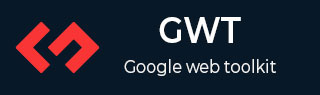
- GWT Tutorial
- GWT - Home
- GWT - Overview
- GWT - Environment Setup
- GWT - Applications
- GWT - Create Application
- GWT - Deploy Application
- GWT - Style with CSS
- GWT - Basic Widgets
- GWT - Form Widgets
- GWT - Complex widgets
- GWT - Layout Panels
- GWT - Event Handling
- GWT - Custom Widgets
- GWT - UIBinder
- GWT - RPC Communication
- GWT - JUnit Integration
- GWT - Debugging Application
- GWT - Internationalization
- GWT - History Class
- GWT - Bookmark Support
- GWT - Logging Framework
- GWT Useful Resources
- GWT - Questions and Answers
- GWT - Quick Guide
- GWT - Useful Resources
- GWT - Discussion
GWT - HorizontalSplitPanel Widget
Introduction
The HorizantalSplitPanel widget represents a panel that arranges two widgets in a single horizontal row and allows the user to interactively change the proportion of the width dedicated to each of the two widgets. Widgets contained within a HorizontalSplitPanel will be automatically decorated with scrollbars when necessary.
Class Declaration
Following is the declaration for com.google.gwt.user.client.ui.HorizontalSplitPanel class −
@Deprecated public final class HorizontalSplitPanel extends Panel
CSS Style Rules
Following default CSS Style rules will be applied to all the HorizontalSpiltPanel widget. You can override it as per your requirements.
.gwt-HorizontalSplitPanel { } .gwt-HorizontalSplitPanel hsplitter { }
Class Constructors
Sr.No. | Constructor & Description |
---|---|
1 |
HorizontalSplitPanel() Deprecated. |
2 |
HorizontalSplitPanel(HorizontalSplitPanel.Resources resources) Deprecated. Creates an empty horizontal split panel. |
3 |
HorizontalSplitPanel(HorizontalSplitPanel (HorizontalSplitPanelImages images)) Deprecated. replaced by HorizontalSplitPanel(Resources) |
Class Methods
Sr.No. | Function name & Description |
---|---|
1 |
void add(Widget w) Deprecated. Adds a widget to a pane in the HorizontalSplitPanel. |
2 |
protected Element getElement(int index) Deprecated. Gets the content element for the given index. |
3 |
Widget getEndOfLineWidget() Deprecated. Gets the widget in the pane that is at the end of the line direction for the layout. |
4 |
Widget getLeftWidget() Deprecated. Gets the widget in the left side of the panel. |
5 |
Widget getRightWidget() Deprecated. Gets the widget in the right side of the panel. |
6 |
protected Element getSplitElement() Deprecated. Gets the element that is acting as the splitter. |
7 |
Widget getStartOfLineWidget() Deprecated. Gets the widget in the pane that is at the start of the line direction for the layout. |
8 |
protected Widget getWidget(int index) Deprecated. Gets one of the contained widgets. |
9 |
boolean isResizing() Deprecated. Indicates whether the split panel is being resized. |
10 |
java.util.Iterator<Widget> iterator() Deprecated. Gets an iterator for the contained widgets. |
11 |
void onBrowserEvent(Event event) Deprecated. Fired whenever a browser event is received. |
12 |
protected void onEnsureDebugId(java.lang.String baseID) Deprecated. Affected Elements: -splitter = the container containing the splitter element. -right = the container on the right side of the splitter. -left = the container on the left side of the splitter. |
13 |
protected void onLoad() Deprecated. This method is called immediately after a widget becomes attached to the browser's document. |
14 |
protected void onUnload() Deprecated. This method is called immediately before a widget will be detached from the browser's document. |
15 |
boolean remove(Widget widget) Deprecated. Removes a child widget. |
16 |
void setEndOfLineWidget(Widget w) Deprecated. Sets the widget in the pane that is at the end of the line direction for the layout. |
17 |
void setLeftWidget(Widget w) Deprecated. Sets the widget in the left side of the panel. |
18 |
void setRightWidget(Widget w) Deprecated. Sets the widget in the right side of the panel. |
19 |
void setSplitPosition(java.lang.String pos) Deprecated. Moves the position of the splitter. |
20 |
void setStartOfLineWidget(Widget w) Deprecated. Sets the widget in the pane that is at the start of the line direction for the layout. |
21 |
protected void setWidget(int index, Widget w) Deprecated. Sets one of the contained widgets. |
Methods Inherited
This class inherits methods from the following classes −
com.google.gwt.user.client.ui.UIObject
com.google.gwt.user.client.ui.Widget
com.google.gwt.user.client.ui.Panel
java.lang.Object
HorizontalSplitPanel Widget Example
This example will take you through simple steps to show usage of a HorizontalSplitPanel Widget in GWT. Follow the following steps to update the GWT application we created in GWT - Create Application chapter −
Step | Description |
---|---|
1 | Create a project with a name HelloWorld under a package com.tutorialspoint as explained in the GWT - Create Application chapter. |
2 | Modify HelloWorld.gwt.xml, HelloWorld.css, HelloWorld.html and HelloWorld.java as explained below. Keep rest of the files unchanged. |
3 | Compile and run the application to verify the result of the implemented logic. |
Following is the content of the modified module descriptor src/com.tutorialspoint/HelloWorld.gwt.xml.
