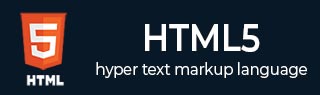
- HTML5 Tutorial
- HTML5 - Home
- HTML5 - Overview
- HTML5 - Syntax
- HTML5 - Attributes
- HTML5 - Events
- HTML5 - Web Forms 2.0
- HTML5 - SVG
- HTML5 - MathML
- HTML5 - Web Storage
- HTML5 - Web SQL Database
- HTML5 - Server-Sent Events
- HTML5 - WebSocket
- HTML5 - Canvas
- HTML5 - Audio & Video
- HTML5 - Geolocation
- HTML5 - Microdata
- HTML5 - Drag & drop
- HTML5 - Web Workers
- HTML5 - IndexedDB
- HTML5 - Web Messaging
- HTML5 - Web CORS
- HTML5 - Web RTC
- HTML5 Demo
- HTML5 - Web Storage
- HTML5 - Server Sent Events
- HTML5 - Canvas
- HTML5 - Audio Players
- HTML5 - Video Players
- HTML5 - Geo-Location
- HTML5 - Drag and Drop
- HTML5 - Web Worker
- HTML5 - Web slide Desk
- HTML5 Tools
- HTML5 - SVG Generator
- HTML5 - MathML
- HTML5 - Velocity Draw
- HTML5 - QR Code
- HTML5 - Validator.nu Validation
- HTML5 - Modernizr
- HTML5 - Validation
- HTML5 - Online Editor
- HTML5 - Color Code Builder
- HTML5 Useful References
- HTML5 - Quick Guide
- HTML5 - Color Names
- HTML5 - Fonts Reference
- HTML5 - URL Encoding
- HTML5 - Entities
- HTML5 - Char Encodings
- HTML5 Tag Reference
- HTML5 - Question and Answers
- HTML5 - Tags Reference
- HTML5 - Deprecated Tags
- HTML5 - New Tags
- HTML5 Resources
- HTML5 - Useful Resources
- HTML5 - Discussion
HTML5 - SVG
SVG stands for Scalable Vector Graphics and it is a language for describing 2D-graphics and graphical applications in XML and the XML is then rendered by an SVG viewer.
SVG is mostly useful for vector type diagrams like Pie charts, Two-dimensional graphs in an X,Y coordinate system etc.
SVG became a W3C Recommendation 14. January 2003 and you can check latest version of SVG specification at SVG Specification.
Viewing SVG Files
Most of the web browsers can display SVG just like they can display PNG, GIF, and JPG. Internet Explorer users may have to install the Adobe SVG Viewer to be able to view SVG in the browser.
Embedding SVG in HTML5
HTML5 allows embedding SVG directly using <svg>...</svg> tag which has following simple syntax −
<svg xmlns = "http://www.w3.org/2000/svg"> ... </svg>
Firefox 3.7 has also introduced a configuration option ("about:config") where you can enable HTML5 using the following steps −
Type about:config in your Firefox address bar.
Click the "I'll be careful, I promise!" button on the warning message that appears (and make sure you adhere to it!).
Type html5.enable into the filter bar at the top of the page.
Currently it would be disabled, so click it to toggle the value to true.
Now your Firefox HTML5 parser should be enabled and you should be able to experiment with the following examples.
HTML5 − SVG Circle
Following is the HTML5 version of an SVG example which would draw a circle using <circle> tag −
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-20%); -ms-transform: translateX(-20%); transform: translateX(-20%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Circle</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <circle id = "redcircle" cx = "50" cy = "50" r = "50" fill = "red" /> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Rectangle
Following is the HTML5 version of an SVG example which would draw a rectangle using <rect> tag −
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-50%); -ms-transform: translateX(-50%); transform: translateX(-50%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Rectangle</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <rect id = "redrect" width = "300" height = "100" fill = "red" /> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Line
Following is the HTML5 version of an SVG example which would draw a line using <line> tag −
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-50%); -ms-transform: translateX(-50%); transform: translateX(-50%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Line</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <line x1 = "0" y1 = "0" x2 = "200" y2 = "100" style = "stroke:red;stroke-width:2"/> </svg> </body> </html>
You can use the style attribute which allows you to set additional style information like stroke and fill colors, width of the stroke, etc.
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Ellipse
Following is the HTML5 version of an SVG example which would draw an ellipse using <ellipse> tag −
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-40%); -ms-transform: translateX(-40%); transform: translateX(-40%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Ellipse</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <ellipse cx = "100" cy = "50" rx = "100" ry = "50" fill = "red" /> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Polygon
Following is the HTML5 version of an SVG example which would draw a polygon using <polygon> tag −
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-50%); -ms-transform: translateX(-50%); transform: translateX(-50%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Polygon</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <polygon points = "20,10 300,20, 170,50" fill = "red" /> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Polyline
Following is the HTML5 version of an SVG example which would draw a polyline using <polyline> tag −
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-20%); -ms-transform: translateX(-20%); transform: translateX(-20%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Polyline</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <polyline points = "0,0 0,20 20,20 20,40 40,40 40,60" fill = "red" /> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Gradients
Following is the HTML5 version of an SVG example which would draw an ellipse using <ellipse> tag and would use <radialGradient> tag to define an SVG radial gradient.
Similarly, you can use <linearGradient> tag to create SVG linear gradient.
<!DOCTYPE html> <html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-40%); -ms-transform: translateX(-40%); transform: translateX(-40%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Gradient Ellipse</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <defs> <radialGradient id="gradient" cx = "50%" cy = "50%" r = "50%" fx = "50%" fy = "50%"> <stop offset = "0%" style = "stop-color:rgb(200,200,200); stop-opacity:0"/> <stop offset = "100%" style = "stop-color:rgb(0,0,255); stop-opacity:1"/> </radialGradient> </defs> <ellipse cx = "100" cy = "50" rx = "100" ry = "50" style = "fill:url(#gradient)" /> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.
HTML5 − SVG Star
Following is the HTML5 version of an SVG example which would draw a star using <polygon> tag.
<html> <head> <style> #svgelem { position: relative; left: 50%; -webkit-transform: translateX(-40%); -ms-transform: translateX(-40%); transform: translateX(-40%); } </style> <title>SVG</title> <meta charset = "utf-8" /> </head> <body> <h2 align = "center">HTML5 SVG Star</h2> <svg id = "svgelem" height = "200" xmlns = "http://www.w3.org/2000/svg"> <polygon points = "100,10 40,180 190,60 10,60 160,180" fill = "red"/> </svg> </body> </html>
This would produce the following result in HTML5 enabled latest version of Firefox.