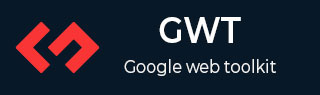
- GWT Tutorial
- GWT - Home
- GWT - Overview
- GWT - Environment Setup
- GWT - Applications
- GWT - Create Application
- GWT - Deploy Application
- GWT - Style with CSS
- GWT - Basic Widgets
- GWT - Form Widgets
- GWT - Complex widgets
- GWT - Layout Panels
- GWT - Event Handling
- GWT - Custom Widgets
- GWT - UIBinder
- GWT - RPC Communication
- GWT - JUnit Integration
- GWT - Debugging Application
- GWT - Internationalization
- GWT - History Class
- GWT - Bookmark Support
- GWT - Logging Framework
- GWT Useful Resources
- GWT - Questions and Answers
- GWT - Quick Guide
- GWT - Useful Resources
- GWT - Discussion
GWT - Bookmark Support
GWT supports browser history management using a History class for which you can reference GWT - History Class chapter.
GWT uses a term token which is simply a string that the application can parse to return to a particular state. Application will save this token in browser's history as URL fragment.
In GWT - History Class chapter, we handle the token creation and setting in the history by writing code.
In this article, we will discuss a special widget Hyperlink which does the token creation and history management for us automatically and gives application capability of bookmarking.
Bookmarking Example
This example will take you through simple steps to demonstrate Bookmarking of a GWT application.
The following steps to update the GWT application we created in GWT - Create Application chapter −
Step | Description |
---|---|
1 | Create a project with a name HelloWorld under a package com.tutorialspoint as explained in the GWT - Create Application chapter. |
2 | Modify HelloWorld.gwt.xml, HelloWorld.css, HelloWorld.html and HelloWorld.java as explained below. Keep rest of the files unchanged. |
3 | Compile and run the application to verify the result of the implemented logic. |
Following is the content of the modified module descriptor src/com.tutorialspoint/HelloWorld.gwt.xml.
<?xml version = "1.0" encoding = "UTF-8"?> <module rename-to = 'helloworld'> <!-- Inherit the core Web Toolkit stuff. --> <inherits name = 'com.google.gwt.user.User'/> <!-- Inherit the default GWT style sheet. --> <inherits name = 'com.google.gwt.user.theme.clean.Clean'/> <!-- Specify the app entry point class. --> <entry-point class = 'com.tutorialspoint.client.HelloWorld'/> <!-- Specify the paths for translatable code --> <source path = 'client'/> <source path = 'shared'/> </module>
Following is the content of the modified Style Sheet file war/HelloWorld.css.
body { text-align: center; font-family: verdana, sans-serif; } h1 { font-size: 2em; font-weight: bold; color: #777777; margin: 40px 0px 70px; text-align: center; }
Following is the content of the modified HTML host file war/HelloWorld.html
<html> <head> <title>Hello World</title> <link rel = "stylesheet" href = "HelloWorld.css"/> <script language = "javascript" src = "helloworld/helloworld.nocache.js"> </script> </head> <body> <iframe src = "javascript:''"id = "__gwt_historyFrame" style = "width:0;height:0;border:0"></iframe> <h1> Bookmarking Demonstration</h1> <div id = "gwtContainer"></div> </body> </html>
Let us have following content of Java file src/com.tutorialspoint/HelloWorld.java using which we will demonstrate Bookmarking in GWT Code.
