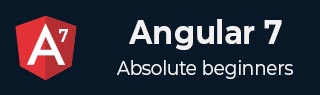
- Angular7 Tutorial
- Angular7 - Home
- Angular7 - Overview
- Angular7 - Environment Setup
- Angular7 - Project Setup
- Angular7 - Components
- Angular7 - Modules
- Angular7 - Data Binding
- Angular7 - Event Binding
- Angular7 - Templates
- Angular7 - Directives
- Angular7 - Pipes
- Angular7 - Routing
- Angular7 - Services
- Angular7 - Http Client
- Angular7 - CLI Prompts
- Angular7 - Forms
- Materials/CDK-Virtual Scrolling
- Angular7 - Materials/CDK-Drag & Drop
- Angular7 - Animations
- Angular7 - Materials
- Testing & Building Angular7 Project
- Angular7 Useful Resources
- Angular7 - Quick Guide
- Angular7 - Useful Resources
- Angular7 - Discussion
Angular7 - Http Client
HttpClient will help us fetch external data, post to it, etc. We need to import the http module to make use of the http service. Let us consider an example to understand how to make use of the http service.
To start using the http service, we need to import the module in app.module.ts as shown below −
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule , RoutingComponent} from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { ChangeTextDirective } from './change-text.directive'; import { SqrtPipe } from './app.sqrt'; import { MyserviceService } from './myservice.service'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [ SqrtPipe, AppComponent, NewCmpComponent, ChangeTextDirective, RoutingComponent ], imports: [ BrowserModule, AppRoutingModule, HttpClientModule ], providers: [MyserviceService], bootstrap: [AppComponent] }) export class AppModule { }
If you see the highlighted code, we have imported the HttpClientModule from @angular/common/http and the same is also added in the imports array.
We will fetch the data from the server using httpclient module declared above. We will do that inside a service we created in the previous chapter and use the data inside the components which we want.
myservice.service.ts
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Injectable({ providedIn: 'root' }) export class MyserviceService { private finaldata = []; private apiurl = "http://jsonplaceholder.typicode.com/users"; constructor(private http: HttpClient) { } getData() { return this.http.get(this.apiurl); } }
There is a method added called getData that returns the data fetched for the url given.
The method getData is called from app.component.ts as follows −
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; public persondata = []; constructor(private myservice: MyserviceService) {} ngOnInit() { this.myservice.getData().subscribe((data) => { this.persondata = Array.from(Object.keys(data), k=>data[k]); console.log(this.persondata); }); } }
We are calling the method getData which gives back an observable type data. The subscribe method is used on it which has an arrow function with the data we need.
When we check in the browser, the console displays the data as shown below −
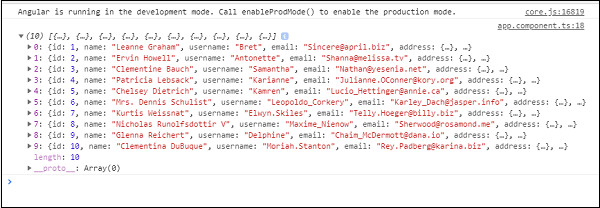
Let us use the data in app.component.html as follows −
<h3>Users Data</h3> <ul> <li *ngFor="let item of persondata; let i = index"< {{item.name}} </li> </ul>
Output
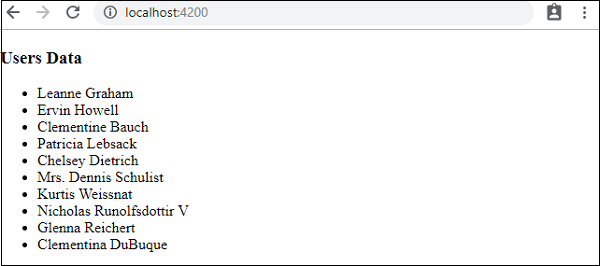