<?xml version = "1.0" encoding = "UTF-8"?> <module rename-to = 'helloworld'> <!-- Inherit the core Web Toolkit stuff. --> <inherits name = 'com.google.gwt.user.User'/> <!-- Inherit the default GWT style sheet. --> <inherits name = 'com.google.gwt.user.theme.clean.Clean'/> <!-- Specify the app entry point class. --> <entry-point class = 'com.tutorialspoint.client.HelloWorld'/> <!-- Specify the paths for translatable code --> <source path = 'client'/> <source path = 'shared'/> </module>
Following is the content of the modified Style Sheet file war/HelloWorld.css.
body { text-align: center; font-family: verdana, sans-serif; } h1 { font-size: 2em; font-weight: bold; color: #777777; margin: 40px 0px 70px; text-align: center; }
Following is the content of the modified HTML host file war/HelloWorld.html.
<html> <head> <title>Hello World</title> <link rel = "stylesheet" href = "HelloWorld.css"/> <script language = "javascript" src = "helloworld/helloworld.nocache.js"> </script> </head> <body> <h1>HorizontalSplitPanel Widget Demonstration</h1> <div id = "gwtContainer"></div> </body> </html>
Let us have following content of Java file src/com.tutorialspoint/HelloWorld.java which will demonstrate use of HorizontalSplitPanel widget.
package com.tutorialspoint.client; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.user.client.ui.CheckBox; import com.google.gwt.user.client.ui.DecoratorPanel; import com.google.gwt.user.client.ui.HTML; import com.google.gwt.user.client.ui.HorizontalSplitPanel; import com.google.gwt.user.client.ui.RootPanel; import com.google.gwt.user.client.ui.VerticalPanel; public class HelloWorld implements EntryPoint { public void onModuleLoad() { // Create a Horizontal Split Panel HorizontalSplitPanel horizontalSplitPanel = new HorizontalSplitPanel(); horizontalSplitPanel.setSize("300px", "200px"); horizontalSplitPanel.setSplitPosition("30%"); // Add some content String randomText = "This is a sample text."; for (int i = 0; i < 2; i++) { randomText += randomText; } horizontalSplitPanel.setRightWidget(new HTML(randomText)); horizontalSplitPanel.setLeftWidget(new HTML(randomText)); DecoratorPanel decoratorPanel = new DecoratorPanel(); decoratorPanel.add(horizontalSplitPanel); // Add the widgets to the root panel. RootPanel.get().add(decoratorPanel); } }
Once you are ready with all the changes done, let us compile and run the application in development mode as we did in GWT - Create Application chapter. If everything is fine with your application, this will produce following result −
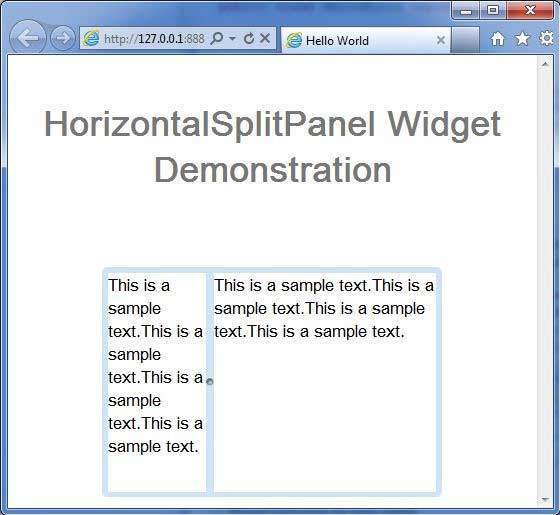