package com.tutorialspoint.client; import com.google.gwt.core.client.EntryPoint; import com.google.gwt.event.logical.shared.ValueChangeEvent; import com.google.gwt.event.logical.shared.ValueChangeHandler; import com.google.gwt.user.client.History; import com.google.gwt.user.client.ui.HTML; import com.google.gwt.user.client.ui.HorizontalPanel; import com.google.gwt.user.client.ui.Hyperlink; import com.google.gwt.user.client.ui.RootPanel; import com.google.gwt.user.client.ui.TabPanel; import com.google.gwt.user.client.ui.VerticalPanel; public class HelloWorld implements EntryPoint { private TabPanel tabPanel; private void selectTab(String historyToken){ /* parse the history token */ try { if (historyToken.substring(0, 9).equals("pageIndex")) { String tabIndexToken = historyToken.substring(9, 10); int tabIndex = Integer.parseInt(tabIndexToken); /* Select the specified tab panel */ tabPanel.selectTab(tabIndex); } else { tabPanel.selectTab(0); } } catch (IndexOutOfBoundsException e) { tabPanel.selectTab(0); } } /** * This is the entry point method. */ public void onModuleLoad() { /* create a tab panel to carry multiple pages */ tabPanel = new TabPanel(); /* create pages */ HTML firstPage = new HTML("<h1>We are on first Page.</h1>"); HTML secondPage = new HTML("<h1>We are on second Page.</h1>"); HTML thirdPage = new HTML("<h1>We are on third Page.</h1>"); String firstPageTitle = "First Page"; String secondPageTitle = "Second Page"; String thirdPageTitle = "Third Page"; Hyperlink firstPageLink = new Hyperlink("1", "pageIndex0"); Hyperlink secondPageLink = new Hyperlink("2", "pageIndex1"); Hyperlink thirdPageLink = new Hyperlink("3", "pageIndex2"); HorizontalPanel linksHPanel = new HorizontalPanel(); linksHPanel.setSpacing(10); linksHPanel.add(firstPageLink); linksHPanel.add(secondPageLink); linksHPanel.add(thirdPageLink); /* If the application starts with no history token, redirect to a pageIndex0 */ String initToken = History.getToken(); if (initToken.length() == 0) { History.newItem("pageIndex0"); initToken = "pageIndex0"; } tabPanel.setWidth("400"); /* add pages to tabPanel*/ tabPanel.add(firstPage, firstPageTitle); tabPanel.add(secondPage,secondPageTitle); tabPanel.add(thirdPage, thirdPageTitle); /* add value change handler to History * this method will be called, when browser's Back button * or Forward button are clicked. * and URL of application changes. * */ History.addValueChangeHandler(new ValueChangeHandler<String>() { @Override public void onValueChange(ValueChangeEvent<String> event) { selectTab(event.getValue()); } }); selectTab(initToken); VerticalPanel vPanel = new VerticalPanel(); vPanel.setSpacing(10); vPanel.add(tabPanel); vPanel.add(linksHPanel); /* add controls to RootPanel */ RootPanel.get().add(vPanel); } }
Once you are ready with all the changes done, let us compile and run the application in development mode as we did in GWT - Create Application chapter. If everything is fine with your application, this will produce following result −
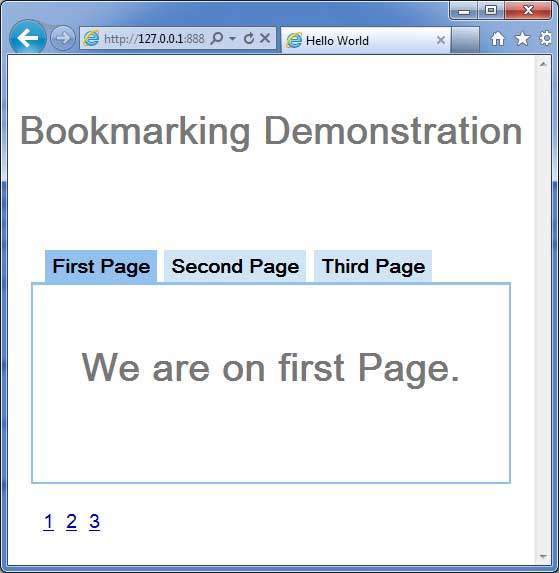
Now click on 1, 2 or 3. You can notice that the tab changes with indexes.
You should notice, when you click on 1,2 or 3 ,application url is changed and #pageIndex is added to the url
You can also see that browser's back and forward buttons are enabled now.
Use back and forward button of the browser and you will see the different tabs get selected accordingly.
Right Click on 1, 2 or 3. You can see options like open, open in new window, open in new tab, add to favourites etc.
Right Click on 3. Choose add to favourites. Save bookmark as page 3.
Open favourites and choose page 3. You will see the third tab selected.