
- Entity Framework Tutorial
- Entity Framework - Home
- Entity Framework - Overview
- Entity Framework - Architecture
- Entity F - Environment Setup
- Entity Framework - Database Setup
- Entity Framework - Data Model
- Entity Framework - DbContext
- Entity Framework - Types
- Entity Framework - Relationships
- Entity Framework - Lifecycle
- Entity F - Code First Approach
- Entity F - Model First Approach
- Entity F - Database First Approach
- Entity Framework - DEV Approaches
- Entity F - Database Operations
- Entity Framework - Concurrency
- Entity Framework - Transaction
- Entity Framework - Views
- Entity Framework - Index
- Entity F - Stored Procedures
- Entity F - Disconnected Entities
- Entity F - Table-Valued Function
- Entity Framework - Native SQL
- Entity Framework - Enum Support
- Entity F - Asynchronous Query
- Entity Framework - Persistence
- Entity F - Projection Queries
- Entity F - Command Logging
- Entity F - Command Interception
- Entity Framework - Spatial Data Type
- Entity Framework - Inheritance
- Entity Framework - Migration
- Entity Framework - Eager Loading
- Entity Framework - Lazy Loading
- Entity Framework - Explicit Loading
- Entity Framework - Validation
- Entity Framework - Track Changes
- Entity Framework - Colored Entities
- Entity F - Code First Approach
- Entity Framework - First Example
- Entity Framework - Data Annotations
- Entity Framework - Fluent API
- Entity Framework - Seed Database
- Entity F - Code First Migration
- Entity F - Multiple DbContext
- Entity F - Nested Entity Types
- Entity Framework Resources
- Entity Framework - Quick Guide
- Entity Framework - Useful Resources
- Entity Framework - Discussion
Entity Framework - Views
A view is an object that contains data obtained by a predefined query. A view is a virtual object or table whose result set is derived from a query. It is very similar to a real table because it contains columns and rows of data. Following are some typical uses of views −
- Filter data of underlying tables
- Filter data for security purposes
- Centralize data distributed across several servers
- Create a reusable set of data
Views can be used in a similar way as you can use tables. To use view as an entity, first you will need to add database views to EDM. After adding views to your model then you can work with it the same way as normal entities except for Create, Update, and Delete operations.
Let’s take a look, how to add views into the model from the database.
Step 1 − Create a new Console Application project.
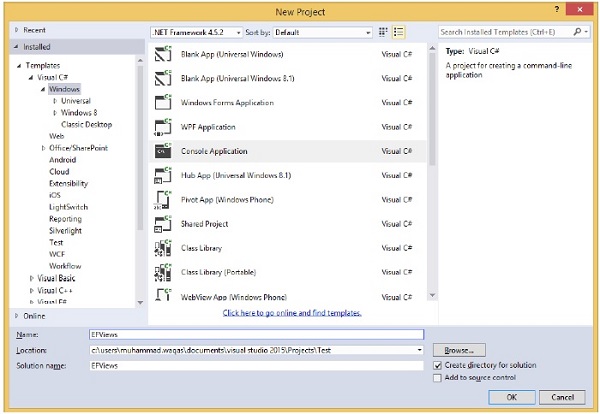
Step 2 − Right-click on project in solution explorer and select Add → New Item.
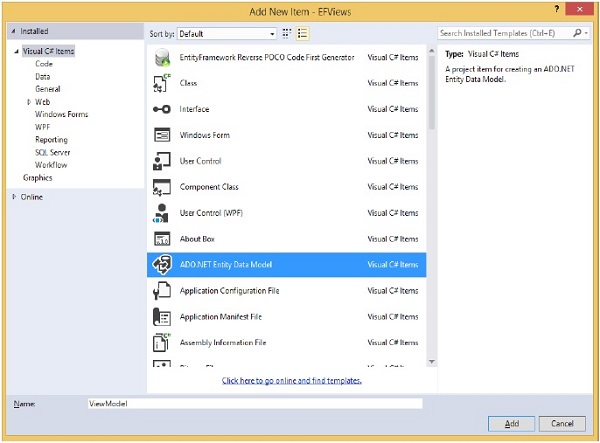
Step 3 − Select ADO.NET Entity Data Model from the middle pane and enter name ViewModel in the Name field.
Step 4 − Click Add button which will launch the Entity Data Model Wizard dialog.
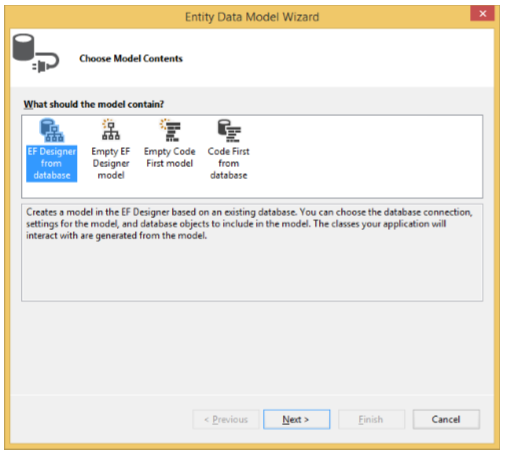
Step 5 − Select EF Designer from database and click Next button.
