eventsource src = "/cgi-bin/ticker.cgi" /> </div> <div id = "ticker" name = "ticker"> [TIME] </div> </body> </html>
Before testing Server-Sent events, I would suggest that you make sure your web browser supports this concept.