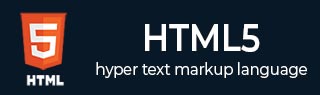
- HTML5 Tutorial
- HTML5 - Home
- HTML5 - Overview
- HTML5 - Syntax
- HTML5 - Attributes
- HTML5 - Events
- HTML5 - Web Forms 2.0
- HTML5 - SVG
- HTML5 - MathML
- HTML5 - Web Storage
- HTML5 - Web SQL Database
- HTML5 - Server-Sent Events
- HTML5 - WebSocket
- HTML5 - Canvas
- HTML5 - Audio & Video
- HTML5 - Geolocation
- HTML5 - Microdata
- HTML5 - Drag & drop
- HTML5 - Web Workers
- HTML5 - IndexedDB
- HTML5 - Web Messaging
- HTML5 - Web CORS
- HTML5 - Web RTC
- HTML5 Demo
- HTML5 - Web Storage
- HTML5 - Server Sent Events
- HTML5 - Canvas
- HTML5 - Audio Players
- HTML5 - Video Players
- HTML5 - Geo-Location
- HTML5 - Drag and Drop
- HTML5 - Web Worker
- HTML5 - Web slide Desk
- HTML5 Tools
- HTML5 - SVG Generator
- HTML5 - MathML
- HTML5 - Velocity Draw
- HTML5 - QR Code
- HTML5 - Validator.nu Validation
- HTML5 - Modernizr
- HTML5 - Validation
- HTML5 - Online Editor
- HTML5 - Color Code Builder
- HTML5 Useful References
- HTML5 - Quick Guide
- HTML5 - Color Names
- HTML5 - Fonts Reference
- HTML5 - URL Encoding
- HTML5 - Entities
- HTML5 - Char Encodings
- HTML5 Tag Reference
- HTML5 - Question and Answers
- HTML5 - Tags Reference
- HTML5 - Deprecated Tags
- HTML5 - New Tags
- HTML5 Resources
- HTML5 - Useful Resources
- HTML5 - Discussion
HTML5 Canvas - Save and Restore States
HTML5 canvas provides two important methods to save and restore the canvas states. The canvas drawing state is basically a snapshot of all the styles and transformations that have been applied and consists of the followings −
The transformations such as translate, rotate and scale etc.
The current clipping region.
The current values of the following attributes − strokeStyle, fillStyle, globalAlpha, lineWidth, lineCap, lineJoin, miterLimit, shadowOffsetX, shadowOffsetY, shadowBlur, shadowColor, globalCompositeOperation, font, textAlign, textBaseline.
Canvas states are stored on a stack every time the save method is called, and the last saved state is returned from the stack every time the restore method is called.
Sr.No. | Method and Description |
---|---|
1 | save() This method pushes the current state onto the stack.. |
2 | restore() This method pops the top state on the stack, restoring the context to that state. |
Example
Following is a simple example which makes use of above mentioned methods to show how the restore is called, to restore the original state and the last rectangle is once again drawn in black.
<!DOCTYPE HTML> <html> <head> <style> #test { width: 100px; height:100px; margin: 0px auto; } </style> <script type = "text/javascript"> function drawShape() { // get the canvas element using the DOM var canvas = document.getElementById('mycanvas'); // Make sure we don't execute when canvas isn't supported if (canvas.getContext) { // use getContext to use the canvas for drawing var ctx = canvas.getContext('2d'); // draw a rectangle with default settings ctx.fillRect(0,0,150,150); // Save the default state ctx.save(); // Make changes to the settings ctx.fillStyle = '#66FFFF' ctx.fillRect( 15,15,120,120); // Save the current state ctx.save(); // Make the new changes to the settings ctx.fillStyle = '#993333' ctx.globalAlpha = 0.5; ctx.fillRect(30,30,90,90); // Restore previous state ctx.restore(); // Draw a rectangle with restored settings ctx.fillRect(45,45,60,60); // Restore original state ctx.restore(); // Draw a rectangle with restored settings ctx.fillRect(40,40,90,90); } else { alert('You need Safari or Firefox 1.5+ to see this demo.'); } } </script> </head> <body id = "test" onload = "drawShape();"> <canvas id = "mycanvas"></canvas> </body> </html>
The above example would produce the following result −