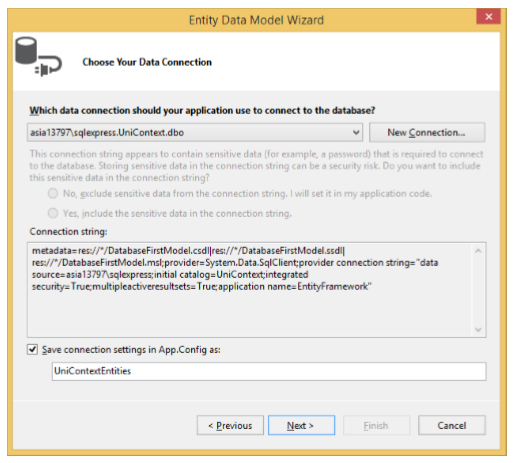
Step 6 − Select the existing database and click Next.
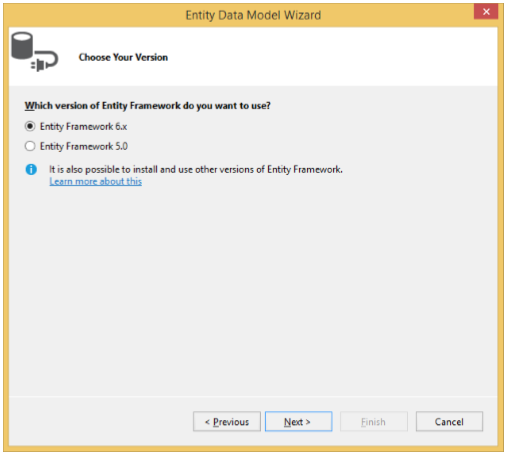
Step 7 − Choose Entity Framework 6.x and click Next.
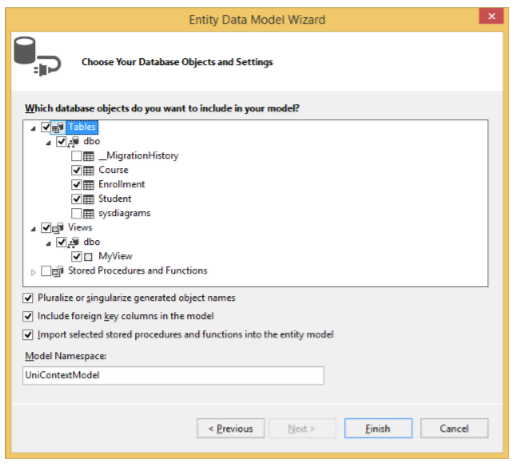
Step 8 − Select tables and views from your database and click Finish.
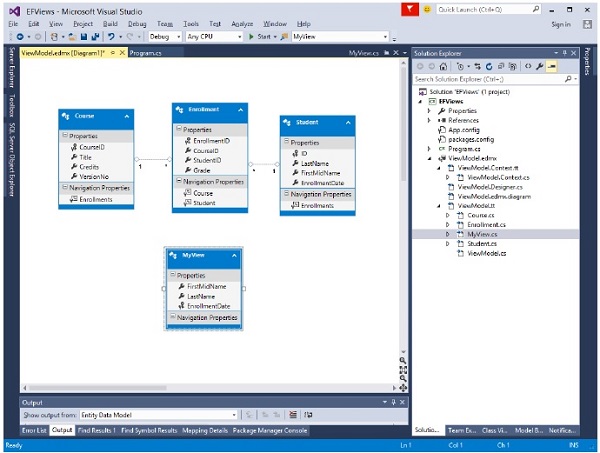
You can see in the designer window that a view is created and you can use it in the program as an entity.
In the solution explorer, you can see that MyView class is also generated from the database.
Let’s take an example in which all data is retrieved from view. Following is the code −
class Program { static void Main(string[] args) { using (var db = new UniContextEntities()) { var query = from b in db.MyViews orderby b.FirstMidName select b; Console.WriteLine("All student in the database:"); foreach (var item in query) { Console.WriteLine(item.FirstMidName + " " + item.LastName); } Console.WriteLine("Press any key to exit..."); Console.ReadKey(); } } }
When the above code is executed, you will receive the following output −
All student in the database: Ali Khan Arturo finand Bill Gates Carson Alexander Gytis Barzdukas Laura Norman Meredith Alonso Nino Olivetto Peggy Justice Yan Li Press any key to exit...
We recommend you to execute the above example in a step-by-step manner for better understanding.